Question
// The language is java, please put comments for better understanding Obtain example code files Shape.java, Circle2.java, Sphere.java, and CircleShapeApp.java from the downloaded files in
// The language is java, please put comments for better understanding
Obtain example code files Shape.java, Circle2.java, Sphere.java, and CircleShapeApp.java from the downloaded files in Ch8. Compile and execute the example and understand the polymorphism it performs. ****I have each of these pasted below.
Modify the application as follows:
-
Code a class called Cone inherited from class Circle. Cone will override the computeArea() and computeVolume() methods from its superclass to calculate the area and volume of a Cone object. Must apply code-reusability in the calculation formula.
-
Modify the driver code, CircleShapeApp.java, so it will also create an object of Cone with a height of 12.5 and diameter of 10.48. Modify the polymorphic calls to calculate and display the result of the calculations.
-
Instead of hard-coded data, modify your application to be interactive using JOptionPane, thus it will ask the user to enter proper data for different objects, continue to utilize the Validator class you have developed before to validate all wrong data entries, including negative and non-numerical data. Your program must continue to run until the user enters n to terminate the execution. You must also validate the user's entry so that only y and n are accepted.
Shape.java:
//abstract class Shape
public abstract class Shape { protected double x1, y1, x2, y2; public Shape() { x1 = y1 = x2 = y2 = 0.0; } public Shape(double x1, double y1, double x2, double y2) { this.x1 = x1; this.y1 = y1; this.x2 = x2; this.y2 = y2; }
public String toString() { String message = "(" + x1 + "," + y1 + "), (" + x2 + "," + y2 + ") "; return message; }
public abstract void computeArea(); // public abstract void computeVolume(); public abstract double getArea(); public abstract double getVolume(); }
Circle2.java
//abstract class CircleShape2
public abstract class CircleShape2 extends Shape { //Inheritance protected double radius;
public CircleShape2() { x1 = y1 = x2 = y2 = 0.0; } public CircleShape2(double x1, double y1, double x2, double y2) { super(x1, y1, x2, y2); //Call constructor of Shape } public CircleShape2(double radius) { this.radius = radius; } public void setRadius(double radius) { this.radius = radius; } public double getRadius() { return radius; } public void computeRadius() { radius = Math.sqrt((x1 - x2)*(x1 - x2) + (y1 - y2) * (y1 - y2)); } public String toString() { return super.toString() + "Radius: " + radius + " "; } }
Sphere.java
//subclass class Sphere inherites Circle
class Sphere extends Circle{ public Sphere() { super(); //call super class non-argument constructor } public Sphere(double radius) { super(radius); //call super class one-argument constructor } public Sphere(double x1, double y1, double x2, double y2) { super(x1, y1, x2, y2);//call super class four-argument constructor }
public void computeArea() { //compute sphere's area super.computeArea(); //call super class' method area = 4* area; }
public void computeVolume() { //compute sphere's valume super.computeArea(); volume = 4.0/3 * radius * area; } }
CircleShapeApp.java
//the driver class for circle shapes
public class CircleShapeApp{
public static void main(String[] args) { Circle circle = new Circle(12.98); Sphere sphere = new Sphere(25.55);
Shape shape = circle;
shape.computeArea(); System.out.println("circle area: " + shape.getArea()); shape.computeVolume(); System.out.println("circle volume: " + shape.getVolume());
shape = sphere; shape.computeArea(); System.out.println("Sphere area: " + shape.getArea()); shape.computeVolume(); System.out.println("Sphere volume: " + shape.getVolume()); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
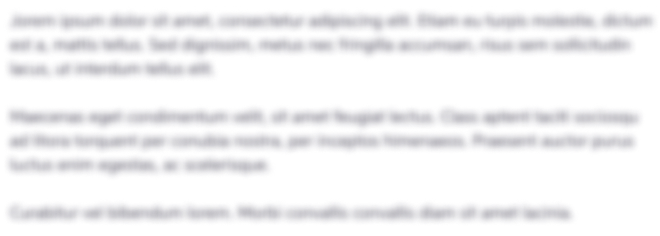
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started