Question
The objectives of this homework assignment are: 1. Understand basic Graphical User Interface (GUI) objects 2. Write a GUI application a. Identify the GUI objects
The objectives of this homework assignment are: 1. Understand basic Graphical User Interface (GUI) objects 2. Write a GUI application a. Identify the GUI objects that are necessary for the application b. Organize the positions and functions of these GUI objects for the application c. Design the application such that the user interface is sensible and user-friendly d. Design the application such that the user interface is robust and can recover from errant response from the user 3. Make use of event-driven programming to replace while loops a. Learn how Javas delegation-based event model allows a GUI application to be always ready to receive the next user input 4. Familiarize with the javax.swing and java.AWT packages and layout management 5. Familiarize with a searching method 6. Master code documentation, compilation, and execution 7. Expose to Java syntax, programming styles, and a Java classes. The Graphical User Interface (GUI) is an important component in software. It provides a userfriendly communication medium between the users and an application. Users provide input to the application; the application processes the input, performs some computations and provides some feedback to the users. The role of the GUI is to provide the bridge between the user and the application. Java provides a variety of tools to help us build a GUI easily. The Swing library provides a rich set of graphical components. For example, JFrame, JPanel, and JDialog are some of the classes that can help you put graphical component into your interface. JButton, JTextfield, JRadioButton, and JCheckBox are graphical components you might use for your GUI. Layout Managers help you organize your interface components in a natural and user-friendly manner. Objectives Problem Description 2 Java also provides an event-handling mechanism that facilitates user centric programming. Any action performed by the user is captured and notification is send to the application. The application must respond to these actions accordingly. You can write action listeners to process different actions that you think are meaningful to your application. For this assignment, you will update your solutions from the previous Vending Machine Assignment to include a Graphical User Interface while still mimicking the operations of several vending machines. More specifically, the program simulates what happens when the user chooses one of the vending machines, inputs money, searches for and picks an item from the vending machine. Assume there are two vending machines: one for drinks and one for snacks. Each vending machine contains several items. The name, price, and quantity of each item are given in two text files; one named drinks.txt for the drinks vending machine and the other named snacks.txt for the snacks vending machine. The format of the input values is comma-separated. The items listed should be organized in the file with the following order: name, price, quantity.
/VendingMachine.java
import java.io.*;
import java.util.Scanner;
/*************************************************************************
* Simulates a real life vending machine with stock read from a file.
*
* CSCE 155A Fall 2017
*
* @file VendingMachine.java
* @author Jeremy Suing
* @version 1.0
* @date November 3, 2017
*************************************************************************/
public class VendingMachine {
// data members
private Item[] stock; // Array of Item objects in machine
private double money; // Amount of revenue earned by machine
private double userMoney=-1; //to store the user input money
/*********************************************************************
* This is the constructor of the VendingMachine class that take a file name
* for the items to be loaded into the vending machine.
*
* It creates objects of the Item class from the information in the file to
* populate into the stock of the vending machine. It does this by looping
* the file to determine the number of items and then reading the items and
* populating the array of stock.
*
* @param filename
* Name of the file containing the items to stock into this
* instance of the vending machine.
* @throws FileNotFoundException
* If issues reading the file.
*********************************************************************/
public VendingMachine(String filename) throws FileNotFoundException {
// Open the file to read with the scanner
File file = new File(filename);
Scanner scan = new Scanner(file);
// Determine the total number of items listed in the file
int totalItem = 0;
while (scan.hasNextLine()) {
scan.nextLine();
totalItem++;
} // End while another item in file
// Create the array of stock with the appropriate number of items
stock = new Item[totalItem];
scan.close();
// Open the file again with a new scanner to read the items
scan = new Scanner(file);
int itemQuantity = -1;
double itemPrice = -1;
String itemDesc = "";
int count = 0;
String line = "";
// Read through the items in the file to get their information
// Create the item objects and put them into the array of stock
while (scan.hasNextLine()) {
line = scan.nextLine();
String[] tokens = line.split(",");
try {
itemDesc = tokens[0];
itemPrice = Double.parseDouble(tokens[1]);
itemQuantity = Integer.parseInt(tokens[2]);
stock[count] = new Item(itemDesc, itemPrice, itemQuantity);
count++;
} catch (NumberFormatException nfe) {
System.out.println("Bad item in file " + filename + " on row "
+ (count + 1) + ".");
}
} // End while another item in file
scan.close();
// Initialize the money data variable.
