Question
The programming language is Java. Post only the complete code please. All classes should be serializable and comparable. The driver is also required for this.
The programming language is Java. Post only the complete code please. All classes should be serializable and comparable. The driver is also required for this.
Implementing GUI and sorting methods to this is optional, but greatly appreciated.
You are not required to use GUI for this project. Your output can be on the screen . However at the end of each run you must store all your lists in an array and then into a file.
Problem: you are to create an app for CSUS library. A Library has different members such as students. Members should be able to search for a book by different criteria such as title, author, and subject. As soon as the book is found in the library, then the student will check out the book and this book will be added to the list of the books that the student has borrowed. When a student returns a book, then that book must be remove from his/her list. The information about items and students must be read from files.
Your program must have the input validation such that no user can enter wrong data. Use exception handling whenever possible. In the client class you must use methods. Everything needs to be done through a method call. You are free to add any functionality to your program and any creativity will be appreciated. Your program must read the data from a file. You need three different files for books, students and administrators.
.
Steps to complete your project: 1. There are 6 different classes and you need to implement all these classes and make sure that they work properly by writing client class. You must read the information for the students and books from files to populate the ArrayList for the class students and books.
2. In the main method you must provide the following functionalities Students should be able to do :
o Search by author //must write a method o Search by subject//must write a method o Search by topic//must write a method o Search by keyword//must write a method o After the item is selected, the item must be added to the student list of
checked out items if the item is available.
Admin people should be able to do the following.
o Search for items o Addanewitem o Delete a new item o Add a student
o Remove a student o Search for a student
When implementing the client class, you must use many different methods. Everything must be done through a method call. For example for searching a student in the array you must write a method. Codes without any methods will receive no credit.
Before logging out the application all the updated data must be stored in a file. When you run your program all the data must be loaded from the file back to the lists that you are suppose to create for your program.
Description: In this project you are going to develop a library application. Using this application students will be able to search for books, video CD, journals, and audio CD. Administrators will use this app to add or remove resources from the database in the library. Therefore the main page should have to different options
o Students (id number is required). If student is not found in the list of the students then n error message should be displayed
o Administrators (id number is required). If the id is not found an error message must be displayed
Students Students should be able to search the data base using different search criteria such as:
Title Subject
Keywords in the subject
Keywords in the title
Once the search criteria is declared by the user, then the list of all the available resources must appear on the screen. Once the user selects the desired item, then the status for the item must be changed to check out and the item must be added to the student account. A return date must be specified for the user. When a book is returned by a student, first your app must make sure that the book has been returned on time otherwise a penalty will be added to the user account. If the total penalty is more than 50 dollars on a student account, then a hold will be put on the user account and the student will no longer be able to check out any books.
All the information for the students and resources must be stored in a file therefore every time you log out the system all the info must be saved to a file. As soon as you log in all the info must be read from files and stored in appropriate collections. You must make all your classes serializable
Administrators: Administrators should be able
To add or remove items to/from the database
Add or remove students
Put a hold on a student account
Search through the students and send a notification to the students who have pass
due item
To implement this application we need the following classes 1. Item (holds information about a book, cd or journal)
Item |
-String title -String subject -String author -String publisher -String yearPublished// must be validated -boolean status -String type// validate type it can only be book, journal audio CD , Video CD |
+Item(String t, string s, string a, string p, string y, boolean stat, string typ) +Item() +all the accessors +all the mutator +toString(): String +equals(Item i): Boolean +contains(String s)// returns true if the string s is found in the title +compareTo(Item i): int //compare based on the title and author -Validatedate(string d): boolean |
2. Person (holds info such as name, last name, ID, email, phone number) |
Person |
- String name - String lastName - String id //must validate the format - String email //must be validate the format - String phone//must validate the format |
+ Person(String n, string l, string initialed, String em, string p) +person() +accessors, mutators +toString(): String +equals(Person p): Boolean //based on the ID number +compaerTo(Persom p): int -validateId(String id): boolean -validateemail(String email) : boolean -validate(String phone) : boolean //based on the last name |
3. Library (holds a collection of items) |
Library |
-ArrayList items |
+Library() +getSize(): int +add(Item i): Boolean //returns false if the item already exists, need to use equals method from the item class |
+remove(item i): boolean //returns false if the item is not in the list, need to use the equals method from the item class +searchTitle(String title): item //search the array based on the title, returns null if the object not found, need to use the equals method from the item class +searachSubject(String subject): item //search the item based on the subject, return null if the object is not found, need to use the equals method from the item class +searchkeyword(String key): Item // returns the item based on the keyword, the keyword could be in the subject or title of the item. Need to use the contains method from the item class |
4. Student |
Student |
-person -ArrayList checkedout |
+student(Person p) +accessors, mutators +equals(Students s) // use the equals method from the person class +addcheckedOut(Item i)// adds the checked out item to the list +toString(): String |
5. Students |
Students |
-ArrayList |
+Students() +add(Student s) +remove(Student) +serachStudent(String id): Boolean +toString(): String |
6. Administrator |
Administrator |
-ArrayList |
+Administrator() +add(Person p) +remove(Person p) +search(Person P) +toString(): String |
Here is a list of the subjects that you can use
Accounting and Finance
Anthropology
Area Studies
Art
Basic Search
Biological Sciences
Business and Management
Chemistry
Child Development
Communication Studies
Computer Science
Construction Management
Criminal Justice
Design
Economics
Education
Engineering
English Language and Literature
Environmental Studies
Ethnic Studies
Family and Consumer Science
Film Studies
Foreign Languages and Literature
Geography and Maps
Geology and Earth Sciences
Gerontology
Government and Political Science
Health and Medical Sciences
Hellenic Studies
History
Humanities
International Affairs
International Business
Journalism
Kinesiology
Law
Library and Information Technology
Management Information Science
Marketing
Mathematics
Music
News and Reference
Nursing
Philosophy
Physical Therapy
Physics and Astronomy Psychology Public Policy and Administration
The following is flawed/imcomplete code. Feel free to change it when neccessary.
