Question
The Phone Directory Write a program for a phone directory by reusing the cStrTools module of part one and adding a Phone module and a
The Phone Directory
Write a program for a phone directory by reusing the cStrTools module of part one and adding a Phone module and a directory main module.
This program should be set up using a title for the phone directory and a file name for a data file. (see the directory module)
the data file
The data file holds records of a phone number in a tab separated format as follows:
[Name]\t[areaCode]\t[prefix]\t[number]
For example the following information:
Fred Soley (416)555-0123
is kept in the file as follows:
Fred Soley\t416\t491\t0123
Hint: to read the above record use the following fscanf format fscanf(fptr, "%[^\t]\t%s\t%s\t%s ",name,area,prefix,number)
*Below The data file ( phones.txt )
Jango Fett 905 555 6016 Padme Amidala 416 555 1464 Q'ira 647 555 9446 Firmus Piett 301 555 7279 Wedge Antilles 217 555 6370 Mon Mothma 905 555 4329 Count Dooku 416 555 7110 Nien Nunb 647 555 5708 Ponda Baba 301 555 3388 Max Rebo 217 555 4953 Salacious B. Crumb 905 555 4613 Enfys Nest 416 555 4962 Figrin D'an and the Modal Nodes 647 555 7124 Wicket W. Warrick 301 555 5476 Poe Dameron 217 555 8522 Qui-Gon Jinn 905 555 7446 Bib Fortuna 416 555 1293 Finn 647 555 0952 Kylo Ren 301 555 3302 Chirrut Imwe 217 555 0619 Mace Windu 905 555 6210 Chewbacca 416 555 1079 Jabba the Hut 647 555 3445 Greedo 301 555 2246 Lando Calrissian 217 555 7110 Darth Maul 905 555 1479 Obi-Wan Kenobi 416 555 1479 Rey 647 555 8396 Luke Skywalker 301 555 0630 Princess Leia 217 555 8508 Yoda 217 555 5979 Boba Fett 905 555 9382 Darth Vader 416 555 5011 Han Solo 647 555 5144 C3PO 301 555 2504 R2D2 217 555 6707
the maximum length for a name is 50 characters.
Execution
- When running, after showing the title, the program should prompt for a partial name entry.
- After receiving the partial name the program should search through the names in the file and if a name is found containing the partial entry, the matching phone recored is displayed.
- Nothing is displayed if no match is found.
- If the user enters '!' the program exits.
- If the data file could not be opened the program exits displaying an error message
- A thank you message is displayed at the end of the program.
For output formatting and messages, see the execution samples
Phone module
Only one mandatory function is required for the Phone module:
// runs the phone directory appication void phoneDir(const char* programTitle, const char* fileName);
You are free to create any function you find necessary to accomplish this task.
Tester Program
#include "Phone.h" using namespace sdds; int main() { phoneDir("Star Wars", "phones.txt"); return 0; }
Execution example (existing data file)
Star Wars phone direcotry search ------------------------------------------------------- Enter a partial name to search (no spaces) or enter '!' to exit > lukE Luke Skywalker: (301) 555-0630 Enter a partial name to search (no spaces) or enter '!' to exit > sky Luke Skywalker: (301) 555-0630 Enter a partial name to search (no spaces) or enter '!' to exit > fett Jango Fett: (905) 555-6016 Boba Fett: (905) 555-9382 Enter a partial name to search (no spaces) or enter '!' to exit > feT Jango Fett: (905) 555-6016 Boba Fett: (905) 555-9382 Enter a partial name to search (no spaces) or enter '!' to exit > Jack Enter a partial name to search (no spaces) or enter '!' to exit > ! Thank you for using Star Wars directory.
unsuccessful execution
#include "Phone.h" using namespace sdds; int main() { phoneDir("Star Wars", "badfile.txt"); return 0; }
unsuccessful Execution example (data file not found)
Star Wars phone direcotry search ------------------------------------------------------- badfile.txt file not found! Thank you for using Star Wars directory. D:\Users\phard\Documents\Seneca\oop244\DEV\Workshops\WS01\DIY\Debug\DIY.exe (process 8424) exited with code 0. Press any key to close this window . . .
