Question
The programming Languange is Java. You must write a robust program meaning that your program should not crash with any given data The shell for
The programming Languange is Java.
You must write a robust program meaning that your program should not crash with any given data
The shell for all the programs that you need to write has been provided
Write a program that
1. Gets an infix expression form the user and evaluate the expression using stack ADT
Finds the postfix equivalent of the given infix expression
Evaluate the created postfix expression.
Note: your program should not crash when you enter an invalid expression such as: 2 + 4 * (6 -3
You need to create the Stack interface and implements the interface using ArrayList.
Also you need to have a tester class. In the tester class you must have the following methods. Programs that are not implemented using the following methods will get no credit.
You must implement the following methods in the tester/driver class
1. infixToPostfix(String s): This method receives an infix expression and then creates its postfix and return it.
a. go to the website http://docs.oracle.com/javase/6/docs/api/java/util/StringTokenizer.html and read about the class called StringTokenizer. You need to use this class to tokenize the expression that you receive. In the expression 2 + 3, the tokens are 2, 3, +. If there are any spaces in the expression they must be ignored.
b. Here is the algorithm to convert infix to postfix: Initialize the stack with the dummy operator #. Repeat until no more tokens.
Get the next token.
If the next token is an operand, enqueue the operand to the postfix String. Declare a String called postfix, every time you read an operand concatenate that to the postfix string.
If the next token is a (, push it in the stack.
If the next token is a ), pop and enqueue operators to the postfix string until a ( is on the top of the stack. Pop ( out of the stack.
If the next token is an operator, pop and enqueue operators to the postfix until the top of the stack has a lower precedence. Push the current operator into the stack.
If more tokens go to the beginning, If no more tokens, pop and enqueue operators until the dummy oprerator # is on the top of the stack. Pop # out of the stack.
Return the postfix String. Note that # and (has a precedence lower than other operators.
2. Now that you converted the infix to postfix, we can evaluate it using stack. You need to write a method called: postFixEvaluater(String post) Use the following algorithm to evaluate a postfix expression
Repeat until no more tokens Get the next token:
a. If the next token is an operand, push it into the stack. b. If the next token is an operator pop two operands from the stack, do the operation,
and push the result back to the stack. After you are done reading all the tokens and doing the operations, the final result is the only thing on the stack when done. (If theres more than one thing in the stack, there is an error in the expression.)
Here is a sample output:
Enter your infix expression : 3+7*6/3-8*2 postFix = 3 7 6 * 3 / + 8 2 * -
3 7 6 * 3/+82*-=1 Do you have another expression :yes
Enter your infix expression:(7+9)*(6+3*5/3) postFix = 7 9 + 6 3 5 * 3 / + * 7 9 + 6 3 5 * 3 / + * = 176 Do you have another expression :yes
Enter your infix expression:3+8*5-3+25/5 postFix = 3 8 5 * + 3 - 25 5 / + 3 8 5 * + 3 - 25 5 / + = 45 Do you have another expression :no
Shell code:
import java.util.*; import java.util.StringTokenizer;//a class for tokenizing a string public class Expression { public static void main(String[] args) { //your code } public static String postFix(String s) { //your code return ""; } public static int postfixEvaluate(String s) { //your code return 0; } }
//this a generic stack and the type can be replaced at the compile time, errors are be detected at compile time rather than //run time, //implement the stack class using ArrayList import java.util.ArrayList; public class GenericStack implements Stack { //instance variables public int size() { return 0; } public Object peek() { return 0; } public Object pop() { return null; } public void push(Object o) { } public boolean isEmpty() { return false; } }
public interface Stack { public int size(); public boolean isEmpty(); public Object pop(); public void push (Object element); public Object peek(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
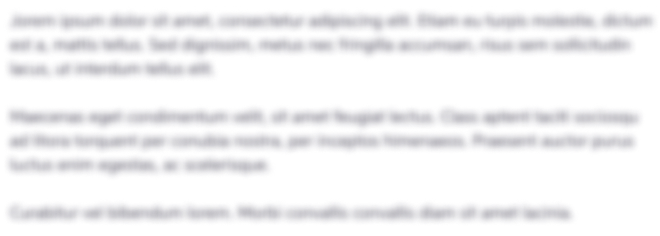
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started