Question
The program should compute the area for each shape instance then write dimension(s) and the area to the corresponding output file. You will create an
The program should compute the area for each shape instance then write dimension(s) and the area to the corresponding output file. You will create an output file for each shape type. All circle data should be written to a file called circles.txt, square data to squares.txt, rectangle data to rectangles.txt, and triangle data to triangles.txt.
Use the given Shape, Square, Circle, Rectangle, and Triangle classes. Areas.java contains a skeleton of the program. Do the following:
1. Set up a Scanner object scan from the input file and PrintWriter objects circles, squares, rectangles, and triangles to the corresponding output files inside the try clause (see the comments in the program
2. Inside the while loop add code to read the input fileget the shape and based on the name, get the corresponding number of dimension values. Instantiate the corresponding shapeObj then compute the area. Write the dimension(s) and the area to the correct output file.
3. After the loop close the Scanner and PrintWriter objects in a finally block.
4. Think about the exceptions that could be thrown by this program:
A FileNotFoundException if the input file does not exist
An InputMismatchException if it cant read an int or double when it tries to this indicates an error in the input file format
- A NegativeNumberException if a negative input value is entered.
Add a catch for each of these situations, and in each case give as specific a message as you can. The program will terminate if any of these exceptions is thrown, but at least you can supply the user with useful information.
5. Test the program. Test data is in the file shapes.txt. Be sure to test each of the exceptions as well.
6. Modify your program so that it prompts the user for the input filename. Test your program again.
LAB FILES THAT WERE PROVIDED:
// **************************************************************************** // Areas.java // // Reads shape data from a text file and writes data to corresponding text files. // ****************************************************************************
import java.util.*; import java.io.*;
public class Areas { // -------------------------------------------------------------------- // Reads shape data from a text file, computes the area, // then writes data to another file based on the shape type // -------------------------------------------------------------------- public static void main (String[] args) {
String shapeType; // type of shape double area; // area of the shape double radius; // circle dimension double side; // square dimension double length, width; // rectangle dimensions double base, height; // triangle dimensions
String name, inputName = "shapes.txt"; String circleName = "circles.txt"; String squareName = "squares.txt"; String rectangleName = "rectangles.txt"; String triangleName = "triangles.txt"; Shape shapeObj = null;
try { // Set up Scanner to input file
// Set up the output file streams
// Print a header to each of the output files squares.println (); squares.println ("Squares"); squares.println ();
circles.println (); circles.println ("Circles"); circles.println (); rectangles.println (); rectangles.println ("Rectangles"); rectangles.println (); triangles.println (); triangles.println ("Triangles"); triangles.println (); // Process the input file, one token at a time while (scan.hasNext()) { // Get the shape name and dimension(s) // Instantiate the correct object for shapeObj // Calculate the corresponding area using the correct area() method // Write the shape dimension(s) and area in the correct file } } //Add a catch for each of the specified exceptions, and in each case //give as specific a message as you can //Close all files in a finally block } }
public abstract class Shape { private String shapeName; public Shape(String name) { shapeName = name; } public abstract double area(); public String getName() { return shapeName; } public String toString() { return "Shape: " + shapeName; } }
public class Circle extends Shape{ private double radius; public Circle (String name, double r) { super(name); radius = r; } public double area() { return Math.PI * radius * radius; } public String toString() { return super.toString() + "Radius = " + radius; } public boolean equals(Object otherObj) { if (otherObj == null) { return false; } else if (otherObj.getClass() != this.getClass()) return false; else { Circle otherCirc = (Circle) otherObj; if (otherCirc.getName().equals(this.getName()) && Math.abs(otherCirc.radius - this.radius) < 0.0001 ) return true; else return false; }
public class Rectangle extends Shape{ private double length, width; public Rectangle (String name, double l, double w) { super(name); length = l; width = w; } public double area() { return length * width; } public String toString() { return super.toString() + " Length = " + length + " Width = " + width; } public boolean equals(Object otherObj) { if (otherObj == null) { return false; } else if (otherObj.getClass() != this.getClass()) return false; else { Rectangle otherRect = (Rectangle) otherObj; if (otherRect.getName().equals(this.getName()) && Math.abs(otherRect.length - this.length) < 0.0001 && Math.abs(otherRect.width - otherRect.width) < 0.0001) return true; else return false; } } }
public class Square extends Shape{ private double side; public Square (String name, double s) { super(name); side = s; } public double area() { return side * side; } public String toString() { return super.toString() + "Side = " + side; } public boolean equals(Object otherObj) { if (otherObj == null) { return false; } else if (otherObj.getClass() != this.getClass()) return false; else { Square otherSq = (Square) otherObj; if (otherSq.getName().equals(this.getName()) && Math.abs(otherSq.side - this.side) < 0.0001 ) return true; else return false; } } }
public class Triangle extends Shape{ private double base, height; public Triangle (String name, double b, double h) { super(name); base = b; height = h; } public double area() { return 0.5 * base * height; } public String toString() { return super.toString() + "Base = " + base + "Height = " + height; } public boolean equals(Object otherObj) { if (otherObj == null) { return false; } else if (otherObj.getClass() != this.getClass()) return false; else { Triangle otherTri = (Triangle) otherObj; if (otherTri.getName().equals(this.getName()) && Math.abs(otherTri.height - this.height) < 0.0001 && Math.abs(otherTri.base - otherTri.base) < 0.0001) return true; else return false; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
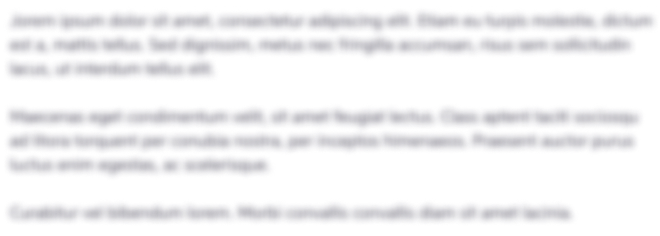
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started