Question
The mortgage finance officers at Frontier Savings and Loan have-asked you to create a mortgage calculator that customers can use to estimate, monthly mortgage payments.
The mortgage finance officers at Frontier Savings and Loan have-asked you to create a mortgage calculator that customers can use to estimate, monthly mortgage payments. Your project manager, Lisa Drummond, explains that the Web page should contain a form in which the customers select the type of loan, enter the loan amount, the number of monthly payments, the yearly interest rate, and indicate if they are full-time students. They then click a button to see what the monthly payment and total payments for the loan would be. You need JavaScript to accomplish this task. A function named monthly(), which is used to calculate the monthly payment, follows: function monthly(I, N, S) { //Inputs: //I : Yearly Interest Year in decimal (e.g. 8.5% = 0.085) //N : Number of monthly payments //S : Loan amount //Outputs: //returns monthly payments on the loan return (S*I/12*Math.pow(I/12+1, N))/(Math.pow(I/12+1,N)-1); } The monthly(I, N, S) function takes three parameter values, as documented above, and returns the value of the monthly payment for the loan. Once you know the value of the monthly payment, the total amount paid is simply the monthly payment multiplied by the number of monthly payments. Using the monthly() function, you'll complete the rest of the Web page for Frontier Savings and Loan. The following figure shows one possible solution to this assignment. To create the mortgage calculator Web page: 1. Create a project. Create an html file called mortgage.html, and a script file call main.js. Also, create a main.css file to be used for rendering your page. This is a similar structure to my demos, so you can review my videos if you are not clear about this. 2. Open mortgage.html file and create a form and give it a value fincalc for its name attribute. The form must contains the following fields (the layout of the form is up to you, but must include the text, labels and fields shown in the above diagram): Seven input fields used for the type of loan, loan amount, yearly interest rate, length of the loan, full-time student, monthly payment amount, and total payments. o Type of loan should be a collection of three radio buttons as shown in the above figure with personal as the default selection. o Loan amount will be supplied by the user and will have no default value. o Interest rate should have a default value of 7.5. o Length of loan should be a selection field with values of 5, 10, 15, 20, 25 and 30 and option 5 should be the default selected value. o Full-time student should be a checkbox that is not selected by default. o Monthly payment and total payments will be used as user output fields whose values will be supplied by your code. Two buttons: one labeled Calculate Monthly Payments (used to calculate the monthly and total payments) and the other labeled Reload (used to reset the form). Add an event handler to the Calculate Monthly Payments button such that it will call the function showVal() when the button is clicked. 3. Debugging step: Make sure your form is displayed correctly before moving to the next sections. 4. Link the external script file main.js to your mortgage.html file. 5. Add the monthly() function, described earlier, to your main.js file. 6. In your main.js, create a function named showVal() that performs the following tasks in the order specified: Extracts the value in the loan amount filed and stores in the variable loanAmount. It will then check the extracted value and if it is null, negative or 0, it produces a prompt dialog to the user as shown below and forces the user to enter a value for positive loan amount before it continues. NOTE: To accomplish this task, you must use a while loop. The loop should continually prompt the user, until the user enters a valid value (positive and non-zero) for the loan amount. Note that the dialog will look different depending on your operating system. Please do not concern yourself with the look of the dialog box. Debugging step: Display the loanAmount you just extracted in the Total Payment filed. Open your page and click the Calculate Monthly Payments button and make sure the correct loan amount is displayed on the Total Payment filed before moving to the next step. Note that at this point you are just using the Total Payment filed as a dummy display filed for debugging purpose. Extracts the values of interest rate and store in a variable. Convert the rate to decimal. For example, if the user entered 8 for interest rate you should divide it by 100 and store in the interest rate the resulting value of 0.08. Debugging step: Display the interest rate you just extracted and converted in the Total Payment filed and make sure it is correct before moving to the next step. Extract the length of the loan. Convert the length you extracted from number of years to number of months (multiply it by 12). Debugging step: Display the loan length you just extracted and converted in the Total Payment filed and make sure it is correct before moving to the next step. Extract the selected type of loan. Use the value you extracted to decide how much you need to add to the loan amount. For example, if personal is selected, then you will need to increase the loan amount by $1000. (See the figure above for the amounts associated with the other types of loan) Debugging step: Display the loan amount you just recomputed in the Total Payment filed. Make sure to do this for each loan type and check to see if the correct amount is added to the loan amount before moving to the next step. Extracts the full-time student flag and if it is checked, reduce the interest rate by 1%. Debugging step: Display the interest rate you just recomputed in the Total Payment filed. Make sure to do this for both full-time and not full-time and check to see if the correct interest rate is computed before moving to the next step. Make a call to the monthly() function with values you just extracted and recomputed and save the returned value in a variable. Debugging step: Display the returned value in the Monthly payment filed and make sure it is correct. To make sure it is correct, you should use a calculator and hand calculate the expected value and see if it is the same. It should also produce the same results as the figure above for the value used. Use the value returned by the monthly() function to compute the total amount of payments to the bank. This is simply the monthly payment multiplied by the number of monthly payments made during the length of the loan. Debugging step: Display the total payment you just computed in the Total payment filed and make sure it is correct. To make sure it is correct, you should use a calculator and hand calculate the expected value and see if it is the same. It should also produce the same results as the figure above for the value used. Format Monthly Payment and Total Payment such that they are produced with 2 digits after the decimal. At this point, if you have done everything correct, clicking the Calculate Monthly Payments should display correct values of Monthly and Total Payments. Test many different combinations and make sure it works correct before moving to the next step. Write statements that would check the monthly payment and if it is greater than $5000.00, it would produce the following dialog to the user: Debugging step: Change the filed values so that the monthly payment goes over $5000.0 and make sure the dialog box is displayed. 7. Add an event handler to the Reload button so that it resets the form when clicked. Also, make sure that if Reload is clicked, the user is presented with the following dialog and only if their response is OK, the page should reload. 8. Modify the form such that every time you click in the Monthly payments or Total Payments boxes, the ShowVal() function would execute. 9. Include the "Financial Calculator" title, along with a paragraph describing the purpose of the Web page and how to use the calculator, at the top of the Web page, located before the calculator as shown on the diagram. 10. Link your main.css to your page and add style rules to render the page with your own style. Make sure it is logical and related fields and grouped and easily distinguished. I recommend using a table to organize your elements on the page. Using a table to organize page content with many control elements often helps with organization and formatting of your page. 11. Check and make sure your page displays and works as described both inside the eclipse preview and in your web browser. 12. At this point you are ready to submit your assignment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
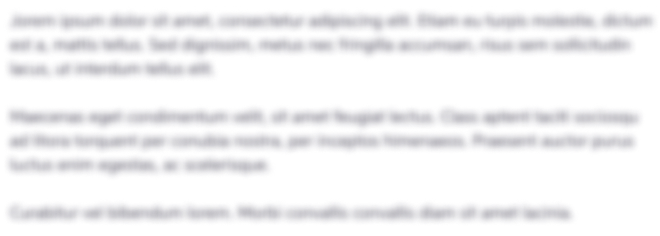
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started