Question
The following rules should be observed throughout the assignment: Each polynomial should be represented as a singly-linked list (see files list.h and list.c from the
The following rules should be observed throughout the assignment:
Each polynomial should be represented as a singly-linked list (see files list.h and list.c from the book).
Each element in the linked list should represent one of the terms in the polynomial.
The data held by each element should be type double representing the constant for that term.
For example, the polynomial 6.0x3 - 5.3x + 3.1 would be represented by the linked list 6.0 0.0 -5.3 3.1.
a) (1 point) Implement a function called appendTerm:
void appendTerm(List *pPolynomial, double constant);
This function should append (insert at the end) the value constant to pPolynomial. For example, appending 3.1 to pPolynomial already containing 6.0 0.0 -5.3 should result in the value 3.1 being added at the end: 6.0 0.0 -5.3 3.1. If the append fails the program should exit.
b) (2 points) Implement a function called display:
void display(List *pPolynomial);
This function should output the polynomial to stdout in proper polynomial format. For example, displaying polynomial 6.0 0.0 -5.3 3.1 should result in 6.0x^3 - 5.3x + 3.1 being output.
c) (2 points) Implement a function called evaluate:
double evaluate(List *pPolynomial, double x);
This function should evaluate the polynomial for the given value of x and return the result. For example, given polynomial 6.0 0.0 -5.3 3.1 and x having value 7.0 the function should return 2024.0 (the result of evaluating 6.0*7.03 - 5.3*7.0 + 3.1).
d) (4 points) Write a program to test the functions from parts a c. Your test program should demonstrate creating, displaying, and evaluating the following polynomials with the given values for x:
x + 1.0 with x = 1.0
x 2 - 1.0 with x = 2.03
-3.0x3 + 0.5x2 - 2.0x with x = 05.0
-0.3125x4 - 9.915x2 - 7.75x - 40.0 with x = 123.45
e. (1 point) Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
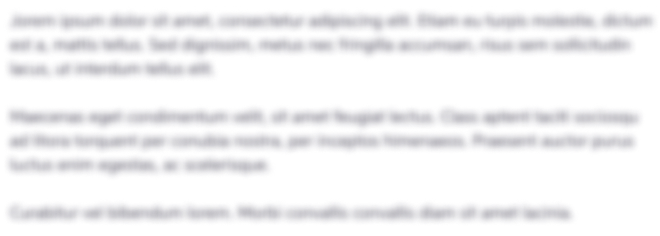
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started