Question
The promgram must be implemented in C language. Description Write a simple calculator program that allows the user to enter numbers or operators to complete
The promgram must be implemented in C language.
Description
Write a simple calculator program that allows the user to enter numbers or operators to complete a calculation.
Base Specification
Your program will implement a loop that continually waits for the user to either enter a floating point number (a double) or an operator (a character). When a number is entered, your program remembers it. When an operator is entered, your program performs that operation on the last two inputs. For example, here is a sequence of inputs and outputs for your program:
3
33
-
= 30.000
Note that the minus sign subtracted the 3 from 33. This brings us to the first rule of the spec:
Rule 1: The earlier input (called EARLIER) is subtracted from the later input (called LATER). All the operators will work this way. The output should be printed with a preceding = using the following format string: =%.3lf.
Here is another example:
3
33
+
= 36.000
+
= 39.000
In this example, the plus sign is entered and addition is performed. When we enter + again, we get the result 39. How did that happen? Well, if you look through the numbers, you might guess that 33 was added to 3. This is because the calculator will only store two numbers at a time. Originally, the programmer entered 3 and 33. So, initially EARLIER=3 and LATER=33. But, after the addition, we change LATER=36, the result of the addition. Then, when we enter + again, we add LATER+EARLIER and print 39.000. And, you might have guessed it, we set LATER=39. So, if we entered + again, we would print 42.000, and LATER=42. This brings us to the second rule of the spec:
Rule 2: The result of any operation is retained in LATER. By entering an operator (OP) multiple times, you are performing LATER = LATER OP EARLIER repeatedly.
Rule 3: Your program will support the following operators with the following meaning:
Commands (character) | Meaning | Example Input/Output |
x | LATER= LATER * EARLIER | 1 2 x =2.000 |
d | LATER=LATER / EARLIER If EARLIER is 0, you must print Divide by zero error. and abort the operation. EARLIER and LATER retain the same values they had before the command was attempted. | 1 2 d =2.000 |
a | LATER=LATER+EARLIER | 1 2 a =3.000 |
s | LATER=LATER-EARLIER | 1 2 s =1.000 |
^ | LATER=LATEREARLIER | 1 2 ^ =2.000 |
w | Swap LATER and EARLIER: tmp=LATER LATER=EARLIER EARLIER=tmp | 1 2 w p 2.000 1.000 |
c | Clear LATER and EARLIER: LATER=0.0 EARLIER=0.0 | 5 c p 0.000 0.000 |
p | Print the values of EARLIER and LATER using this format string: %.3lf | 5 p 0.000 5.000 |
h | Print a help message that describes each command succinctly. | Omitted |
q | quit; print the numbers one last time. | 1 2 q 1.000 2.000 |
Anything else | Error; print a message saying that its not supported using this format string The operator %c is not supported. | 0 0 t The operator t is not supported. |
Rule 4: If more than two numbers are entered in a row, the last two numbers entered are retained as EARLIER and LATER.
For example:
33
4
5
x
= 20.000
Rule 5: You may assume that EARLIER and LATER start out as 0. If one number is entered, then EARLIER is 0 and LATER got its value as an input. If two numbers are entered, both EARLIER and LATER must have values from input.
Examples (note, the comments are not part of the input or output):
x // EARLIER=0 and LATER=0 as initial condition
= 0 // Result is LATER=0
1 // Now LATER=1 and EARLIER=0
a
= 1 // LATER=1
2 // LATER=2 and EARLIER=1
a
= 3 // Now LATER=3
Rule 6: When the quit command (q) is entered, the program must print the values for EARLIER and LATER one last time. Like this, with EARLIER on one line followed by LATER on the next line:
*
= 0
q
0.000
0.000
Beware: The numbering of the rules is the order I chose to describe them, NOT the order you should implement them!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
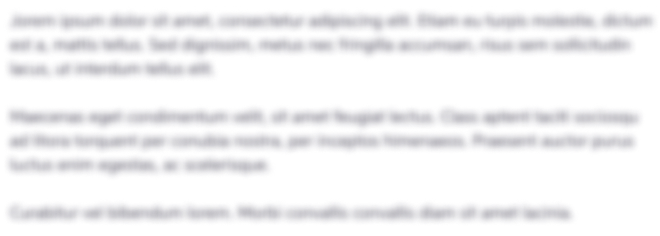
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started