Question
The Node class must be represented as a template. The Node class will require these private fields. T data: The data to hold in the
The Node class must be represented as a template.
The Node class will require these private fields.
- T data: The data to hold in the Node
- Node* next: A pointer to the next Node (if any) in the chain of Nodes.
- Node* prev: A pointer to the previous Node (if any) in the chain of Nodes.
Linked List
This class will require the following fields:
- Node* mHead: Points the first Node in the chain
- Node* mTail: Points the last Node in the chain
- int mSize: Keeps a count of the number of Nodes in the chain
Your LinkedList class must also support the following public member functions.
- LinkedList(): A default constructor sets both pointers to null and sets the size to 0.
- LinkedList(const LinkedList&): A copy constructor that takes a LinkedList object and performs a deep copy of the passed list into this list.
- ~LinkedList(): A destructor that performs pop_front on the list while the list is not empty.
- operator=(const LinkedList&): LinkedList& An overloaded assignment operator that deletes the current array and replaces it with deep copy of the passed list.
- size() const: int: Returns the size of the LinkedList.
- push_back(const T): void: Creates a new Node and assigns it to the end of the list of Nodes while updating the size by 1.
- push_front(const T): void: Creates a new Node and assigns it to the front of the list of Nodes while updating the size by 1.
- pop_back(): T: Deletes the Node at the end of the list (or throws an error if this is not possible). Decreases size by 1. Returns the deleted element.
- pop_front(): T: Deletes the Node at the front of the list (or throws an error if this is not possible). Decreases size by 1. Returns the deleted element.
- at(int) const: T: Returns the element stored at the specified index. If the index is out-of-bounds (either for being negative or greater than/equal the size of the list), throw invalid\_argument("Index out of bounds.");. This will require you to include the stdexcept library. This method should operate at O(1)
for accessing the first or last element in a list and at O(n)
for any element other than the first or the last.
- front() const: T: Returns the first element.
- back() const: T: Returns the last element.
Return to the Books object.
Return to your Books code. Find Books.h and remove the import statements to vector.hpp and replace those with the local version of LinkedList.hpp that you made.
- Add a menu option to add to the beginning of the list.
- Add a menu option to remove the first Book in the list.
- Add a menu option to remove the last Book in the list.
- Add a menu option to remove every Book in the list.
Test your linked list application. Add a Book to the list 1,000 times. Add a second Book to the list 1,000 times. Remove the first Book in the list. Remove the last Book in the list. Verify that you have 1,998 Books in the list.
After you verify that there are 1,998 Books in the list, clear the list using your newest menu option. Verify that you have 0 Books in the list.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
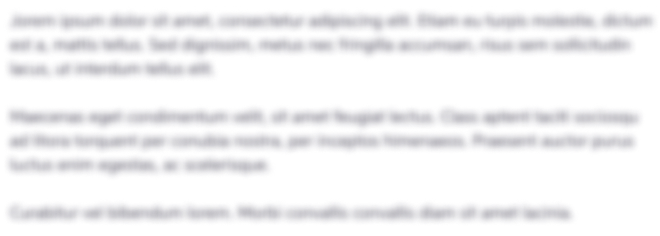
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started