Question
The goal is to practice: How to reuse previously implemented classes Observe how to use Graphics to Draw on JPanel To extract java files from
The goal is to practice:
How to reuse previously implemented classes
Observe how to use Graphics to Draw on JPanel
To extract java files from a jar file
Observe how to use mouse events
You are going to implement a GUI to draw certain objects on the Drawing Panel. I provided you the toolbar, panel creation piece of code, hence all you need to do is implement the paint functions of the classes Circle, Cube and Square. Also, please explore in detail the project. Observe how OO paradigm is applied and how it makes the life easy in terms of modularity, reusability and maintenance.
In the implementation, I reused my solution to the 3rd Assignment, and modified it tiny-bit. I added position information on the panel, abstract paint function and also updated the mSide and mRadius types to int from float according to needs of the current assignment.
A small note: I used a black boundary color for the square and the circle, but it is completely optional.
Run Scenario:
Select an object on the toolbar
Click a point on the blue drawing panel
Selected object is drawn on the drawing panel:
Circle center at the clicked point
Square top-left corner at the clicked point
Cube front-top-left corner at the clicked point
Sample output : Assignment4_Screenshot.png
You are going to build upon this project : Assignment4_ShareWithStudents.jar
// Assignment4.java
package ShareWithStudents;
import java.awt.Color; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.ItemEvent; import java.awt.event.ItemListener; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.util.ArrayList; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JToggleButton; import javax.swing.JToolBar; import javax.swing.border.EtchedBorder;
public class Assignment4
extends JFrame implements MouseListener { final int NUMBER_OF_SHAPES = 3; JToolBar mToolbar; ShapeSelected mSelectedAction; JToggleButton mCircleButton; JToggleButton mCubeButton; JToggleButton mSquareButton; DrawPanel mDrawPanel; static ArrayList mShapes = new ArrayList(); public static void main(String[] args) { new Assignment4(); } private JToggleButton addButton(String title, final ShapeSelected shapeSelected) { JToggleButton button = new JToggleButton(title); button.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent ev) { if (ev.getStateChange() == 1) {
switch (Assignment4.3.$SwitchMap$ShareWithStudents$ShapeSelected[mSelectedAction.ordinal()]) { case 1: mCircleButton.setSelected(false); break; case 2: mCubeButton.setSelected(false); break; case 3: mSquareButton.setSelected(false); }
mSelectedAction = shapeSelected; } else if (ev.getStateChange() == 2) { mSelectedAction = ShapeSelected.None; } } }); mToolbar.add(button); return button; }
public Assignment4() { int WIDTH = 1000; int HEIGHT = 800; mSelectedAction = ShapeSelected.None; JFrame guiFrame = new JFrame();
guiFrame.setDefaultCloseOperation(3); guiFrame.setTitle("SHAPE GUI"); guiFrame.setSize(1000, 800);
guiFrame.setLocationRelativeTo(null);
mToolbar = new JToolBar(); mToolbar.setBorder(new EtchedBorder()); mDrawPanel = new DrawPanel(); mDrawPanel.setBackground(Color.blue); mDrawPanel.paintDisplay(mShapes); guiFrame.add(mDrawPanel); guiFrame.add(mToolbar, "North"); mCircleButton = addButton("Circle", ShapeSelected.Circle); mCubeButton = addButton("Cube", ShapeSelected.Cube); mSquareButton = addButton("Square", ShapeSelected.Square);
JButton button = new JButton("Quit"); button.setToolTipText("Quit the program"); button.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { System.exit(0); } }); mToolbar.add(button); guiFrame.addMouseListener(this);
guiFrame.setVisible(true); }
