Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The list works like this: entries begin at index 0 the size of the list is limited ( capped ) ; when the
The list works like this:
entries begin at index
the size of the list is limited capped; when the size is reached, no more entries can be added
entries can only be added to and removed from the front or back of the list
entries can be accessed in any position
Write a class that implements this interface. The class uses linked nodes to implement the list.
requirements:
Class Header and Instance Data Variables
Your class header and instance data variables will be:
public class LinkedFrontBackCappedList implements FrontBackCappedList
private Node head, tail;
Use the same nested Node class that is used in LList. I have provided a shell file for you to start with.
You CAN add additional variables other than head and tail.
For Full Credit:
You must use a head and tail reference!
Note that these are singlylinked nodes meaning they only have "next" and do not have "previous"
You have two of these singlylinked nodes: head and tail.
Implement an efficient solution when possible.
All of the methods in the class should be O or On
On methods will not receive full credit.
Make sure to account for special conditions such as empty lists and singleton lists.
Your code should not crash under these conditions.
Carefully consider what the values of head and tail will be for an empty and singleton list!
You must implement every method from the interface.
Follow the API descriptions from the interface file and the additional method characteristics listed below.
Class Requirements
I strongly recommend working on the methods below in the order listed. Just like in Project A the tester program relies on some methods to be working to test other methods. Hopefully writing these in order will help make sure that the tester is working as intended!
points constructorint
the parameter specifies the maximum size ie capacity that the list can be
points String toString
the output must contain:
the size
the capacity max size
a display of all elements in the list
when the list is not empty, the output should include the head and tail values
see the driver program for the format of the text representation your display must match this format!
Note: I strongly recommend writing the toString method right after the constructor because the tester will be much less useful without this method written!
points boolean isEmpty
points boolean isFull
points int size
points T getEntryint
null should be returned when an invalid position is passed in as a parameter
points void clear
points boolean addFrontT
when there is room to add an element, the new element is added to the beginning of the list; true is returned
when the list is full, no changes are made to the list and false is returned
points boolean addBackT
when there is room to add an element, the new element is added to the end of the list and true is returned
when the list is full, no changes are made to the list and false is returned
points T removeFront
the element is removed from the front
null is returned from an empty list
points T removeBack
null is returned from an empty list
points boolean contains T
points int indexOfT
points int lastIndexOfT
Style and Efficiency
points Style and Efficiency
use a head and tail reference of singlylinked nodes
ensure that all methods are O or On
follow Java coding conventions Links to an external site.
follow general best practices and principles of objectoriented programming
properly format and indent code
follow naming conventions for variables, classes, and methods
reduce duplicated code. To use the driver without the extra credit, you either need to comment out the runExtraCreditTests AND testCompareTo methods or update the class header and add an empty compareTo method in your list class. The tester won't compile otherwise.Remove package statements from all files so that no classes are in a named package.
Upload your java file.
file must compile no extra credit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
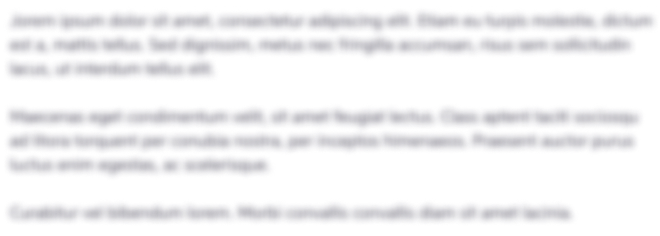
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started