Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
/ / The Planting Synchronization Problem / / import java.util.Random; import java.util.concurrent.Semaphore; public class Planting { public static void main ( String args [ ]
The Planting Synchronization Problem import java.util.Random; import java.util.concurrent.Semaphore; public class Planting public static void mainString args int i; Create Student, TA Professor threads TA ta new TA; Professor prof new Professorta; Student stdnt new Studentta; Start the threads prof.start; tastart; stdntstart; Wait for prof to call it quits try profjoin; catchInterruptedException e; Terminate the TA and Student Threads tainterrupt; stdntinterrupt; class Student extends Thread TA ta; public StudentTA taThread ta taThread; public void run whiletrue System.out.printlnStudent: Must wait for TA tagetMAX holes ahead"; Can dig a hole lets get the shovel System.out.printlnStudent: Got the shovel"; try sleepintMathrandom; catch Exception e break; Time to fill hole taincrHoleDug; hole filled increment the number System.out.printlnStudent: Hole tagetHoleDug Dug"; System.out.printlnStudent: Letting go of the shovel"; ifisInterrupted break; System.out.printlnStudent is done"; class TA extends Thread Some variables to count number of holes dug and filled the TA keeps track of things private int holeFilledNum; number of the hole filled private int holePlantedNum; number of the hole planted private int holeDugNum; number of hole dug private final int MAX; can only get holes ahead add semaphores the professor lets the TA manage things. public int getMAX returnMAX; public void incrHoleDug holeDugNum; public int getHoleDug returnholeDugNum; public void incrHolePlanted holePlantedNum; public int getHolePlanted returnholePlantedNum; public TA Initialise things here public void run whiletrue System.out.printlnTA: Got the shovel"; try sleepintMathrandom; catch Exception e break; Time to fill hole holeFilledNum; hole filled increment the number System.out.printlnTA: The hole holeFilledNum has been filled"; System.out.printlnTA: Letting go of the shovel"; ifisInterrupted break; System.out.printlnTA is done"; class Professor extends Thread TA ta; public ProfessorTA taThread ta taThread; public void run whiletagetHolePlanted try sleepintMathrandom; catch Exception e break; Time to plant taincrHolePlanted; the seed is planted increment the number System.out.printlnProfessor: All be advised that I have completed planting hole tagetHolePlanted; System.out.printlnProfessor: We have worked enough for today"; Code is in C
The Planting Synchronization Problem
import java.util.Random;
import java.util.concurrent.Semaphore;
public class Planting
public static void mainString args
int i;
Create Student, TA Professor threads
TA ta new TA;
Professor prof new Professorta;
Student stdnt new Studentta;
Start the threads
prof.start;
tastart;
stdntstart;
Wait for prof to call it quits
try profjoin; catchInterruptedException e;
Terminate the TA and Student Threads
tainterrupt;
stdntinterrupt;
class Student extends Thread
TA ta;
public StudentTA taThread
ta taThread;
public void run
whiletrue
System.out.printlnStudent: Must wait for TA tagetMAX holes ahead";
Can dig a hole lets get the shovel
System.out.printlnStudent: Got the shovel";
try sleepintMathrandom; catch Exception e break; Time to fill hole
taincrHoleDug; hole filled increment the number
System.out.printlnStudent: Hole tagetHoleDug Dug";
System.out.printlnStudent: Letting go of the shovel";
ifisInterrupted break;
System.out.printlnStudent is done";
class TA extends Thread
Some variables to count number of holes dug and filled the TA keeps track of things
private int holeFilledNum; number of the hole filled
private int holePlantedNum; number of the hole planted
private int holeDugNum; number of hole dug
private final int MAX; can only get holes ahead
add semaphores the professor lets the TA manage things.
public int getMAX returnMAX;
public void incrHoleDug holeDugNum;
public int getHoleDug returnholeDugNum;
public void incrHolePlanted holePlantedNum;
public int getHolePlanted returnholePlantedNum;
public TA
Initialise things here
public void run
whiletrue
System.out.printlnTA: Got the shovel";
try sleepintMathrandom; catch Exception e break; Time to fill hole
holeFilledNum; hole filled increment the number
System.out.printlnTA: The hole holeFilledNum has been filled";
System.out.printlnTA: Letting go of the shovel";
ifisInterrupted break;
System.out.printlnTA is done";
class Professor extends Thread
TA ta;
public ProfessorTA taThread
ta taThread;
public void run
whiletagetHolePlanted
try sleepintMathrandom; catch Exception e break; Time to plant
taincrHolePlanted; the seed is planted increment the number
System.out.printlnProfessor: All be advised that I have completed planting hole
tagetHolePlanted;
System.out.printlnProfessor: We have worked enough for today";
Code is in C
Step by Step Solution
There are 3 Steps involved in it
Step: 1
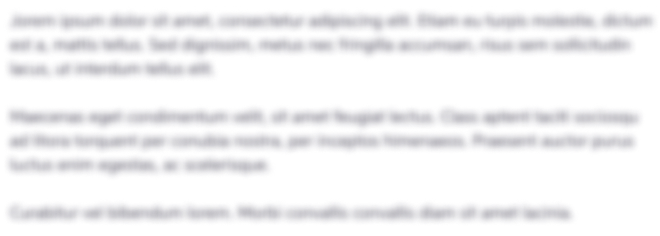
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started