Question
The following program is in C: Modify the following C program so that the card-dealing function deals a five-card poker hand. Then write the following
The following program is in C:
Modify the following C program so that the card-dealing function deals a five-card poker hand. Then write the following additional functions: a) Determine whether the hand contains a pair. b) Determine whether the hand contains two pairs. c) Determine whether the hand contains three of a kind (e.g., three jacks). d) Determine whether the hand contains four of a kind (e.g., four aces). e) Determine whether the hand contains a flush (i.e., all five cards of the same suit). f) Determine whether the hand contains a straight (i.e., five cards of consecutive face values).
#include
#include
#include
#define CARDS 52
#define FACES 13
// card structure definition
struct card {
const char *face; // define pointer face
const char *suit; // define pointer suit
};
typedef struct card Card; // new type name for struct card
// prototypes
void fillDeck(Card * const wDeck, const char * wFace[],
const char * wSuit[]);
void shuffle(Card * const wDeck);
void deal(const Card * const wDeck);
int main(void)
{
Card deck[CARDS]; // define array of Cards
// initialize array of pointers
const char *face[] = { "Ace", "Deuce", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
// initialize array of pointers
const char *suit[] = { "Hearts", "Diamonds", "Clubs", "Spades"};
srand(time(NULL)); // randomize
fillDeck(deck, face, suit); // load the deck with Cards
shuffle(deck); // put Cards in random order
deal(deck); // deal all 52 Cards
}
// place strings into Card structures
void fillDeck(Card * const wDeck, const char * wFace[],
const char * wSuit[])
{
// loop through wDeck
for (size_t i = 0; i < CARDS; ++i) {
wDeck[i].face = wFace[i % FACES];
wDeck[i].suit = wSuit[i / FACES];
}
}
// shuffle cards
void shuffle(Card * const wDeck)
{
// loop through wDeck randomly swapping Cards
for (size_t i = 0; i < CARDS; ++i) {
size_t j = rand() % CARDS;
Card temp = wDeck[i];
wDeck[i] = wDeck[j];
wDeck[j] = temp;
}
}
// deal cards
void deal(const Card * const wDeck)
{
// loop through wDeck
for (size_t i = 0; i < CARDS; ++i) {
printf("%5s of %-8s%s", wDeck[i].face, wDeck[i].suit,
(i + 1) % 4 ? " " : " ");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
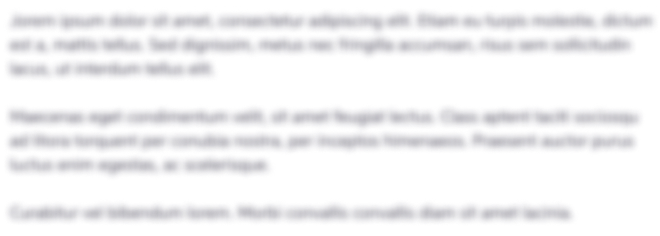
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started