Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The program must use these 2 threads to communicate with each other using a Producer - Consumer approach: The Producer loop: while ( 1 )
The program must use these threads to communicate with each other using a ProducerConsumer approach:
The Producer loop:
while
pthreadmutexlock&mutex; Lock the mutex before checking if the buffer has data
while count SIZE Buffer is full; Wait for signal that space is available
pthreadcondwait&spaceavailable, &mutex;
putitemvalue; Space is free, add an item! This will increment int count
pthreadmutexunlock&mutex; Unlock the mutex
pthreadcondsignal&hasdata; Signal consumer that the buffer is no longer empty
The Consumer loop:
while
pthreadmutexlock&mutex; Lock the mutex before checking if the buffer has data
while count Buffer is empty. Wait for signal that the buffer has data
pthreadcondwait&hasdata, &mutex;
value getitem; There's an item, get it This will decrement int count
pthreadmutexunlock&mutex; Unlock the mutex
pthreadcondsignal&spaceavailable; Signal that the buffer has space
Both threads must share one mutex and two condition variables to control and protect the counting of a number. The number must count from a starting value of to by ones, at which point the program will end. You get to decide which parts of the incrementation and printing go in each thread.
Your variables must be named as follows:
Your mutex must be named "myMutex".
Your two conditions variables must be named "myCond and "myCond
Your counting variable must be named "myCount".
Your program must output lines that contain the following text exactly as written, at the following times. No other lines are allowed to be in the output:
When your program begins:
PROGRAM START
When thread is created:
CONSUMER THREAD CREATED
When myCount changes value:
myCount:
Example:
myCount:
When myMutex is unlocked:
: myMutex unlocked
Example:
CONSUMER: myMutex unlocked
When myMutex is locked:
: myMutex locked
Example:
CONSUMER: myMutex locked
When myCond or myCond has pthreadcondwait called on it:
: waiting on
Example:
PRODUCER: waiting on myCond
When myCond or myCond has pthreadcondsignal called on it:
: signaling
Example:
CONSUMER: signaling myCond
When your program ends:
PROGRAM END Example Code: #include
#include
#include
Declaration of thread condition variables
pthreadcondt myCond PTHREADCONDINITIALIZER;
pthreadcondt myCond PTHREADCONDINITIALIZER;
Declaration of mutex
pthreadmutext myMutex PTHREADMUTEXINITIALIZER;
Shared variable
int myCount ;
Thread function for the producer
void producervoid arg
while
Lock mutex
pthreadmutexlock&myMutex;
printfPRODUCER: myMutex locked
;
Check and wait on condition
whilemyCount
printfPRODUCER: waiting on myCond
;
pthreadcondwait&myCond &myMutex;
Critical section
printfmyCount: d d
myCount, myCount;
myCount;
Signal consumer thread and unlock mutex
pthreadcondsignal&myCond;
printfPRODUCER: signaling myCond
;
printfPRODUCER: myMutex unlocked
;
pthreadmutexunlock&myMutex;
Break if count reached
ifmyCount break;
return NULL;
Thread function for the consumer
void consumervoid arg
printfCONSUMER THREAD CREATED
;
while
Lock mutex
pthreadmutexlock&myMutex;
printfCONSUMER: myMutex locked
;
Check and wait on condition
whilemyCount
printfCONSUMER: waiting on myCond
;
pthreadcondwait&myCond &myMutex;
Critical section
printfmyCount: d d
myCount, myCount;
myCount;
Signal producer thread and unlock mutex
pthreadcondsignal&myCond;
printfCONSUMER: signaling myCond
;
printfCONSUMER: myMutex unlocked
;
pthreadmutexunlock&myMutex;
Break if count reached again
ifmyCount break;
return NULL;
int main
pthreadt prod, cons;
printfPROGRAM START
;
Create producer thread
ifpthreadcreate&prod, NULL, &producer, NULL
perrorFailed to create producer thread";
return ;
Create consumer thread
ifpthreadcreate&cons, NULL, &consumer, NULL
perrorFailed to create consumer thread";
return ;
Wait for threads to finish
pthreadjoinprod NULL;
pthreadjoincons NULL;
printfPROGRAM END
;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
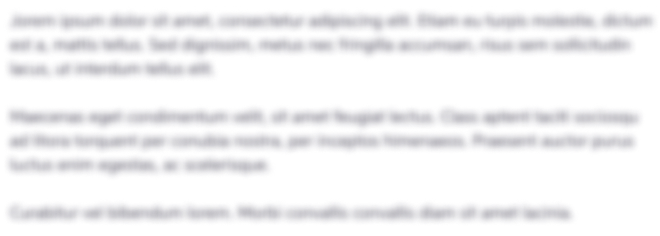
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started