Question
The implementations of the methods get (int index), indexOf (E e), lastIndexOf(E e), set(int index, E e), and the remove() method of the private class
The implementations of the methods get (int index), indexOf (E e), lastIndexOf(E e), set(int index, E e), and the remove() method of the private class LinkedListIterator are omitted in the MyLinkedList class. Implement these methods.
Changes are needed in MyLinkedList.java
Test.java (No changes needed in this)
public class Test {
public static void main(String[] args){
//TestArrayList
//Auto-generated method stub
//-------------------------------------------Test addAll() --------------------------------------------
System.out.println("Test case for addAll() Method");
MyArrayList
al1.add(1);
al1.add(2);
al1.add(3);
al1.add(4);
al1.add(5);
System.out.println("Print all the element of l1");
for (Integer number : al1)
{
System.out.println("Number = " + number );
}
MyArrayList
al2.add(6);
al2.add(7);
al2.add(8);
al2.add(9);
al2.add(10);
System.out.println("Print all the element of l2");
for (Integer number : al2)
{
System.out.println("Number = " + number );
}
System.out.println("");
al1.addAll(al2);
System.out.println("After calling addAll method, the contain of l1 is list below.");
for (Integer number : al1)
{
System.out.println("Number = " + number );
}
//-------------------------------------------Test removeAll() --------------------------------------------
System.out.println("Test case for removeAll() Method");
al1.removeAll(al1);
System.out.println("After calling removeAll method, the contain of l1 should be empty.");
for (Integer number : al1)
{
System.out.println("Number = " + number );
}
//-------------------------------------------Test retainAll() --------------------------------------------
System.out.println("Test case for retainAll() Method");
MyArrayList
al3.add(5);
al3.add(6);
al3.add(14);
al3.add(10);
System.out.println("Print all the element of l3");
for (Integer number : al3)
{
System.out.println("Number = " + number );
}
MyArrayList
al4.add(5);
al4.add(6);
al4.add(9);
System.out.println("Print all the element of l4");
for (Integer number : al4)
{
System.out.println("Number = " + number );
}
al4.retainAll(al3);
System.out.println("After applying the method, l3 is :"+ al3);
System.out.println("After applying the method, l4 is :"+ al4);
//-------------------------------------------Test toArray() --------------------------------------------
// Create an empty array list with initial value
System.out.println("Test case for toArray() Method");
MyArrayList
al5.add(5);
al5.add(8);
al5.add(10);
al5.add(12);
al5.add(15);
// print out before toArray method
for (Integer number : al5)
{
System.out.println("Number = " + number );
}
// Call toArray() method.
Object[] ob = al5.toArray();
// Print out the result after calling toArray() Method.
System.out.println("Printing elements of list1 from 1st to last");
for (Object value : ob)
{
System.out.println("Number = " + value );
}
//-------------------------------------------Test toArray(T[]) --------------------------------------------
System.out.println("Test case for toArray(T[]) Method");
// Create an empty array list with initial value
MyArrayList
al6.add(1);
al6.add(2);
al6.add(3);
al6.add(4);
al6.add(5);
for (Integer number : al6)
{
System.out.println("Number = " + number );
}
Integer list2[] = new Integer[al6.size()];
// Call toArray() method.
list2 = al6.toArray(list2);
// Print out the result after calling toArray(T[]) Method.
System.out.println("Printing elements of list2 from 1st to last");
for (Integer number : list2)
{
System.out.println("Number = " + number );
}
// Test LinkedList
// TODO Auto-generated method stub
//-------------------------------------------Test get(index) Method --------------------------------------------
System.out.println("Test case for get(index) Method");
MyLinkedList ll1 = new MyLinkedList();
ll1.add("What");
ll1.add("to");
ll1.add("eat");
ll1.add("tonight");
ll1.add("?");
// Print out the list first
System.out.println("Linked list " + ll1);
// Print the element of l1 at index 2
System.out.println("Element at index 0 is " + ll1.get(0));
System.out.println("Element at index 1 is " + ll1.get(1));
System.out.println("Element at index 2 is " + ll1.get(2));
System.out.println("Element at index 3 is " + ll1.get(3));
System.out.println("Element at index 4 is " + ll1.get(4));
//-------------------------------------------Test indexOf(Object e) Method --------------------------------------------
System.out.println("Test case for indexOf(Object e) Method");
System.out.println("index of element 'What' is " + ll1.indexOf("What"));
System.out.println("index of element 'to' is " + ll1.indexOf("to"));
System.out.println("index of element 'eat' is " + ll1.indexOf("eat"));
System.out.println("index of element 'tonight' is " + ll1.indexOf("tonight"));
System.out.println("index of element '?' is " + ll1.indexOf("?"));
//-------------------------------------------Test lastIndexOf(E e) Method --------------------------------------------
System.out.println("Test case for lastIndexOf(E e) Method");
MyLinkedList ll2 = new MyLinkedList();
Integer m = new Integer (343);
Integer n = new Integer(565);
ll2.add(m);
ll2.add(n);
ll2.add(m);
ll2.add(n);
System.out.println("The index of n is " + ll2.indexOf(n));
System.out.println("The last index of n is " + ll2.lastIndexOf(n));
//-------------------------------------------Test set(int index, E e) Method --------------------------------------------
System.out.println("Test case for set(int index, E e) Method");
System.out.println("Before calling set(1, m), l2 is " + ll2);