============================================================================================================================
For Reusing cStrTools module
< cStrTools.cpp >
#define _CRT_SECURE_NO_WARNINGS #include#include "cStrTools.h" using namespace std; namespace sdds { // returns the lower case value of a character char toLower(char ch) { if (ch >= 'A' && ch <= 'Z') ch += ('a' - 'A'); return ch; } // compares s1 and s2 cStrings and returns: // > 0 if s1 > s2 // < 0 if s1 < s3 // == 0 if s1 == s2 int strCmp(const char* s1, const char* s2) { int i; for (i = 0; s1[i] && s2[i] && s1[i] == s2[i]; i++); return s1[i] - s2[i]; } // compares s1 and s2 cStrings upto len characters and returns: // > 0 if s1 > s2 // < 0 if s1 < s3 // == 0 if s1 == s2 int strnCmp(const char* s1, const char* s2, int len) { int i = 0; while (i < len - 1 && s1[i] && s2[i] && s1[i] == s2[i]) { i++; } return s1[i] - s2[i]; } // returns the length of str int strLen(const char* str) { int len; for (len = 0; str[len]; len++); return len; } // if "find" is found in "str" it will return the addres of the match // if not found it will returns nullptr (zero) const char* strStr(const char* str, const char* find) { const char* faddress = nullptr; int i, flen = strLen(find), slen = strLen(str); for (i = 0; i <= slen - flen && strnCmp(&str[i], find, flen); i++); if (i <= slen - flen) faddress = &str[i]; return faddress; } // copies src to des void strCpy(char* des, const char* src) { int i; for (i = 0; src[i]; i++) des[i] = src[i]; des[i] = 0; } // returns true if ch is alphabetical int isAlpha(char ch) { return (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z'); } // returns true if ch is a whitespace character int isSpace(char ch) { return ch == ' ' || ch == '\t' || ch == ' ' || ch == '\v' || ch == '\f' || ch == ' '; } // removes the non-alphabetic characters from the begining and end of a word void trim(char word[]) { int i; while (word[0] && !isAlpha(word[0])) { strCpy(word, word + 1); } i = strLen(word); while (i && !isAlpha(word[i - 1])) { word[i-- - 1] = 0; } } // copies the lower case version of the source into des. void toLowerCaseAndCopy(char des[], const char source[]) { int i = 0, j = 0; for (; source[i] != 0; i++) { des[j++] = toLower(source[i]); } des[j] = 0; } }
#ifndef CSTRTOOLS_H #define CSTRTOOLS_H namespace sdds { char toLower(char ch); int strCmp(const char* s1, const char* s2); int strnCmp(const char* s1, const char* s2, int len); int strLen(const char* str); const char* strStr(const char* str, const char* find); void strCpy(char* des, const char* src); int isAlpha(char ch); int isSpace(char ch); void trim(char word[]); void toLowerCaseAndCopy(char des[], const char source[]); } #endif
Here is all file I need
cStrTools.h cStrTools.cpp Phone.h Phone.cpp direcotry.cpp phones.txt
Step by Step Solution
There are 3 Steps involved in it
Step: 1
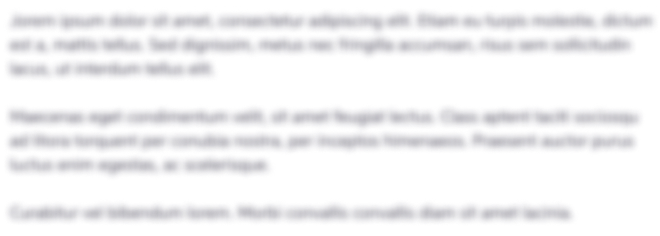
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started