money = 0.0;
} // End VendingMachine constructor
// End VendingMachine class definition
// setter and getter method
// method to handle the vending transaction
public void vend(Item[] userMachine, Scanner scnr) {
if(userMoney==-1){
userMoney = getMoneyInput(scnr);
}
if (userMoney != -1) {
System.out.printf("you have $%.2f to spend,Please make a selection (enter 0 to exit) ",userMoney);
int choice=getUserChoice(scnr);
if(choice==0){
/**
* didnt buy anything from machine
*/
System.out.printf("%s. Your change is $%.2f ",outputMessage(5),userMoney);
/**
* resetting the user money
*/
userMoney=-1;
}else{
if(choice<0 || choice>userMachine.length){
/**
* Invalid selection of item, displays the error message and exits to the main menu
*/
System.out.println(outputMessage(3));
System.out.printf("Your change is $%.2f",userMoney);
userMoney=-1;
}else{
if(userMachine[choice-1].getQuantity()==0){
/**
* Out of stock, prompt the user to enter a new selection
*/
System.out.println(outputMessage(4));/*out of stock*/
vend(userMachine, scnr); /*recalling the method to prompt the user again*/
}else {
if(userMachine[choice-1].getPrice()>userMoney){
/**
* Insufficient money
*/
System.out.println(outputMessage(1));
double additionalMoney=getMoneyInput(scnr);
if(additionalMoney==-1){
/**
* Didn't buy anything from machine
*/
System.out.printf("%s. Your change is $%.2f ",outputMessage(5),userMoney);
userMoney=-1;
}else{
/**
* Additional money received
*/
userMoney+=additionalMoney;
vend(userMachine, scnr);
}
}else{
/**
* A vending transaction taking place
*/
String item=userMachine[choice-1].getItemDesc();
double price=userMachine[choice-1].getPrice();
double change=userMoney-userMachine[choice-1].getPrice();
/**
* Adding money to the vending machine (business)
*/
money+=price;
/**
* updating the quantity
*/
userMachine[choice-1].setQuantity(userMachine[choice-1].getQuantity()-1);//updating the quantity
System.out.printf("You bought %s for $%.2f, your change is $%.2f",item,price,change);
/**
* resetting the user money
*/
userMoney=-1;
}
}
}
}
}
}
// returns the proper message if vend has an issue
public String outputMessage(Integer message) {
if (message == 1) {
return "You do not have enough money. Please add more money or exit.";
} else if (message == 2) {
return "Invalid input... try again!";
}else if(message==3){
return "Invalid choice";
}else if(message==4){
return "Sorry the machine is currently out of that item.";
}else if(message==5){
return "You did not buy anything from this machine";
}
return null;
}
/**
* A helper method designed to get valid money input from the user
*/
public double getMoneyInput(Scanner scnr) {
double money = -1;
System.out
.println("Please enter some money into the machine.(Enter -1 to exit.)");
try {
money = Double.parseDouble(scnr.nextLine());
if(money!=-1 && money<=0){
/**
* if the user tries to enter money less than 0
*/
throw new Exception();
}
} catch (Exception e) {
System.out.println("Invalid input... try again!");
return getMoneyInput(scnr);
}
return money;
}
/**
* helper method to get a valid choice from user
*/
public int getUserChoice(Scanner scnr) {
int choice = -1;
try {
choice = Integer.parseInt(scnr.nextLine());
} catch (Exception e) {
System.out.println(outputMessage(2));
return getUserChoice(scnr);
}
return choice;
}
// Creates and prints the menu of the vending machine
public void printMenu(Item[] machineChoice, Scanner scnr) {
System.out.println("Item#\tItem\tPrice\tQty");
for (int i = 0; i < machineChoice.length; i++) {
System.out
.printf("%d\t%s\t%.2f\t%d ", (i + 1),
machineChoice[i].getItemDesc(),
machineChoice[i].getPrice(),
machineChoice[i].getQuantity());
}
/**
* Scanning a blank line to clear the buffer.