public class Item { private String title; private String subject; private String author; private String publisher; private String yearPublished; private String type; private boolean status; public Item() { title = ""; subject = ""; author = ""; publisher = ""; yearPublished = ""; type = ""; status = false; } public Item(String t, String s, String a, String p, String y, boolean stat, String typ) { title = t; subject = s; author = a; publisher = p; yearPublished = y; type = typ; status = stat; } public String getTitle() { return title; } public String getSubject() { return subject; } public String getAuthor() { return author; } public String getPublisher() { return publisher; } public String getYear() { return yearPublished; } public boolean getStatus() { return status; } public String getType() { return type; } public void setTitle(String t) { title =t; } public void setSubject(String sub) { subject = sub; } public void setAuthor(String a) { author = a; } public void setPublisher(String pub) { publisher = pub; } public void setYear(String year) { yearPublished = year; } public void setStatus(boolean newStat) { status = newStat; } public void setType(String t) { //validate type it can only be book, journal audio CD , Video CD while(!t.equalsIgnoreCase("book") && !t.equalsIgnoreCase("journal audio CD") && !t.equalsIgnoreCase("Video CD")) { if(t.equalsIgnoreCase("book") || t.equalsIgnoreCase("journal audio CD") || t.equalsIgnoreCase("Video CD")) { type = t; } else { System.out.println("Wrong input. Please enter book, journal audio CD, or Video CD"); } } } public String toString() { String s = "Title: " + title + " " + "Subject: " + subject + " " + "Author: " + author + " "; s += "Publisher: " + publisher + " " + "Year Published: " + yearPublished + " " + "Type: " + type + " "; return s; } public boolean equals(Item i) { if(this.title == i.title || this.subject == i.subject) { status = true; } return status; } public boolean contains(String s) { if(title.contains(s) || subject.contains(s)) { return true; } return false; } public int compareTo(Item i) { if(this.title == i.title && this.author == i.author) { return 1; } else if(this.title == i.title || this.author == i.author ){ return 0; } else { return -1; } } public boolean validateDate(String d) { if(this.yearPublished == d) { return true; } return false; } }
import java.io.*; import java.util.*; public class Library extends Item { private ArrayList items; public Library() { items = new ArrayList(); } public int getSize() { return items.size(); } public boolean add(Item i) { boolean duplicate = equals(i); if(duplicate == true) { return false; } else { return true; } } public boolean remove(Item i) { boolean duplicate = equals(i); if(duplicate == false) { return false; } else { return true; } } public Item searchTitle(String t) { //searches for items by string title for(int i = 0; i < items.size(); i++) { if(items.get(i).equals(t)) { //returns items return items.get(i); } } //else return null return null; } public Item searchSubject(String s) { //searches for items by string subject for(int i = 0; i < items.size(); i++) { if(items.get(i).equals(s)) { //returns items return items.get(i); } } //else return null return null; } public Item searchKeyword(String k) { //searches for items by string keyword for(int i = 0; i < items.size(); i++) { if(items.get(i).contains(k)) { //returns items return items.get(i); } } //else return null return null; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
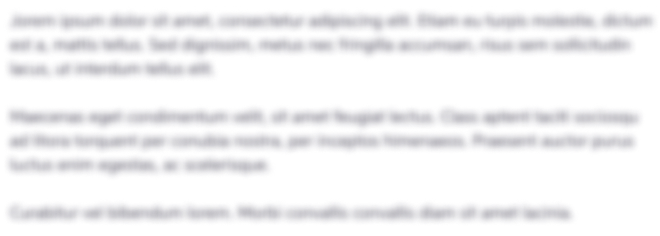
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started