Question
The main objective of this assignment is to implement the RANSAC algorithm for linear regression import numpy as np from sklearn import linear_model,
""" The main objective of this assignment is to implement the RANSAC algorithm for linear regression """
import numpy as np from sklearn import linear_model, datasets from matplotlib import pyplot as plt import json
def RANSAC(observed_data,threshold): """ observed_data: the set of obverserved data for fitting a linear regression model. threshold: the threshold is used to differentiate inliers from outliers by testing the error deviation. You can use a predefined percentage as the threshold as we show in the class. """ X,y=observed_data """ Write your codes here Find outliers and remove them from (X,y), return (newX,newY) """ #Keep the following code unchanged return (newX,newy)
""" Use the following code to test your RANSAC implementation. DON'T change this part. """ with open('ass3input.txt','r') as infile: indata=json.load(infile)
X=np.array(indata['X']) y=np.array(indata['y'])
newX,newy=RANSAC((X,y),0.01)
model = linear_model.LinearRegression() model.fit(newX, newy) yhat=model.predict(newX)
m_coef=model.coef_ m_intercept=model.intercept_
outdata={'coef':m_coef.tolist(),'intercept':m_intercept.tolist()} with open('ass3output.txt','w') as outfile: json.dump(outdata,outfile)
plt.scatter(X, y, color='yellowgreen', marker='.') plt.plot(X, yhat, color='navy', linestyle='-', linewidth=2, label='Linear regressor')
plt.show()
The data below is supposed to be in ass3input.txt, which is referenced in the code above
{"X": [[3.8250700159511686], [2.8013092244407325], [3.1350903919267568], [2.052294239917671], [2.0005816088198065], [2.4692655976341884], [2.4347269184589373], [2.9557818803822706], [3.6355449432817224], [2.5583415698407066], [2.333653149402417], [2.857934857941193], [3.006885070814933], [2.924507270880392], [3.165109332242483], [3.580847750721733], [1.8005711040436922], [2.4151051284482543], [3.7087603624763537], [3.1619761595315268], [2.6939076340612793], [3.764373401730665], [3.0073610570436906], [2.6165046088796746], [2.622904661475525], [3.3827383601455208], [2.9405645578905846], [3.6351334869216285], [2.7968578264796027], [3.1345583771190153], [3.3756570361738376], [2.4226077084677407], [3.2585677081389486], [2.7992324712950487], [3.1982318388286295], [2.765606423087821], [2.589225945285082], [2.406433218958898], [3.231731426414658], [3.726999319909056], [2.840926673094576], [2.8918326431114045], [2.3669137531677924], [2.045454371514693], [2.519101733638762], [3.031510834578404], [2.7429096076412174], [3.3857872511968794], [2.94546949209015], [2.9679521025790883], [3.2724162252955353], [2.6426017583623684], [2.598147364819576], [3.1980698055952126], [2.6565628574236047], [2.9592534348994928], [3.268481435481026], [2.6569991381955447], [3.050710844645635], [3.0922782488853384], [3.923091295647669], [3.7220854774446224], [2.9994111963230337], [2.807136128601728], [3.2086436405999885], [2.4641221584077426], [2.7969215490733843], [3.6108806129585647], [2.4231294308983617], [2.6823627396696033], [3.5582942661200936], [3.443076677073919], [2.330212519899814], [3.5859966090294084], [3.2357236782435193], [3.1046141654672206], [2.375875954256637], [2.8452458727074976], [3.5329940900463743], [4.296229233544027], [3.1797362701838345], [3.107207027392857], [3.640502602917031], [3.66559789133962], [2.6946514356096447], [2.950149184512562], [2.836568185649175], [3.319480190074941], [3.468496752405057], [3.7898193181147475], [2.6070983878016554], [3.205314682085448], [2.8783705085117983], [2.811309982965468], [2.954590432868027], [2.864385005954566], [3.097420799315048], [3.4670307254882564], [3.394921713023453], [2.5615840498168794], [0.3452671664838518], [-0.6638573931011081], [-0.7417602315025392], [0.6487946933187454], [0.33823145580942365], [0.2019062286299831], [0.15908402404219177], [-2.094247816222812], [0.34284631059528275], [-1.2125121036529087], [1.1825557977622998], [-0.0017850620187045423], [-0.8036189314182972], [-0.45165124861796846], [-0.4440563060990606], [-1.1252621770993945], [-0.1538858184730633], [0.5203871720401353], [-1.3638680246204355], [-0.3600684561454375], [0.20246222800689795], [-0.9356249358244432], [-0.4072451285196812], [1.5593455991881504], [