Question
The producer-consumer problem is a classic example of multi-thread/ multiprocess synchronization problem. In this code a producer produces a letter and puts it in a
The producer-consumer problem is a classic example of multi-thread/ multiprocess synchronization problem. In this code a producer produces a letter and puts it in a buffer and a consumer retrieves a letter and prints it. Compile and run the program as given below and EXPLAIN the output generated by the program
//---------------------------
#include
#include
#define BUFF_SIZE 20
char buffer[BUFF_SIZE];
int nextIn = 0;
int nextOut = 0;
void Put(char item)
{
buffer[nextIn] = item;
nextIn = (nextIn + 1) % BUFF_SIZE;
printf("Producing %c ... ", item);
}
void * Producer()
{
int i;
for(i = 0; i < 15; i++)
{
sleep(rand()%9);
Put((char)('A'+ i % 26));
}
}
void Get(char item)
{
item = buffer[nextOut];
nextOut = (nextOut + 1) % BUFF_SIZE;
printf("Consuming %c ... ", item);
}
void * Consumer()
{
int i;
char item;
for(i = 0; i < 15; i++)
{
sleep(rand()%4);
Get(item);
}
}
void main()
{
pthread_t pid, cid;
pthread_create(&pid, NULL, Producer, NULL);
pthread_create(&cid, NULL, Consumer, NULL);
pthread_join(pid, NULL);
pthread_join(cid, NULL);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
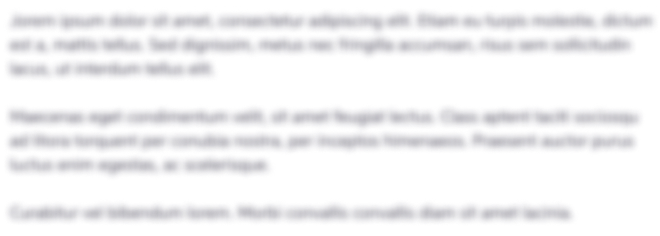
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started