Question
The purpose of this assignment is to practice writing C programs and gain experience working in this low-level, non-object oriented language. After completing both p2A
The purpose of this assignment is to practice writing C programs and gain experience working in this low-level, non-object oriented language. After completing both p2A and p2B, you should be comfortable with pointers, arrays, address arithmetic, structures, command-line arguments, and file I/O in C. For this part, your focus will be on pointers, arrays and address arithmetic.
#include
char *DELIM = ","; // commas ',' are a common delimiter character for data strings
/* COMPLETED (DO NOT EDIT): * Read the first line of input file to get the size of that board. * * PRE-CONDITION #1: file exists * PRE-CONDITION #2: first line of file contains valid non-zero integer value * * fptr: file pointer for the board's input file * size: a pointer to an int to store the size */ void get_board_size(FILE *fptr, int *size) { char *line1 = NULL; size_t len = 0; if ( getline(&line1, &len, fptr) == -1 ) { printf("Error reading the input file. "); exit(1); }
char *size_chars = NULL; size_chars = strtok(line1, DELIM); *size = atoi(size_chars); }
/* TODO: * Returns 1 if and only if the board is in a valid Sudoku board state. * Otherwise returns 0. * * A valid row or column contains only blanks or the digits 1-size, * with no duplicate digits, where size is the value 1 to 9. * * Note: p2A requires only that each row and each column are valid. * * board: heap allocated 2D array of integers * size: number of rows and columns in the board */
int valid_board(int **board, int size) {
return 0; }
/* TODO: COMPLETE THE MAIN FUNCTION * This program prints "valid" (without quotes) if the input file contains * a valid state of a Sudoku puzzle board wrt to rows and columns only. * * argc: CLA count * argv: CLA value */ int main( int argc, char *argv[] ) {
// TODO: Check if number of command-line arguments is correct.
// Open the file and check if it opened successfully. FILE *fp = fopen(*(argv + 1), "r"); if (fp == NULL) { printf("Can't open file for reading. "); exit(1); }
// Declare local variables. int size;
// TODO: Call get_board_size to read first line of file as the board size.
// TODO: Dynamically allocate a 2D array for given board size.
// Read the rest of the file line by line. // Tokenize each line wrt the delimiter character // and store the values in your 2D array. char *line = NULL; sizew_t len = 0; char *token = NULL; for (int i = 0; i < size; i++) {
if (getline(&line, &len, fp) == -1) { printf("Error while reading line %i of the file. ", i+2); exit(1); }
token = strtok(line, DELIM); for (int j = 0; j < size; j++) { // TODO: Complete the line of code below // to initialize your 2D array. /* ADD ARRAY ACCESS CODE HERE */ = atoi(token); token = strtok(NULL, DELIM); } }
// TODO: Call the function valid_board and print the appropriate // output depending on the function's return value.
// TODO: Free all dynamically allocated memory.
//Close the file. if (fclose(fp) != 0) { printf("Error while closing the file. "); exit(1); }
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
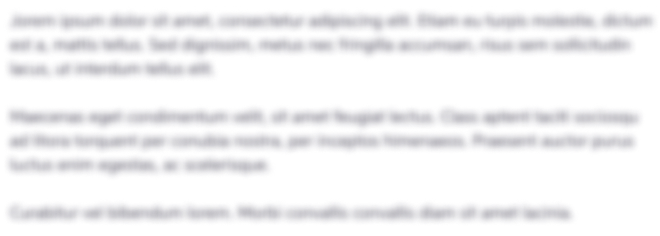
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started