-0.4618495049410465], [0.21233876041488856], [-1.7534134684624807], [-0.3152216669875888], [-0.3763598928082924], [-1.178783700330632], [0.26418463484258997], [0.3638469368228928], [-1.1156918267431322], [0.0891175300595829], [1.3097339728019273], [1.6924546010277466], [1.4236144293489872], [-1.4342137169891076], [0.9391726718903264], [-0.26070246025058924], [0.716332287869702], [0.47874513116694617], [1.8912873415956053], [-1.3122834112374318], [-0.6025696357683812], [0.7548167342810945], [0.21576096225357072], [-0.3918162398080484], [0.19583233720839927], [0.584932733083613], [0.027655053690957953], [-1.9014104727600916], [0.6252181869673088], [-0.7891408068160674], [0.39062079927072996], [0.923512919292642]], "y": [[4.376881751537914], [10.817335010405987], [-17.872823601713044], [-0.9512088988871619], [10.967124174447903], [-10.37861501426713], [-0.46607845303218065], [1.1131004431343543], [4.787764284936236], [2.94816791992415], [-14.160139481723721], [10.222586542752815], [-1.4073876501660134], [-2.892937254774406], [-6.08240262853939], [1.4107745129352294], [-8.571273624791655], [-19.259788262504454], [-9.141948999670657], [14.3810490915855], [17.76099029119441], [-12.143030001257365], [-12.231713718419243], [-9.88586753696928], [8.78302216877909], [-10.863813748013023], [-13.306034489012488], [-6.664182719344865], [11.051541228603792], [-7.978327479510294], [-10.006490129924147], [-14.283696014103718], [-8.457862191467646], [-1.2713659176842316], [-16.74677317176846], [-0.7566336881445292], [14.344410570198999], [7.260376379571463], [-9.07674720108572], [-4.772223910942364], [-4.178136515263589], [-6.642820757257919], [-0.5456306920869163], [-17.943418942862465], [-9.621381839590523], [-22.775790308416717], [10.905001439207826], [-8.208867526524429], [3.638322746598069], [9.853757771506295], [3.3359256553451875], [-18.18871050780231], [5.343920868914898], [11.534771228179613], [-2.9995302214941884], [7.115134344249384], [3.341673000539643], [9.080367824497314], [-5.50997279040369], [0.3597087621283359], [3.1092956232436304], [-10.095938711338604], [3.959351579143471], [-8.961216043867962], [-6.452544226173132], [-2.562823293823298], [10.423468875709014], [-8.367746712484097], [-2.6855480660414353], [-12.998855658177998], [-12.124540796270015], [14.35938408950453], [-6.685375365495749], [-13.46165003307529], [4.754117281122887], [-16.492443644090336], [-16.573942517698278], [-16.73313535623368], [-17.81508840286903], [4.838625068712405], [10.029072252016555], [-4.865110117157775], [2.2116154325240807], [5.0706278056717515], [-6.136613134797728], [-14.806597262219018], [-19.541992826743726], [-14.192839793847263], [6.171140098326964], [-12.808528790931298], [18.521322946903936], [-13.313380100221712], [-11.627230464871857], [-15.947436964009851], [-11.662193755009419], [-31.999955606872682], [-3.198400358350096], [8.10347763243007], [-15.026306922570614], [-10.522455743801384], [137.70325723202606], [42.26899974090343], [27.595862571881202], [161.10617687132185], [140.09989539826725], [128.10155571901873], [100.66170283739491], [-65.55962212194207], [139.01969583290733], [-12.428130965229524], [210.51953280792608], [88.77619500069193], [31.597410284194506], [61.75879813894896], [28.61623387821834], [0.9950930294902334], [86.47372829688436], [144.69438784869806], [0.08388823143500801], [65.00159842479007], [116.0638790540696], [23.60676804238757], [52.55117745421633], [230.6066217290814], [72.66593508046192], [88.47894931907577], [-37.3477425903521], [85.55494460333648], [64.26418001744325], [2.1463643465888964], [113.02999399871777], [133.79315785154418], [7.632978916563502], [112.23096321920507], [217.35422202501496], [235.4431309752856], [226.28976595805642], [-29.940131758094772], [200.4248631945613], [74.64778975939196], [165.58929448355843], [146.06376059990845], [267.86778931852206], [-4.163256482481428], [47.232967168593724], [177.9505379537885], [113.85421329690843], [51.481404602780245], [125.26151088249243], [145.86036516499328], [93.66925305109824], [-66.3078232599695], [133.99060645087957], [51.488096776557015], [135.87442827817188], [172.67616493198557]]}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
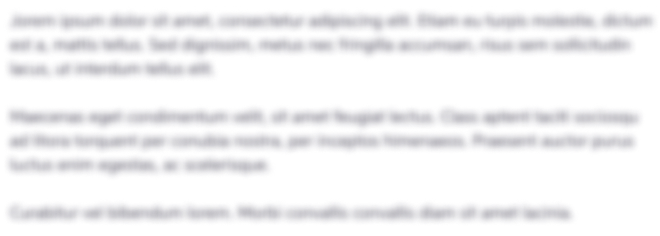
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started