Question
The goal of this project is to create a model that adds People to a database (list) in python ! *fix all these files *
The goal of this project is to create a model that adds People to a database (list) in python !
*fix all these files *
To start with, you have to carefully distinguish between the person.py file and the persons.py file. The first file is where all the persons basic information are handled, where the second file is there to hold a set of these person's objects.
You should start by implementing the person.py file. Many methods are either implemented for you or you are given a skeleton. Any comment that says "Fix Me" means the method should be reimplemented.
To completely fix the Person class, you have to fix the method in the utility.py file. There is a test file name test_utility.py given just for this task. Also, you are given full instructions on the utility file itself.
Next, you should move to the persons.py file. There two methods that needs fixing. The first is given (remove). Follow the instructions given to implement it correctly. The other methods (append) is not given at all. However, there is a comment that describes what it should do.
Now you have the Model files fixed. Next, you should move to the controller file. You are given an example on how to add a student information. You need to do the same for the faculty case.
The CLI class is almost completely given for you. All you need to add is the key for the fourth case "remove person". Use all the information given to you in the class to understand how the system as a whole works.
person.py :
import utility
from datetime import datetime
class Person:
def __init__(self, firstname: str, lastname: str, date_of_birth: str): """ Initialize a persons object. Although the date_of_birth is of type str, the initializer here should take care of converting it to datetime object.
Hint: Fix what is in the util file!
:param firstname: The person's first name. :param lastname: The person's last name. :param date_of_birth: The person's date of birth as a string. """ self._first_name = firstname self._last_name = lastname # TODO: Fix Me! The _dob should take a datetime object based on the passed string. self._dob = None
def get_name(self) -> str: """ Format the name such that the last name appears before the first name. Example, my name should be written as 'Alsaeed, Ziyad'. :return: A string of the full name where the last name appears first, and they are seperated by a comma. """ # TODO: Fix Me! pass
def get_age(self) -> int: """ Calculate the age of the person given today's date and their date of birth. Hint: there are 365.2425 days in a year! :return: The age of the person in years as an integer. """ # TODO: Fix Me! pass
def __str__(self): """ Format the object to print the full name with the age all together. :return: """ return f"{self.get_name()}: {self.get_age()}"
class Faculty(Person):
def __init__(self, firstname: str, lastname: str, date_of_birth: str, office: int): super().__init__(firstname=firstname, lastname=lastname, date_of_birth=date_of_birth) self._office = office
def get_office_number(self) -> int: """ Get the faculty office number! """ # TODO: Fix Me! pass
def __str__(self): return f"Faculty: {super().__str__()} | Office: {self.get_office_number()}"
class Student(Person):
def __init__(self, firstname: str, lastname: str, date_of_birth: str, sid: int): super().__init__(firstname=firstname, lastname=lastname, date_of_birth=date_of_birth) self._sid = sid
def get_student_id(self) -> int: """ Get the student's id number! """ # TODO: Fix Me! pass
def __str__(self): return f"Student: {super().__str__()} | SID: {self.get_student_id()}"
persons.py :
""" A person list module where we keep track of all the persons added to our database.
The class here should wrap the list class instead of inheriting it.
"""
from typing import List from person import Person
class PersonList: """ A person list class. It wraps the built-in list class to keep track of all the person's information added """
def __init__(self): """ Start by initializing an empty list. Preferably indicate the type of the list and what it takes. """ self.people: List[Person] = []
def get_people(self) -> list: """ Return all the person objects in the listed sorted based on their get_name(). :return: A sorted list of the people variable. """ return sorted(self.people, key=lambda person: person.get_name())
def remove(self, last_name: str): """ Given some person last_name, remove any matches to it. :param last_name: A person(s) last name to be removed if found in the list of people. """ # TODO: Fix Me! this method takes a string as a last_name and remove it from the list if it exist. pass
# TODO: Add an "append" method that takes a Person and add it to the list. No Skeleton given!
controller.py :
""" This is the Controller Module. Although we might not implement it to be perfect, the hope is that most of the methods here will not change with any UI changes.
"""
from cli import CLI from persons import PersonList from person import Student, Faculty
class Controller:
def __init__(self): self._persons = PersonList() self._view = CLI()
def run(self, args: list): """
:param args: :return: """ while True: inp = self._view.show_menu() if inp == "1": # add student self.add_student(self._view.add_student()) elif inp == "2": # add teacher self.add_faculty(self._view.add_faculty()) elif inp == "3": # list people self._view.list_people(self._persons.get_people()) # TODO: Fix Me! Where is case #4? (it is used to remove a person by lastname) elif inp == "5": # exit break else: self._view.show_error("Invalid Input!")
def add_student(self, inp: str): """ Add a student information using the CLI passed inputs. :param inp: the student information as given in the command line. """ data = inp.split(" ") try: p = Student(data[0], data[1], data[2], int(data[3])) self._persons.append(p) except SyntaxError as e: # notify the view of the error! self._view.show_error(f"Unable to parse input! {e}")
def add_faculty(self, inp: str): """ Add a student information using the CLI passed inputs. :param inp: the student information as given in the command line. """ # TODO: Fix Me! same as seen in the add_student function. pass
def remove_person(self, inp: str): """ Remove a person from the list of persons using their last name This should call the remove function on the PersonList class. """ # TODO: Fix Me! pass
utility.py:
""" All the utility functions needed to easily complete this project!
"""
from datetime import datetime
def convert_string_to_datetime(date: str) -> datetime: """ Takes a date in a string format (e.g., `2022-10-05`) and returns a datetime object using the format. If the string given is wrong, it throws and error.
Hint: Search for "python split a string by delimiter"!
:param date: a string representing the date. :return: A datetime object reflecting the date given as a string. """ # TODO: Fix Me! pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
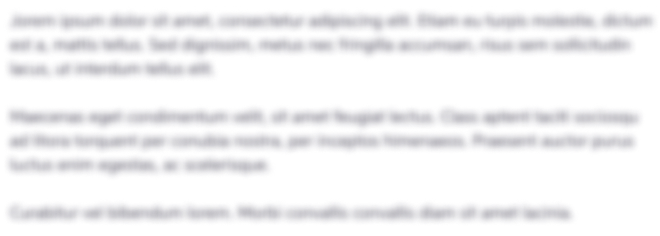
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started