Question
The Java HW This Assignment will give you experience modifying a reference-based implementation of an ADT.(JAVA) Modify the ListReferenceBased List class we discussed in lecture.
The Java HW
This Assignment will give you experience modifying a reference-based implementation of an ADT.(JAVA)
Modify the ListReferenceBased List class we discussed in lecture. Its description is given at the bottom and in your class notes. The ListReferenceBased List class should be modified by adding methods corresponding to the following UML: +replace(in oldValue:Object, in newValue:Object) : integer // replaces each occurrence of oldValue in the list with newValue // returns number of items replaced +equals(in aList:List) : boolean // returns true if aList has the same values in the same order as the current list // object; else returns false
You will also need the Node class, which is also given at the bottom. Additionally, you will need the ListInterface interface also given at the bottom. Note that there is also the need to use ListIndexOutOfBoundsException needed that you should use from the previous lab.
NOTE: Do not call any ListInterface methods from within the methods that you write.
Test your modified ListReferenceBased class by writing a driver program, with sample dialog displayed: What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 1 Enter a list of integers: 10 14 10 13 25 10 Enter a value to be modified: 10 Enter replacement value: 2 Modified list: 2 14 2 13 25 2 Number of items replaced: 3 What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 1 Enter a list of integers: 10 14 10 13 25 10 Enter a value to be modified: 9 Enter replacement value: 2 Modified list: 10 14 10 13 25 10 Number of items replaced: 0 What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 2 Enter first list to be compared: 10 14 10 13 25 10 Enter second list to be compared: 10 14 10 13 25 10 The two lists are equal. What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 2 Enter first list to be compared: 10 14 10 13 25 10 Enter second list to be compared: 10 14 10 13 25 The two lists are not equal. What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 2 Enter first list to be compared: 10 14 10 13 25 10 Enter second list to be compared: 10 14 10 11 25 10 The two lists are not equal. What you want to do? 1) Replace a value in a list of integers 2) Compare two lists of integers 3) Quit Enter your choice: 3 To turn in: Submit your program listing, along with a capture of a program run, using the data shown in this lab assignment description, on Canvas.
ListReferencedBased, Node, and ListInterface Information
=========
class Node { Object item; Node next; Node(Object newItem) { item = newItem; next = null; } // end constructor Node(Object newItem, Node nextNode) { item = newItem; next = nextNode; } // end constructor } // end class Node
public class ListReferencedBased implements ListInterface { // reference to linked list of items private Node head; private int numItems: // number of items in list // definitions of constructors and methods public ListReferencedBased() { numItems = 0; head = null; } // end default constructor public boolean isEmpty() { return numItems == 0; } public int size() { return numItems; } // end size }
private Node find(int index) { // // Locates a specified node in a linked list. // Precondition: index is the number of the desired // node. Assumes that 1 <= index <= numItems+1 // Postcondition: Returns a reference to the desired // node. // Node curr = head; for (int skip = 0; skip < index; skip++) { curr = curr.next; } // end for return curr; } // end find private Object get(int index) throws ListIndexOutOfBoundsException { if (index >= 0 && index < numItems) { // get reference to node, then data in node Node curr = find(index); Object dataItem = curr.item; return dataItem; } else { throw new ListIndexOutOfBoundsException(List index out of bounds on get); } // end if } // end get public void add(int index, Object item) throws ListIndexOutOfBoundsException { if (index >= 0 && index < numItems+1) { if (index == 0) { // insert the new node containing item at // beginning of list Node newNode = new Node(item, head); head = newNode; } else { Node prev = find(index-1); // insert the new node containing item after // the node that prev references Node newNode = new Node(item, prev.next); prev.next = newNode; } // end if numItems++; } else { throw new ListIndexOutOfBoundsException(List index out of bounds on add); } // end if } // end add public void remove(int index) throws ListIndexOutOfBoundsException { if (index >= 0 && index < numItems) { if (index == 0) { // delete the first node from the list head = head.next; } else { Node prev = find(index-1); // delete the node after the node that prev // references, save reference to node Node curr = prev.next; prev.next = curr.next; } // end if numItems; } else { throw new ListIndexOutOfBoundsException(List index out of bounds on remove); } // end if } // end remove public void removeAll() { // setting head to null causes list to be // unreachable and thus marked for garbage // collection head = null; numItems = 0; } // end removeAll } public interface ListInterface { // ******************************** // Inteface for the ADT List // ******************************** // list operations: public boolean isEmpty(); public int size(); public void add(int index, Object item) throws ListIndexOutOfBoundsException; public void remove(int index) throws ListIndexOutOfBoundsException; public Object get(int index) throws ListIndexOutOfBoundsException; public void removeAll(); } // end ListInteface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
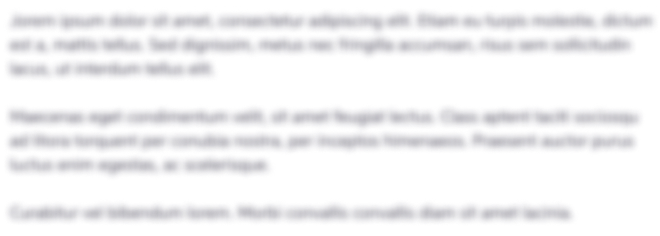
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started