ll2.set(1, m);
System.out.println("After calling the method, l2 is "+ ll2);
//-------------------------------------------Test remove() Method --------------------------------------------
System.out.println("Test case for remove() Method");
MyLinkedList ll3 = new MyLinkedList();
ll3.add("33");
ll3.add("44");
ll3.add("55");
ll3.add("66");
System.out.println("Before calling remove() l3 is " + ll3);
//LinkedListIterator ii = new LinkedListIterator();
ll3.iterator().remove();// 66 should be gone
System.out.println("After calling the method, l3 is "+ ll3);
ll3.iterator().remove(); // 55 should be gone
System.out.println("After calling the method, l3 is "+ ll3);
ll3.iterator().remove(); // 44 should be gone
System.out.println("After calling the method, l3 is "+ ll3);
}
}
MyLinkedList.java (Needs changes)
public class MyLinkedList
private Node
private int size = 0; // Number of elements in the list
/** Create an empty list */
public MyLinkedList() {
}
/** Create a list from an array of objects */
public MyLinkedList(E[] objects) {
for (int i = 0; i < objects.length; i++)
add(objects[i]);
}
/** Return the head element in the list */
public E getFirst() {
if (size == 0) {
return null;
}
else {
return head.element;
}
}
/** Return the last element in the list */
public E getLast() {
if (size == 0) {
return null;
}
else {
return tail.element;
}
}
/** Add an element to the beginning of the list */
public void addFirst(E e) {
Node
newNode.next = head; // link the new node with the head
head = newNode; // head points to the new node
size++; // Increase list size
if (tail == null) // the new node is the only node in list
tail = head;
}
/** Add an element to the end of the list */
public void addLast(E e) {
Node
if (tail == null) {
head = tail = newNode; // The new node is the only node in list
}
else {
tail.next = newNode; // Link the new with the last node
tail = tail.next; // tail now points to the last node
}
size++; // Increase size
}
@Override /** Add a new element at the specified index
* in this list. The index of the head element is 0 */
public void add(int index, E e) {
if (index == 0) {
addFirst(e);
}
else if (index >= size) {
addLast(e);
}
else {
Node
for (int i = 1; i < index; i++) {
current = current.next;
}
Node
current.next = new Node<>(e);
(current.next).next = temp;
size++;
}
}
/** Remove the head node and
* return the object that is contained in the removed node. */
public E removeFirst() {
if (size == 0) {
return null;
}
else {
Node
head = head.next;
size--;
if (head == null) {
tail = null;
}
return temp.element;
}
}
/** Remove the last node and
* return the object that is contained in the removed node. */
public E removeLast() {
if (size == 0) {
return null;
}
else if (size == 1) {
Node
head = tail = null;
size = 0;
return temp.element;
}
else {
Node
for (int i = 0; i < size - 2; i++) {
current = current.next;
}
Node
tail = current;
tail.next = null;
size--;
return temp.element;
}
}
@Override /** Remove the element at the specified position in this
* list. Return the element that was removed from the list. */
public E remove(int index) {
if (index < 0 || index >= size) {
return null;
}
else if (index == 0) {
return removeFirst();
}
else if (index == size - 1) {
return removeLast();
}
else {
Node
for (int i = 1; i < index; i++) {
previous = previous.next;
}
Node
previous.next = current.next;
size--;
return current.element;
}
}
@Override /** Override toString() to return elements in the list */
public String toString() {
StringBuilder result = new StringBuilder("[");
Node
for (int i = 0; i < size; i++) {
result.append(current.element);
current = current.next;
if (current != null) {
result.append(", "); // Separate two elements with a comma
}
else {
result.append("]"); // Insert the closing ] in the string
}
}
return result.toString();
}
@Override /** Clear the list */
public void clear() {
size = 0;
head = tail = null;
}
@Override /** Return true if this list contains the element e */
public boolean contains(Object o) {
// Implement it in this exercise
Node
for (int i = 0; i < size; i++) {
if (current.element.equals(o))
return true;
current = current.next;
}
return false;
}
@Override /** Return the element from this list at the specified index */
public E get(int index) {
// Left as an assignment
}
@Override /** Returns the index of the first matching element in this list.
* Returns -1 if no match. */
public int indexOf(Object e) {
// Left as an assignment
}
@Override /** Returns the index of the last matching element in this list
* Returns -1 if no match. */
public int lastIndexOf(E e) {
// Left as an assignment
}
@Override /** Replace the element at the specified position in this list
* with the specified element. */
public E set(int index, E e) {
// Left as an assignment
}
@Override /** Override iterator() defined in Iterable */
public java.util.Iterator
return new LinkedListIterator();
}
private class LinkedListIterator
implements java.util.Iterator
private Node
@Override
public boolean hasNext() {
return (current != null);
}
@Override
public E next() {
E e = current.element;
current = current.next;
return e;
}
@Override
/* Removes from the underlying list the last element returned by this iterator */
public void remove() {
//Left as an assignment
}
}
private static class Node
E element;
Node
public Node(E element) {
this.element = element;
}
}
@Override /** Return the number of elements in this list */
public int size(){
return size;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
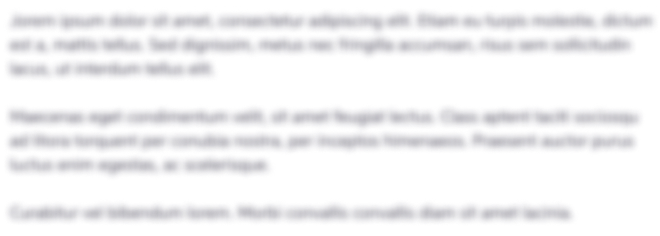
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started