Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The purpose of this C++ programming project is to allow the student to process input and output files. This program has the following four menu
The purpose of this C++ programming project is to allow the student to process input and output files. This program has the following four menu options:
A: Create a new file for Payroll Information B: Enter Payroll Information C: List Payroll Information X: Exit the Payroll Information Module How to Begin Project: 1. Create project P08 2. Add text file output.txt 3. Add text file P08.txt 4. Add C++ source file P08.cpp 5. Copy-and-paste the source code below, and implement options A through C. 6. If the project is built and executed at this point, there will be several warning generated by the compiler, but the program should compile as-is. 7. See the textbook for similar examples. Test Data: Enter the data listed below using option B. Your program will be saving the records in P08.txt as it is entered. 1018 9.50 50 10 25 30 1005 5.00 30 35 40 50 1002 8.00 50 50 10 40 General Processing: See the comments included with the source code provided for hints on what needs to be coded for each function. See the textbook for similar examples. Requirements: Code the createNewFile, enterInfo, and listInfo functions. Turn in: P08.cpp, p08.txt, and sample output (output.txt) from the three options (A, B, and C) Report Layout with Scale: 1 2 3 4 5 6 7 8 12345678901234567890123456789012345678901234567890123456789012345678901234567890 Employee Rate Wk1 Wk2 Wk3 Wk4 1018 9.50 50 10 25 30 1005 5.00 30 35 40 50 1002 8.00 50 50 10 40 Sample Output Option A - Create a new file for Payroll Information: (This option is currently not implemented, but should generate the following.) P08 Main Menu Enter the letter of the desired menu option. Press the Enter key after entering the letter. A: Create a new file for Payroll Information B: Enter Payroll Information C: List Payroll Information X: Exit the Payroll Information Module Choice: a P08 Create new file for Payroll Information Creating a new file will delete any existing information. Do you want to proceed with creating a new file? (Y/N) y New file created successfully! Procedure completed. Press Enter to continue: Sample Output Option B - Enter Payroll Information: (This option is currently not implemented, but should generate the following.) P08 Enter Payroll Information Enter the Employee Id, rate, w1, w2, w3, w4: 1018 9.50 50 10 25 30 Would you like to add another employee? (Y/N) y Enter the Employee Id, rate, w1, w2, w3, w4: 1005 5.00 30 35 40 50 Would you like to add another employee? (Y/N) y Enter the Employee Id, rate, w1, w2, w3, w4: 1002 8.00 50 50 10 40 Would you like to add another employee? (Y/N) n Procedure completed. Press Enter to continue: Sample Output Option C - List Payroll Information: (This option is currently not implemented, but should generate the following.) P08 List Payroll Information Employee Rate Wk1 Wk2 Wk3 Wk4 1018 9.50 50 10 25 30 1005 5.00 30 35 40 50 1002 8.00 50 50 10 40 Procedure completed. Press Enter to continue: Sample Output Option X - Exit: Choice: x Now exiting Payroll Information...please wait. Press any key to continue . . . Source Code: ///P08 File Processing Your Name /* This program allows users to enter and save payroll information to a file. The file can then be read so that the information entered can be displayed. Payroll clerks can choose from the following menu options: A: Create a new file for Payroll Information B: Enter Payroll Information C: Display Payroll Information X: Exit the Payroll Information Module The following items for each employee are saved in the file p08.txt: Employee ID Rate Hours W1,W2,W3,W4 */ #include// file processing #include // cin and cout #include // toupper #include // setw using namespace std; //menu options //Opt A: void createNewFile( ); //Opt B: void enterInfo( ); //Opt C: void listInfo( ); //Supporting functions void displayContinuePrompt( ); //Program starts here void main( ) { //Declare local variable to store menu option selected char choice; //Check to see what the user wants to do do //while (choice != 'X') { cout << "P08 Main Menu "; cout << "Enter the letter of the desired menu option. " << "Press the Enter key after entering the letter. " << " A: Create a new file for Payroll Information " << " B: Enter Payroll Information " << " C: List Payroll Information " << " X: Exit the Payroll Information Module " << "Choice: "; cin >> choice; cout << " "; choice = toupper(choice); //convert to uppercase switch (choice) { case 'A': createNewFile( ); break; case 'B': enterInfo( ); break; case 'C': listInfo( ); break; case 'X': cout << "Now exiting Payroll Information...please wait. "; break; default: cout << "\a"; //alert cout << "Invalid Option Entered - Please try again. "; }//end of switch } while (choice != 'X'); return; }//end of main //Function Definitions void createNewFile( ) { //Declare local variables char answer; cout << "P08 Create new file for Payroll Information "; //Student must remove next line of code and code the rest of the function. cout << "This option has not been implemented yet."; //Prompt the user if they want to create a new file //If Yes, try to create a new file using open function // Open the file for output // If there are any errors, // display an error message and return. // else // display message that a file was created successfully // Close the file //else // display a message that a new file was NOT created //Call displayContinue to pause displayContinuePrompt( ); return; } void enterInfo( ) { //Declare local variables int employeeId; double rate; int w1Hours, w2Hours, w3Hours, w4Hours; char answer; cout << "P08 Enter Payroll Information "; //Student must remove next line of code and code the rest of the function. cout << "This option has not been implemented yet."; //Open the file for output (append) //If there are any errors, // display an error message and return. //Set output to 2 decimal positions for file //Use a do-while loop to get input // Display prompt and get the data // Write data to file and format using setw manipulators // Prompt user if they want to add another employee // Do loop again if they don't enter N //end do-while loop //Close the file //Call displayContinue to pause displayContinuePrompt( ); return; } void listInfo( ) { //Declare local variables int employeeId; double rate; int w1Hours, w2Hours, w3Hours, w4Hours; cout << "P08 List Payroll Information "; //Student must remove next line of code and code the rest of the function. cout << "This option has not been implemented yet. "; //Open the file for input //If there are any errors, // display an error message and return. //Set output to 2 decimal positions for cout cout.setf(ios::fixed); cout.setf(ios::showpoint); cout.precision(2); //Display headings cout << "Employee Rate Wk1 Wk2 Wk3 Wk4 "; //Read the first record. //Use while loop to process file, because // while loops handle empty files. // Display record read and format using setw manipulators // Read next record //end while loop //Close the file //Call displayContinue to pause displayContinuePrompt( ); return; } void displayContinuePrompt( ) { //Declare local variables char prompt; cout << " Procedure completed. Press Enter to continue: "; cin.ignore( ); prompt = cin.get( ); system("cls"); //clear screen - DOS return; } //End of program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
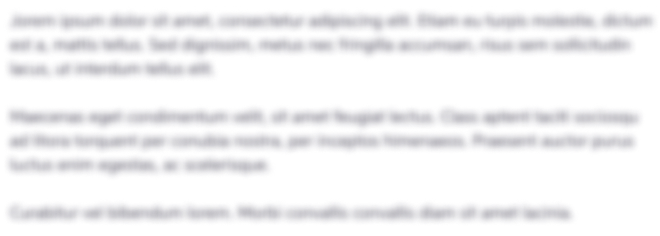
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started