Question
The purpose of this assignment is to practice using pointers. You will learn how and when to use them. Re-write the code for assignment #2,
The purpose of this assignment is to practice using pointers. You will learn how and when to use them.
Re-write the code for assignment #2, to use pointer variables for:
i) parameter passing, where appropriate (think carefully about this. Pointers parameters are used to pass arrays, to change main( ) program variables and to return values from the function to main( ) ). All arrays should be passed using pointers in the receiving function. Remember in the call statement the array name is already an address.
ii) returning values from functions. All called functions should have a void return type. Values being returned by a function should be done through a pointer parameter.
iii) use pointer variables and pointer arithmetic when working with arrays in the functions
HERE is the assignment 2
#include
// function to calculate total sales for one country
int totalCountrySalesInt(int sales[4]);
double totalCountrySalesDouble(double sales[4]);
double totalArtistSales(int usa[5], int canada[5], int uk[5], double australia[5], int artist);
void highestSaleArtist(double artistSales[5], char *artists[5]);
double grandTotal(double totalsales[4]);
int main(void)
{
// Initialize arrays to hold names of countries and artists
char *countries[] = {"USA", "Canada", "UK", "Australia"};
char *artists[] = {"Ed Sheeran", "Drake", "Eminem", "Taylor Swift", "The weekend"};
// Initialize arrays to hold streaming amounts for each artist in each country
int usa[] = {871, 1590, 1190, 993, 1140};
int canada[] = {105, 109, 150, 102, 126};
int uk[] = {291, 140, 229, 98, 157};
double australia[] = {97.7, 43.2, 97.5, 66.5, 71.0};
// make a 2D array to hold all the data
// Initialize arrays to hold artist & country totals as well as the sum of all stream total
double artistTotal[5] = {0};
double countryTotal[4] = {0};
double grandTotals;
// Calculate the total streams for each country and store in countryTotal array
countryTotal[0] = totalCountrySalesInt(usa);
countryTotal[1] = totalCountrySalesInt(canada);
countryTotal[2] = totalCountrySalesInt(uk);
countryTotal[3] = totalCountrySalesDouble(australia);
// Calculate the total streams for each artist and store in artistTotal array
for (int i = 0; i < 5; i++)
{
artistTotal[i] = totalArtistSales(usa, canada, uk, australia, i);
}
// Display the title header for the table where all data are stored
printf("%25s %s %s", "YouTube", "Most Streamed", " Artists of 2021 ");
printf("%27s %s", "by", "Country (millions) ");
// Printing the header row for the table
printf("%-15s %10s %10s %10s %13s %10s ", "Artist", countries[0], countries[1], countries[2], countries[3], "Total");
// Loop through each country and Calculate the total streams for the artist across all artists
for (int i = 0; i < 5; i++)
{
// Print the artist name and their streaming counts for each country
printf("%-15s %10d %8d %13d %10.1f %12.1f ", artists[i], usa[i], canada[i], uk[i], australia[i], artistTotal[i]);
}
// Calculate the total streams for each country and the overall total streams across all countries
grandTotals = grandTotal(countryTotal);
// Print the total streams for each country and the overall total streams across all countries
printf("%-15s %10.f %8.f %13.f %10.1f %12.1f ", "Totals", countryTotal[0], countryTotal[1], countryTotal[2], countryTotal[3],
grandTotals);
// Print the artist with the highest sales
highestSaleArtist(artistTotal, artists);
return 0;
}
// Function to calculate the total sales for one country
int totalCountrySalesInt(int sales[4])
{
int total = 0;
for (int i = 0; i < 5; i++)
{
total += sales[i];
}
return total;
}
// Function to calculate the total sales for one country
double totalCountrySalesDouble(double sales[4])
{
double total = 0;
for (int i = 0; i < 5; i++)
{
total += sales[i];
}
return total;
}
// Function to calculate the total sales for one artist
double totalArtistSales(int usa[5], int canada[5], int uk[5], double australia[5], int artist)
{
double total = 0;
total = usa[artist] + canada[artist] + uk[artist] + australia[artist];
return total;
}
// Function to find the artist with the highest sales
void highestSaleArtist(double artistSales[5], char *artists[5])
{
double highest = 0;
int index = 0;
for (int i = 0; i < 5; i++)
{
if (artistSales[i] > highest)
{
highest = artistSales[i];
index = i;
}
}
printf("The artist with the highest sales is %s with %.1f sales. ", artists[index], highest);
}
// Function to calculate the grand total of all sales
double grandTotal(double totalsales[4])
{
double total = 0;
for (int i = 0; i < 4; i++)
{
total += totalsales[i];
}
return total;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
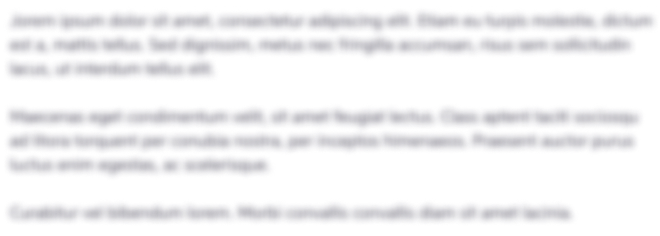
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started