public void mouseClicked(MouseEvent e) { int x = e.getX(); int y = e.getY(); switch (3.$SwitchMap$ShareWithStudents$ShapeSelected[mSelectedAction.ordinal()]) { case 1: Circle circle = new Circle(Color.yellow, "crl", 50); circle.setPos(x, y - 50); mShapes.add(circle); break; case 2: Cube cube = new Cube(Color.red, "cb", 100); cube.setPos(x, y - 50); mShapes.add(cube); break; case 3: Square square = new Square(Color.green, "sq", 100); square.setPos(x, y - 50); mShapes.add(square); } mDrawPanel.paintDisplay(mShapes); } public void mousePressed(MouseEvent e) {} public void mouseReleased(MouseEvent e) {} public void mouseEntered(MouseEvent e) {} public void mouseExited(MouseEvent e) {} }
// Circle.java
package ShareWithStudents;
import java.awt.Color; import java.awt.Graphics; import java.io.PrintStream;
public class Circle extends Shape implements I_twoD { private int mRadius; public Circle(Color color, String name, int radius) { super(color, name); mRadius = radius; mX = -1; mY = -1; } public float computeArea() { return (float)(3.141592653589793D * mRadius * mRadius); } public float computePerimeter() { return (float)(6.283185307179586D * mRadius); } public void print() { System.out.println("Circle (" + mName + ") radius : " + mRadius + " and color : " + mColor.toString()); } public void paint(Graphics g) {} }
// Cube.java
package ShareWithStudents;
import java.awt.Color; import java.awt.Graphics; import java.io.PrintStream;
public class Cube extends Square implements I_threeD { public Cube(Color color, String name, int side) { super(color, name, side); }
public float computeVolume() { return mSide * mSide * mSide; } public void print() { System.out.println("Cube (" + mName + ") side : " + mSide + " and color : " + mColor.toString()); } public void paint(Graphics g) { int step = 20; } }
// DrawPanel.java
package ShareWithStudents;
import java.awt.Graphics; import java.util.ArrayList; import javax.swing.JPanel;
class DrawPanel extends JPanel { ArrayList mShapes; DrawPanel() {} public void paintComponent(Graphics g) { super.paintComponent(g); for (Shape s : mShapes) { s.paint(g); } } public void paintDisplay(ArrayList shapes) { mShapes = shapes; repaint(); } }
// I_threeD.java
package ShareWithStudents;
public abstract interface I_threeD { public abstract float computeVolume(); }
// I_twoD.java
package ShareWithStudents;
public abstract interface I_twoD { public abstract float computeArea(); public abstract float computePerimeter(); }
// Shape.java
package ShareWithStudents;
import java.awt.Color; import java.awt.Graphics;
public abstract class Shape { protected Color mColor; String mName; protected int mX; protected int mY; public Shape(Color color, String name) { mColor = color; mName = name; } public String getName() { return mName; } public Color getColor() { return mColor; } public void setName(String name) { mName = name; }
public void setColor(Color color) { mColor = color; } public abstract void print(); public abstract void paint(Graphics paramGraphics); public void setPos(int x, int y) { mX = x; mY = y; } }
// ShapeSelected.java
package ShareWithStudents;
enum ShapeSelected { None, Circle, Square, Cube; private ShapeSelected() {} }
// Square.java
package ShareWithStudents;
import java.awt.Color; import java.awt.Graphics; import java.io.PrintStream;
public class Square extends Shape implements I_twoD { protected int mSide; Square(Color color, String name, int side) { super(color, name); mSide = side; }
public float computeArea() { return mSide * mSide; }
public float computePerimeter() { return 4.0F * mSide; } public void print() { System.out.println("Square (" + mName + ") side : " + mSide + " and color : " + mColor.toString()); } public void paint(Graphics g) {} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
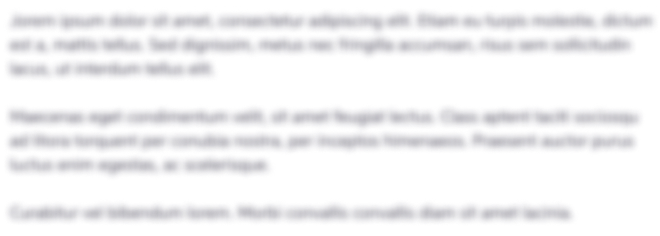
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started