Question
The PPM image format You will work with Plain PPM format images. This is one of a family of simple open source image formats, designed
The PPM image format You will work with Plain PPM format images. This is one of a family of simple open source image formats, designed to be read and written easily by C programs. See the PPM specification for full details of PPM and Plain PPM. A Plain PPM file consists of ASCII text: P3 # comment1 # ... # commentN width height max r1 g1 b1 r2 g2 b2 r3 g3 b3 ...
Requirements
2 - PPM functions Implement in steg.c the following functions. You may also write additional helper functions or type declarations if you like. The template includes some already, e.g. struct Pixel and readPPM, but you can rename or remove these provided you implement struct PPM, getPPM, showPPM, encode and decode. void showPPM(const struct PPM *img); To output the PPM image img to stdout (e.g. by using printf), following the syntax in the PPM specification. You should test this function by using it to write out an image loaded with getPPM. The template code's main function has an extra t t mode for this. 2c - (2 points) Encode data struct PPM *encode(const char *text, const struct PPM *img); To return a copy of PPM image img with message text hidden in its red pixel values. You will need to make it replace successive random red pixels with the characters from the message. How you select pixels is up to you, but make sure that the pixels are chosen in a consistent order (e.g. left to right, top to bottom) and enough pixels are selected to encode all of the message. 2d - (2 points) Decode data char *decode(const struct PPM *oldimg, const struct PPM *newimg); to return a new string containing the message hidden in the red pixel values of PPM image newimg, by comparing it with the red pixel values of PPM image oldimg. The length of the string that it allocates and returns will depend on the length of the message. You may impose a limit on the maximum length of a decoded message if this makes the implementation easier.
=====================================================================================
#include
#include
#include
#include
/* The RGB values of a pixel. */
struct Pixel {
int red;
int green;
int blue;
};
/* An image loaded from a PPM file. */
struct PPM {
int width;
int height;
int max;
struct Pixel *pixels;
};
/* Reads an image from an open PPM file.
* Returns a new struct PPM, or NULL if the image cannot be read. */
struct PPM *getPPM(FILE * f)
{
int width, height, max;
if (fscanf(f, "P3 %d %d %d ", &width, &height, &max) != 3) {
fprintf(stderr, "Error reading PPM header ");
return NULL;
}
}
/* Write img to stdout in PPM format. */
void showPPM(const struct PPM *img)
{
/* TODO: Question 2b */
}
/* Opens and reads a PPM file, returning a pointer to a new struct PPM.
* On error, prints an error message and returns NULL. */
struct PPM *readPPM(const char *filename)
{
/* Open the file for reading */
FILE *f = fopen(filename, "r");
if (f == NULL) {
fprintf(stderr, "File %s could not be opened. ", filename);
return NULL;
}
/* Load the image using getPPM */
struct PPM *img = getPPM(f);
/* Close the file */
fclose(f);
if (img == NULL) {
fprintf(stderr, "File %s could not be read. ", filename);
return NULL;
}
return img;
}
/* Encode the string text into the red channel of image img.
* Returns a new struct PPM, or NULL on error. */
struct PPM *encode(const char *text, const struct PPM *img)
{
/* TODO: Question 2c */
return NULL;
}
/* Extract the string encoded in the red channel of newimg, by comparing it
* with oldimg. The two images must have the same size.
* Returns a new C string, or NULL on error. */
char *decode(const struct PPM *oldimg, const struct PPM *newimg)
{
/* TODO: Question 2d */
return NULL;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
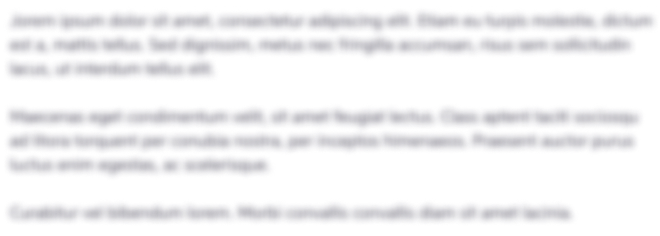
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started