Question
The grocery store simulation is based on a priority queue of grocery lines. The grocery lines are queues, and the priority queue is needed to
The grocery store simulation is based on a priority queue of grocery lines. The grocery lines are queues, and the priority queue is needed to pick the best line for the next person to join. Below is a "plan of attack" for this assignment:
The following classes are provided:
- GroceryStore.java - Represents an entire grocery store and runs a text-based simulation.
- SimGUI.java - A graphical representation of the grocery store with simulation controls. There is a large amount of data structure code we will be completing to support the simulation shown. Do not start writing code yet! Start by getting a good sense of what youll be doing:
- Person.java - This class represents a single person with their cart. It has the comments describing each of the methods we need to complete (see Step 1).
- SimpleList.java - This generic class extends Java's AbstractList class (see Step 2). This is a simple linked list similar to the one we built in class, but it is doubly linked.
- SimpleQueue.java - This generic class extends SimpleList and implements Java's Queue interface (see Step 3). You may want to review how inheritance works in Java using either your CS211 materials or the resources listed in the appendix of this document.
- GroceryLine.java - This class is a type of SimpleQueue specifically designed to order a group of people (it extends SimpleQueue
) and it requires some additional features (see Step 4). You may want to review the Comparable interface Java using either your CS211 materials or the resources listed in the appendix of this document. - PriorityQueue.java - This class extends SimpleQueue to turn it into a priority queue. In class we have discussed a number of ways to implement a priority queue with a list. This class has Big-O restrictions you must meet which will determine how you implement it.
Implement an update()method which accepts an item and "updates" its place in the queue (i.e. update its priority). Lower priority items should be at the front of the queue (since well want to pick the shortestlines, not the longestlines in our grocery store).
import java.util.NoSuchElementException; import java.util.Date; //for testing
//priority queue where the minimum item has the highest priority class PriorityQueue> extends SimpleQueue { //updates an item that's already in the queue //NOTE: This should update the exact item in memory, //not just any "equal" item (in other words, you //should use == here and not .equals())
public void update(T item) { //O(n) //throws NoSuchElementException if item is not //in the queue } //You may need to override some other methods from SimpleQueue! //Restriction 1: all methods from SimpleQueue should still work //(as in, if you add(), the value should be added, if you call //size() it should return the correct value, etc.). However, //remove/poll will remove the _minimum_ value from the queue; //element/peek will return the _minimum_ value from the queue. //Restriction 2: element() and peek() must still both be O(1) //------------------------------------------------------------- // Main Method For Your Testing -- Edit all you want //------------------------------------------------------------- public static void main(String[] args){ PriorityQueue values = new PriorityQueue<>(); Date[] dates = new Date[5]; for (int i=5; i>=1; i--){ dates[i-1] = new Date(86400000*i); values.add(dates[i-1]); } for(Date d : values) { System.out.println(d); }
dates[3].setTime(0); values.update(dates[3]); System.out.println(); for(Date d : values) { System.out.println(d); } if(values.peek().equals(dates[3])) { System.out.println(" Yay 1"); } } }
https://www.chegg.com/homework-help/questions-and-answers/java-personjava-class-represents-single-person-cart-comments-describing-methods-need-compl-q34817547?trackid=2c2ae8c4b1b4&strackid=0bb7bfa62744&ii=1
https://www.chegg.com/homework-help/questions-and-answers/java-reference-https-docsoraclecom-javase-8-docs-api-java-util-abstractlisthtml-going-impl-q34836993?trackid=ece036e8ad1b&strackid=8b5cc9624370&ii=1
https://www.chegg.com/homework-help/questions-and-answers/import-javautilqueue-import-javautilnosuchelementexception-class-simple-queue-based-simple-q34996504?trackid=AkMAJs02
https://www.chegg.com/homework-help/questions-and-answers/import-javautilqueue-import-javautilnosuchelementexception-class-simple-queue-based-simple-q34996504?trackid=DH3N_ydQ
Step by Step Solution
There are 3 Steps involved in it
Step: 1
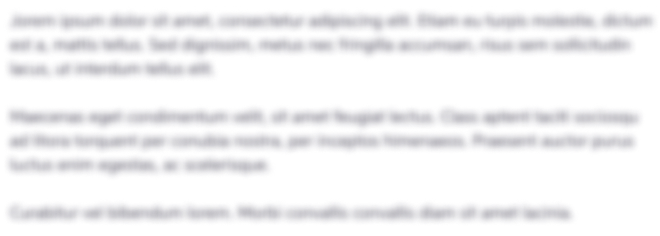
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started