*/
scnr.nextLine();
/**
* Calling the vend() method
*/
vend(machineChoice, scnr);
}
public Item[] getStock() {
return stock;
}
public double getMoney() {
return money;
}
}
//VendingMachineDriver.java
import java.io.FileNotFoundException;
import java.util.Scanner;
public class VendingMachineDriver {
public static void main(String[] args) {
boolean exit = false;
Scanner scnr = new Scanner(System.in);
VendingMachine drinks = null;// creates a VendingMachine object for the
// drink machine
VendingMachine snacks = null;// creates a VendingMachine object for the
// snacks machine
// reads in the correct file and sends it to VendingMachine constructor
// to create a stock[]
// of each file
try {
drinks = new VendingMachine("drinks.txt");
snacks = new VendingMachine("snacks.txt");
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("Welcome to Matt's Super Vending Machines!");
System.out.println(" I sense that you are hungry or thirsty...");
// allows user to chose their machine or exit the program
while (!exit) {
System.out.println(" Please select a vending machine:");
System.out.print("A-Drinks, B-Snacks, X-Exit: ");
String machineChoice = scnr.next();
if (machineChoice.equalsIgnoreCase("a")) {
// pass scanner as parameter, since the Zybook can only
// indentify one scanner
drinks.printMenu(drinks.getStock(), scnr);
} else if (machineChoice.equalsIgnoreCase("b")) {
// pass scanner as parameter, since the Zybook can only
// indentify one scanner
snacks.printMenu(snacks.getStock(), scnr);
} else if (machineChoice.equalsIgnoreCase("x")) {
exit = true;
break;
} else {
System.out.println("Invalid selection try again.");
}
}
// gets the money earned from snack machine and drink machine and
// displays it for the total earnings
System.out
.printf(" The vending machine has made $%.02f. Thank you for your business!",
(snacks.getMoney() + drinks.getMoney()));
scnr.close();
}
}
//Item.java
public class Item {
protected String itemDesc;
protected double price;
protected int quantity;
/**
* @return the itemDesc
*/
public String getItemDesc() {
return itemDesc;
}
/**
* @param itemDesc
* the itemDesc to set
*/
public void setItemDesc(String itemDesc) {
this.itemDesc = itemDesc;
}
/**
* @return the price
*/
public double getPrice() {
return price;
}
/**
* @param price
* the price to set
*/
public void setPrice(double price) {
this.price = price;
}
/**
* @return the quantity
*/
public int getQuantity() {
return quantity;
}
/**
* @param quantity
* the quantity to set
*/
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public Item(String itemDesc, double price, int quantity) {
this.itemDesc = itemDesc;
this.price = price;
this.quantity = quantity;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((itemDesc == null) ? 0 : itemDesc.hashCode());
result = (int) (prime * result + price);
result = prime * result + quantity;
return result;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Item other = (Item) obj;
if (itemDesc == null) {
if (other.itemDesc != null)
return false;
} else if (!itemDesc.equals(other.itemDesc))
return false;
if (price != other.price)
return false;
if (quantity != other.quantity)
return false;
return true;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return "Item [itemDesc=" + itemDesc + ", price=" + price
+ ", quantity=" + quantity + "]";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
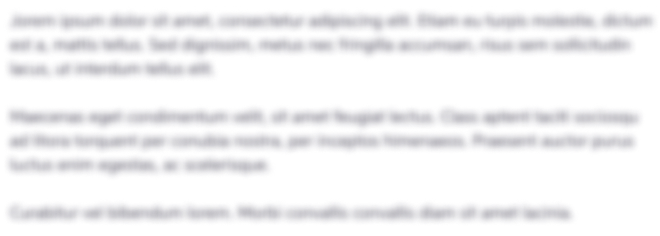
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started