Question
The goal of this assignment is to reinforce the Heap data structure in C++. Finish the implementation of priority_queue_heap. The only member data will be
The goal of this assignment is to reinforce the Heap data structure in C++.
Finish the implementation of priority_queue_heap. The only member data will be a heap. Modify the main.cpp to create two new functions to demonstrate two implementations of priority queues.
- priority_queue_simple
- priority_queue_heap
//Priority_Queue_Heap Header File
#ifndef PRIORITY_QUEUE_HEAP_H #define PRIORITY_QUEUE_HEAP_H
template
bool is_empty() const;
bool is_full() const;
/** * Remove the largest value from this priority queue and return it. * * Precondition: priority queue is not empty. */ T remove();
/** * Inserts the 'value' into the priority queue. * * Precondition: priority queue is not full */ void insert(const T& value);
};
#include "priority_queue_heap.template"
#endif // PRIORITY_QUEUE_HEAP_H
#ifndef PRIORITY_QUEUE_SIMPLE_H #define PRIORITY_QUEUE_SIMPLE_H
/** * This class implements a priority queue using a very simple strategy: * Store the values in an array. * Add new values at the end. * When asked to remove a value, search for the largest (linear search) * */
template
priority_queue_simple() { size = 0; }
bool is_empty() const { return size == 0; }
bool is_full() const { return size == CAPACITY; }
/** * Remove the largest value from this priority queue and return it. * * Precondition: priority queue is not empty. */ T remove();
/** * Inserts the 'value' into the priority queue. * * Precondition: priority queue is not full */ void insert(const T& value);
private: T data[CAPACITY]; int size; };
#include "priority_queue_simple.template"
#endif // PRIORITY_QUEUE_SIMPLE_H
//Template File
#include
/** * Remove the largest value from this priority queue and return it. * * Precondition: priority queue is not empty. */ template
/** * Inserts the 'value' into the priority queue. * * Precondition: priority queue is not full */ template
//Main File
#include "heap.h" #include
int test1() { heap
int x = hp.remove(); cout << "removed " << x << endl; x = hp.remove(); cout << "removed " << x << endl; x = hp.remove(); cout << "removed " << x << endl; x = hp.remove(); cout << "removed " << x << endl; x = hp.remove(); cout << "removed " << x << endl;
cout << "empty? " << hp.is_empty() << endl; }
void test2() { srand(time(NULL)); heap
}
int main() { test1(); test2():
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
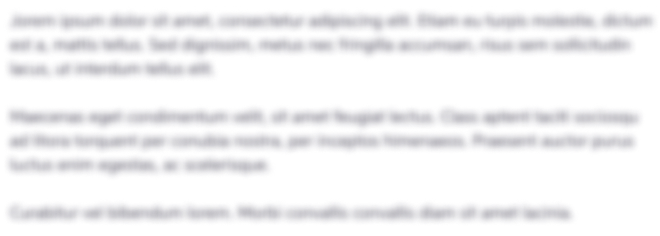
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started