Question
%{ The function phaseFinder takes two inputs, a temperature and composisiton of a two part mixture and returns its corresponding location on the provided phase
%{ The function "phaseFinder" takes two inputs, a temperature and composisiton of a two part mixture and returns its corresponding location on the provided phase diagram.
The input temperature is to be non-negative and measured in Celcius. The input composition of the mixture is to be the percentage of Component B, within the two part (A + B) mixture. (e.g For a mixture of 25% material A and 75% material B, CompB = 75)
******DO NOT EDIT THE "phaseFinder" FUNCTION******
Your task is to complete two of the functions used within "phaseFinder". Those Functions are: "checkBetaLiquid(T,B)" "checkBeta(T,B)" %}
function result = phaseFinder(Temp, CompB) %checks the inputs are valid validInputs = testInputConditions(Temp, CompB); if validInputs == 1 %Determine which regions the inputs correspond to. a = checkAlpha(Temp, CompB); b = checkBeta(Temp, CompB); l = checkLiquid(Temp, CompB); a_b = checkAlphaBeta(Temp,CompB); a_l = checkAlphaLiquid(Temp,CompB); b_l = checkBetaLiquid(Temp,CompB); %Creates an array indicating correspondance of inputs to regions testArray = [a b l a_b a_l b_l]; %Check if the mixture sits at the Eutectic Point if isequal(testArray, [0 0 1 1 1 1]) fprintf('Mixture sits on the Eutectic Point '); result = 1; %Check if the mixture sits at the juntion of any three regions elseif isequal(testArray, [1 0 0 1 1 0]) fprintf(['Mixture sits on the alpha/alpha_liquid/alpha_beta ' ... 'junction on the Eutectic Line ']); result = 2; elseif isequal(testArray, [0 1 0 1 0 1]) fprintf(['Mixture sits on the beta/beta_liquid/alpha_beta ' ... 'junction on the Eutectic Line ']); result = 3; elseif isequal(testArray, [1 0 1 0 1 0]) fprintf(['Mixture sits on the alpha/alpha_liquid/liquid' ... 'junction ']); result = 4; elseif isequal(testArray, [0 1 1 0 0 1]) fprintf(['Mixture sits on the beta/beta_liquid/liquid' ... 'junction ']); result = 5; %Check if the mixture sits at the border of any two regions elseif isequal(testArray, [1 0 0 1 0 0]) fprintf('Mixture sits on the alpha/alpha_beta border '); result = 6; elseif isequal(testArray, [1 0 0 0 1 0]) fprintf('Mixture sits on the alpha/alpha_liquid border '); result = 7; elseif isequal(testArray, [0 0 0 1 1 0]) fprintf(['Mixture sits on the alpha_liquid/alpha_beta ' ... 'border on the Eutectic Line ']); result = 8; elseif isequal(testArray, [0 1 0 1 0 0]) fprintf('Mixture sits on the beta/alpha_beta border '); result = 9; elseif isequal(testArray, [0 0 0 1 0 1]) fprintf(['Mixture sits on the beta_liquid/alpha_beta ' ... 'border on the Eutectic Line ']); result = 10; elseif isequal(testArray, [0 1 0 0 0 1]) fprintf('Mixture sits on the beta/beta_liquid border '); result = 11; elseif isequal(testArray, [0 0 1 0 1 0]) fprintf('Mixture sits on the liquid/alpha_liquid border '); result = 12; elseif isequal(testArray, [0 0 1 0 0 1]) fprintf('Mixture sits on the liquid/beta_liquid border '); result = 13; %check if the mixture sits at within only one of the regions elseif isequal(testArray, [1 0 0 0 0 0]) fprintf('Mixture belongs to the alpha region '); result = 14; elseif