Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The program does not give expected results public class DebugSolutions { // TODO #4 // Calculate Sum Of Numbers In String // The following function
The program does not give expected results public class DebugSolutions { // TODO #4 // Calculate Sum Of Numbers In String // The following function returns a positive integer representing the sum of numbers in the inputString. // Assumes the inputString is not empty. // The following code compiles successfully but fails to return the desired result. // Your task is to fix the code so that it passes all test cases. public int calculateSumOfNumbersInString(String inputString) { String temp = ""; int sum = 0; for (int i = 0; i < inputString.length(); i++) { char ch = inputString.charAt(i); if (Character.isDigit(ch)) temp += ch; else sum += Integer.parseInt(temp); temp = "0"; } return sum + Integer.parseInt(temp); } private boolean isVowel(char ch) { return (ch == 'a') || (ch == 'e') || (ch == 'i') || (ch == 'o') || (ch == 'u'); } // TODO #5 // The following code compiles successfully but fails to return the desired result. // Your task is to fix the code so that it passes all test cases. public String removeConsecutiveVowels(String str) { String str1 = ""; str1 = str1 + str.charAt(0); for (int i = 1; i < str.length(); i++) if ((!isVowel(str.charAt(i - 1))) && (!isVowel(str.charAt(i)))) { char ch = str.charAt(i); str1 = str1 + ch; } return str1; } // TODO #6 // Reverse Alphabet Chars Only // The following function returns a string representing the reversed string in such a way that the position // of the special chars are not affected. // The following code compiles successfully but fails to return the desired result. // Your task is to fix the code so that it passes all test cases. public String reverseAlphabetCharsOnly(String inputString) { char[] inputChar = inputString.toCharArray(); int right = inputString.length() - 1; int left = 0; while (left < right) { if (!Character.isAlphabetic(inputChar[left])) left++; else if (!Character.isAlphabetic(inputChar[right])) right--; else { char temp = inputChar[left]; inputChar[left] = inputChar[right]; inputChar[right] = temp; } left++; right--; } return new String(inputChar); } // TODO #7 // Compare Product // The following function computes two products of digits included in the passed num. // prodValues1 contains a product of every other digit in the num starting with the last digit. // prodValues2 contains a product of every other digit in the num starting with the second last digit. // The method returns true if the products are equal. // The following code compiles successfully but fails to return the desired result. // Your task is to fix the code so that it passes all test cases. public boolean compareProduct(int num) { boolean result = false; boolean toggle = true; if (num > 10) { int prodValues1 = 1, prodValues2 = 1; while (num > 0) { int digit = num / 10; if (toggle) prodValues1 *= digit; else prodValues2 *= digit; num = num / 10; toggle = !toggle; } System.out.println("prodValues1 = " + prodValues1); System.out.println("prodValues2 = " + prodValues2); if (prodValues1 == prodValues2) result = true; } return result; } public static void main(String[] args) { DebugSolutions debug = new DebugSolutions(); // TODO #2 // See the testcases: System.out.println(" \u001B[34m\u001B[1m*** Debugging calculateSumOfNumbersInString method ***\u001B[0m "); String[] inputs1 = {"123", "100 200 300", "1 2 3 4 56 78 9", "100000 20000 3000 400 50 6", "0"}; for (int i = 0; i < inputs1.length; i++) { System.out.print("Sum of numbers in the string \"" + inputs1[i] + "\" is : "); System.out.println(debug.calculateSumOfNumbersInString(inputs1[i])); } System.out.println(" \u001B[34m\u001B[1m*** Debugging removeConsecutiveVowels method ***\u001B[0m "); String[] inputs2 = {"abc", "sweet", "Ceasious", "Euouae", "queueing"}; for (int i = 0; i < inputs2.length; i++) { System.out.print("The input string \"" + inputs2[i] + "\" after consecutive vowels have been removed is: \""); System.out.println(debug.removeConsecutiveVowels(inputs2[i]) + "\""); } System.out.println(" \u001B[34m\u001B[1m*** Debugging reverseAlphabetCharsOnly method ***\u001B[0m "); String[] inputs3 = {"abc+--z", "s.w?e-e!t", "123a?", "@csuci.edu", "abc", "ab&cd"}; for (int i = 0; i < inputs3.length; i++) { System.out.print("The input string \"" + inputs3[i] + "\" after alphabetical chars have been swapped is: \""); System.out.println(debug.reverseAlphabetCharsOnly(inputs3[i]) + "\""); } System.out.println(" \u001B[34m\u001B[1m*** Debugging compareProduct method ***\u001B[0m "); int[] inputs4 = {99, 263, 123456, 3126, 3443, 100, 2}; for (int i = 0; i < inputs4.length; i++) { System.out.println("The input number is " + inputs4[i]); System.out.println("Are the products the same? --> " + debug.compareProduct(inputs4[i]) + " "); } } } // TODO #3 // See the EXPECTED RESULTS below: /* EXPECTED RESULTS: *** Debugging calculateSumOfNumbersInString method *** Sum of numbers in the string "123" is : 123 Sum of numbers in the string "100 200 300" is : 600 Sum of numbers in the string "1 2 3 4 56 78 9" is : 153 Sum of numbers in the string "100000 20000 3000 400 50 6" is : 123456 Sum of numbers in the string "0" is : 0 *** Debugging removeConsecutiveVowels method *** The input string "abc" after consecutive vowels have been removed is: "abc" The input string "sweet" after consecutive vowels have been removed is: "swet" The input string "Ceasious" after consecutive vowels have been removed is: "Cesis" The input string "Euouae" after consecutive vowels have been removed is: "E" The input string "queueing" after consecutive vowels have been removed is: "qung" *** Debugging reverseAlphabetCharsOnly method *** The input string "abc+--z" after alphabetical chars have been swapped is: "zcb+--a" The input string "s.w?e-e!t" after alphabetical chars have been swapped is: "t.e?e-w!s" The input string "123a?" after alphabetical chars have been swapped is: "123a?" The input string "@csuci.edu" after alphabetical chars have been swapped is: "@udeic.usc" The input string "abc" after alphabetical chars have been swapped is: "cba" The input string "ab&cd" after alphabetical chars have been swapped is: "dc&ba" *** Debugging compareProduct method *** The input number is 99 prodValues1 = 9 prodValues2 = 9 Are the products the same? --> true The input number is 263 prodValues1 = 6 prodValues2 = 6 Are the products the same? --> true The input number is 123456 prodValues1 = 48 prodValues2 = 15 Are the products the same? --> false The input number is 3126 prodValues1 = 6 prodValues2 = 6 Are the products the same? --> true The input number is 3443 prodValues1 = 12 prodValues2 = 12 Are the products the same? --> true The input number is 100 prodValues1 = 0 prodValues2 = 0 Are the products the same? --> true The input number is 2 Are the products the same? --> false Process finished with exit code 0 */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
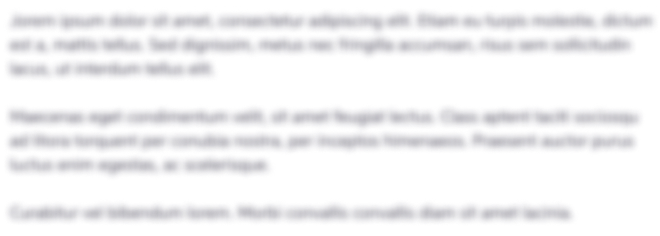
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started