Question
The Person class This class is a basic representation of a person, storing name and social security number. Attributes Type Name Description str name Full
The Person class
This class is a basic representation of a person, storing name and social security number.
Attributes
Type | Name | Description |
str | name | Full name of the person. |
str | ssn | Private attribute of social security number formatted as "123-45-6789". |
Methods
Type | Name | Description |
str | get_ssn(self) | Getter method for accessing social security number. |
Special methods
Type | Name | Description |
str | __str__(self) | Returns a formatted summary of the person as a string. |
str | __repr__(self) | Returns the same formatted summary as __str__. |
bool | __eq__(self, other) | Checks for equality by comparing only the ssn attributes. |
get_ssn(self)
Getter method for accessing the private social security number attribute.
Output | |
str | Social security number. |
__str__(self), __repr__(self)
Returns a formatted summary of the person as a string.
The format to use is: Person(name, ***-**-last four digits)
Output | |
str | Formatted summary of the person. |
__eq__(self, other)
Determines if two objects are equal. For instances of this class, we will define equality when the SSN of one object is the same as SSN of the other object. You can assume at least one of the objects is a Person object.
Input (excluding self) | ||
many | other | The other object to check for equality with. |
Output | |
bool | True if other is a Person object with the same SSN, False otherwise. |
Section 7: The Staff class
This class inherits from the Person class but adds extended functionality for staff members. Attributes, methods, and special methods inherited from Person are not listed in this section.
Attributes
Type | Name | Description |
Staff | supervisor | A private attribute for this person's supervisor. By default, set to None. |
Methods
Type | Name | Description |
str | id(self) | Property method for generating staff's id. |
(many) | setSupervisor(self, new_supervisor) | Updates the private supervisor attribute. |
(many) | getSupervisor(self) | Property method for getting the supervisor. |
(many) | applyHold(self, student) | Applies a hold on a student object. |
(many) | removeHold(self, student) | Removes a hold on a student object. |
(many) | unenrollStudent(self, student) | Sets a student's status to not active. |
Student | createStudent(self, person) | Creates a Student object from a Person object |
Special methods
Type | Name | Description |
str | __str__(self) | Returns a formatted summary of the staff as a string. |
str | __repr__(self) | Returns the same formatted summary as __str__. |
id(self)
Property method (behaves like an attribute) for generating staff's id.
The format should be: 905+initials+last four numbers of ssn. (e.g.: 905abc6789). Ignore the security flaws this generation method presents and assume ids are unique.
Output | |
str | Generated id for the staff member. |
setSupervisor(self, new_supervisor)Updates the private supervisor attribute.
Input (excluding self) | ||
Staff | new_supervisor | The new value to set for the supervisor attribute. |
Output | |
str | "Completed!" |
None | Nothing is returned if new_supervisor is not a Staff object. |
getSupervisor(self)
Property method (behaves like an attribute) for getting the supervisor.
Output | |
Staff | Current value of supervisor. |
applyHold(self, student)
Applies a hold on a student object (set the student's hold attribute to True).
Input (excluding self) | ||
Student | student | The student to apply a hold to. |
Output | |
str | "Completed!" |
None | Nothing is returned if student is not a Student object. |
removeHold(self, student)
Removes a hold on a student object (set the student's hold attribute to False).
Inputs (excluding self) | ||
Student | student | The student to remove a hold from. |
Output | |
str | "Completed!" |
None | Nothing is returned if student is not a Student object. |
unenrollStudent(self, student)
Unenrolls a student object (set the student's active attribute to False).
Inputs (excluding self) | ||
Student | student | The student to unenroll. |
Output | |
str | "Completed!" |
None | Nothing is returned if student is not a Student object. |
createStudent(self, person)
Creates a Student object from a Person object. The new student should have the same information (name, ssn) as the person and always starts out as a freshman ("Freshman").
Input | ||
Person | person | The Person object to make a Student object from. |
Output | ||
Student | The new student created from the input Person object. |
__str__(self), __repr__(self)
Returns a formatted summary of the staff member as a string.
The format to use is: Staff(name, id)
Outputs (normal) | |
str | Formatted summary of the staff member. |
======================================================
class Person:
'''
>>> p1 = Person('Jason Lee', '204-99-2890')
>>> p2 = Person('Karen Lee', '247-01-2670')
>>> p1
Person(Jason Lee, ***-**-2890)
>>> p2
Person(Karen Lee, ***-**-2670)
>>> p3 = Person('Karen Smith', '247-01-2670')
>>> p3
Person(Karen Smith, ***-**-2670)
>>> p2 == p3
True
>>> p1 == p2
False
'''
def __init__(self, name, ssn):
# YOUR CODE STARTS HERE
pass
def __str__(self):
# YOUR CODE STARTS HERE
pass
__repr__ = __str__
def get_ssn(self):
# YOUR CODE STARTS HERE
pass
def __eq__(self, other):
# YOUR CODE STARTS HERE
pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
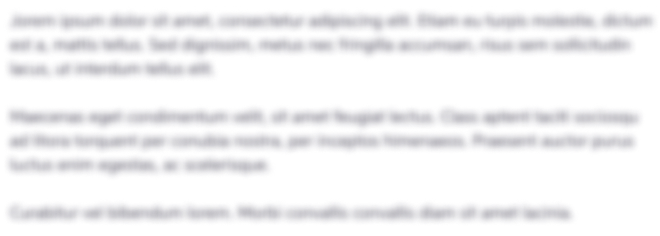
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started