Question
The program below displays the first 50 prime numbers in five lines, each containing ten numbers. Modularize it . public class PrimeNumber { public static
The program below displays the first 50 prime numbers in five lines, each containing ten numbers. Modularize it.
public class PrimeNumber {
public static void main(String[] args) {
final int NUMBER_OF_PRIMES = 50; // Number of primes to display
final int NUMBER_OF_PRIMES_PER_LINE = 10; // Display 10 per line
int count = 0; // Count the number of prime numbers
int number = 2; // A number to be tested for primeness
System.out.println("The first 50 prime numbers are ");
// Repeatedly find prime numbers
while (count < NUMBER_OF_PRIMES) {
// Assume the number is prime
boolean isPrime = true; // Is the current number prime?
// Test whether number is prime
for (int divisor = 2; divisor <= number / 2; divisor++) {
if (number % divisor == 0) { // If true, number is not prime
isPrime = false; // Set isPrime to false
break; // Exit the for loop
}
}
// Display the prime number and increase the count
if (isPrime) {
count++; // Increase the count
if (count % NUMBER_OF_PRIMES_PER_LINE == 0) {
// Display the number and advance to the new line
System.out.println(number);
}
else System.out.print(number + " ");
}
// Check if the next number is prime
number++;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
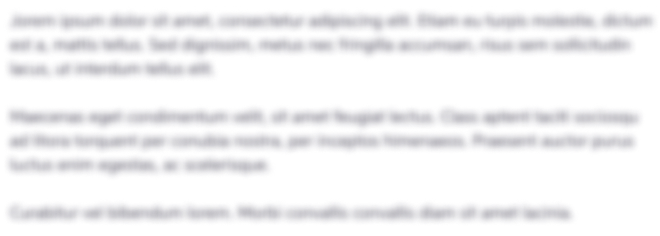
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started