isequal(testArray, [0 1 0 0 0 0]) fprintf('Mixture belongs to the beta region '); result = 15; elseif isequal(testArray, [0 0 1 0 0 0]) fprintf('Mixture belongs to the liquid region '); result = 16; elseif isequal(testArray, [0 0 0 1 0 0]) fprintf('Mixture belongs to the alpha_beta region '); result = 17; elseif isequal(testArray, [0 0 0 0 1 0]) fprintf('Mixture belongs to the alpha_liquid region '); result = 18; elseif isequal(testArray, [0 0 0 0 0 1]) fprintf('Mixture belongs to the beta_liquid region '); result = 19; %Declares that the mixture is not within any of the defined regions else fprintf(['Mixture belongs to an immpossible combination of' ... ' regions ... that can''t be right ']); result = 20; end else %Inputs were not valid result = -1; end end
%The Following function checks that; %Input Temperature is non-negative %Input Composition is between 0 and 100 %DO NOT EDIT THIS FUNCTION function test = testInputConditions(T,B) if (T >= 0) && (B >= 0) && (B <= 100) test = 1; else test = 0; if (T < 0) fprintf('Input Error: Temp(Celcius) must be +ve integer '); end if (B < 0) || (B >100) fprintf('Input Error: Comp(%%A) must be between 0 and 100 '); end end end
%The Following function checks if input conditions indicate that the %mixture is in the 'alpha' region. %DO NOT EDIT THIS FUNCTION
function a = checkAlpha(T,B) maxTemp = -(400/15)*B + 700; minTemp = 20*B; if (T <= maxTemp) && (T >= minTemp) a = 1; else a = 0; end end
%The Following function checks if input conditions indicate that the %mixture is in the 'alpha + beta' region. %DO NOT EDIT THIS FUNCTION
function a_b = checkAlphaBeta(T,B) minComp = 0.05*T; maxComp = 100 - 0.05*T; if (T <= 300) && (B >= minComp) && (B <= maxComp) a_b = 1; else a_b = 0; end end
%The Following function checks if input conditions indicate that the %mixture is in the 'alpha + liquid' region. %Sets output to 1 if the mixtue is WITHIN the region, otherwise sets to 0. %DO NOT EDIT THIS FUNCTION function a_liquid = checkAlphaLiquid(T,B) minComp = 26.25 - 0.0375*T; maxComp = 87.5 - (T/8); if (T>= 300) && (T <= 700) && (B >= minComp) && (B <= maxComp) a_liquid = 1; else a_liquid = 0; end end
%The Following function checks if input conditions indicate that the %mixture is in the 'beta + liquid' region. %Sets output to 1 if the mixtue is WITHIN the region, otherwise sets to 0.
function b_liquid = checkBetaLiquid(T,B) b_liquid = 1;%REMOVE THIS LINE AND COMPLETE THE FUNCTION end
%The Following function checks if input conditions indicate that the %mixture is in the 'liquid' region. %Sets output to 1 if the mixtue is WITHIN the region, otherwise sets to 0. %DO NOT EDIT THIS FUNCTION
function liquid = checkLiquid(T,B) minAlphaTemp = 700 - 8*B; minBetaTemp = 10*B - 200; if (T >= minAlphaTemp) && (T >= minBetaTemp) liquid = 1; else liquid = 0; end end
%The Following function checks if input conditions indicate that the %mixture is in the 'beta' region. %Sets output to 1 if the mixtue is WITHIN the region, otherwise sets to 0.
function b = checkBeta(T,B) b = 0;%REMOVE THIS LINE AND COMPLETE THE FUNCTION end
Step by Step Solution
There are 3 Steps involved in it
Step: 1
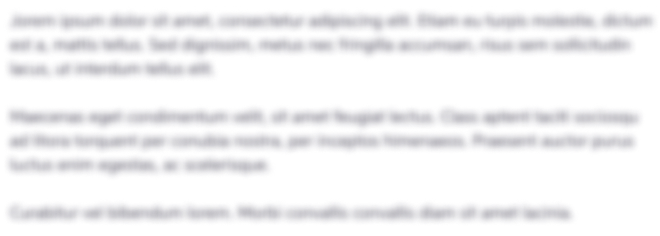
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started