Question
The Program in this lab converts some typed text into Morse code; for example SOS would be converted to --- and it will also sound-out
The Program in this lab converts some typed text into Morse code; for example SOS would be converted to --- and it will also sound-out the dots and dashes. Problem: it only works with some text; you must add the additional functionality so that the program converts any text into correct Morse code, complete with sound.
1) Complete the code by: a. Fill in the comment heading information b. Use the Internet to find the adding the missing Morse Code; c. Identify the programming pattern and include the missing Morse code.
2) Currently the program will convert a single line of keyboard text into Morse code, modify the program such that it will accept a file as input.
3) Research the creation of Morse code and summarize: a. Motivation for its development; b. Was it easy to find investors? give a reason; c. Technical problems that had to be overcome; d. Impact on the world.
#include #include #include using namespace std; //function prototype void morseCode(char); // Simply use the Windows API, Sleep to cause a delay. void delay(int wait) { Sleep( wait); } void dot() { Beep(400, 100); //Beep( freq, duration) cout << '.'; }
void dash() { Beep(500, 200); cout << '-'; delay(1); // Sleep(1000); } // main is the function used by a C/C++ compiler to always start a program. int main () { SetConsoleTitle( "Text to Morse Code" ); string in; cout<< "enter a word: "; getline(cin, in, ' '); // SOME CODE TO HELP YOU WITH FILE ACCESS // // string filename; // cout << "enter filename: "; // cin >> filename; // // fstream infile; // // ---> YOU MUST convert a C++ string into standard C string // // infile.open( filename.str() ); // // if ( !infile.is_open() ) // { // cout << " missing program" << endl; // exit(0); // } // // --> Redirect getline so it uses infile and NOT cin. // getline(infile, in, ' '); string opcode; int value; // cout << "length is :" << in.length(); int l = in.length();
for(int i=0; i<< "letter "<< letter; morseCode( letter); cout << endl; delay(1); } return 0; } // end of main void morseCode(char letter) { switch(toupper(letter)) { case 'O': dash(); dash(); dash(); break; case 'S': dot();dot();dot(); break; case ' ': // blank space, need a pause without sound. break; default: cout<<"bad input"<< endl; } } // end of morse code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
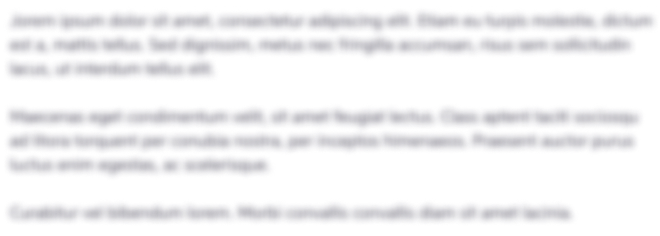
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started