Question
The purpose of this project is to give students exposure to object oriented design and programming using classes and polymorphism in an application that involves
The purpose of this project is to give students exposure to object oriented design and programming using classes and polymorphism in an application that involves arrays of objects and sorting arrays containing objects. You will write several classes for Program 8.
Please submit all classes in one file. The name of the physical program file submitted program8
Assignment: Best Office Supplies Inc, an office supply store, services many customers. As customers orders for office supplies are shipped, information is entered into a file. Best Office Supplies bills their customers once each month. At the end of each month, the Chief Executive Officer requests a report of all customers sorted by their customer id (from lowest to highest). The report includes their bill balance and tax liability. Write a program to produce the outstanding balance report sorted by customer ID number from the data in the text file. Below is a description of the information on the text file:
* The first line on the file contains the number of customers on the file (numeric)
* The fields below repeat for each customer:
o Customer name (String)
o Customer ID (numeric integer)
o Bill balance (numeric)
o EmailAddress (String)
o Tax liability (numeric or String)
The customers served by the office supply store are of two types: tax-exempt or non-taxexempt. For a tax-exempt customer, the tax liability field on the file is the reason for the tax exemptions: education, non-profit, government, other (String). For a non-tax exempt customer, the tax liability field is the percent of tax that the customer will pay (numeric) based on the state where the customers business resides.
Program requirements: From the information provided, write a solution that includes the following:
* A suitable inheritance hierarchy which represents the customers serviced by the office supply company. It is up to you how to design the inheritance hierarchy. I suggest a Customer class and appropriate subclasses.
* For all classes include the following:
o Instance variables
o Constructors
o Accessor and mutator methods
o Suitable toString() methods
o Any other appropriate methods
* Write a class program8 which utilizes the following:
o An Array of Customer objects
o A method which reads the input file provided and stores the objects in the array of Customers.
o A method which sorts the array of Customers in ascending order by the customer ID.
o A method that formats and prints the output report. The report should include the following requirements:
- Report header at the start of each page showing the name of the company, report and page number.
- Report headers for each column of information.
- One Customer per line.
- At most 45 Customers per page.
- All money amounts and percentages should be displayed with 2 decimal places and commas after each thousand.
o A sample report is shown at the end of the assignment. The report produced does not have to exactly match the sample output provided but must contain all required components listed above.
o Any other helper methods used to meet the requirements of the assignment.
o The main method will call all the other methods which will:
- Read the text file and store the objects in the array
- Sort the objects
- Format and print the report
* Test the program so that there are no errors using the input file provided, program8.txt. Note that the program will only be tested using the file posted on Blackboard. You should not submit another file.
* Record your planning time, coding time, testing time and bug fixing time. Include a comment with your name and this information at the top of the program.
Also I need to use printf for the method rather than for loop to print a list.
Sample Report Output: Note that lines have been omitted to show page headers. Your output does not have to match exactly but all information should be properly labeled, report headers and column headers displayed.
Office Supplies Inc. Customer Report Page 1
=======================
Customer ID Email Address Balance Tax Type Tax Amount
======== == ============= ====== ====== ==========
Threads2 001 purchasing@threads2.com 29,000.00 tax liable 1,450.00
DAV2 002 purchasing@dav2.org 4,860.20 non-profit
DAV3 003 purchasing@dav3.org 4,860.20 non-profit
StopandShop3 004 purchasing@stopandshop2.com 8,100.00 tax liable 486.00
Office Supplies Inc. Customer Report Page 2
=======================
Customer ID Email Address Balance Tax Type Tax Amount
======== == ============= ====== ====== ==========
Threads 349 purchasing@threads.com 29,000.00 tax liable 1,450.00
DAV 358 purchasing@dav.org 4,860.20 non-profit
DAV2 359 purchasing@dav2.org 4,860.20 non-profit
StopandShop 377 purchasing@stopandshop.com 8,100.00 tax liable 486.00
program8.txt
100 Amazon 900 20000.00 purchasing@amazon.com 0.08 Nordstrom 210 50000.00 purchasing@nordstrom.com 0.07 Rutgers 010 32000.00 purchasing@rutgers.edu education Alamo 520 23000.00 purchasing@alamo.com 0.05 Kean 001 158000.50 purchasing@kean.edu education Allied 100 85300.00 purchasing@allied.com 0.06 JoesInc 950 999999.00 purchasing@joesinc.com 0.03 BostonU 697 340020.23 purchasing@tufts.edu education TruckersInc 310 55000.00 purchasing@truckersinc.com 0.10 Clothiers 820 20044.00 purchasing@clothiers.com 0.05 RedCross 849 48900.20 purchasing@redcross.org non-profit ChocolateRus 125 3000.50 purchasing@chocolate.com 0.1 CareBear 535 6000.00 purchasing@carebear.com 0.08 BalloonInc 331 5100.50 purchasing@balloon.com 0.06 TiresInc 345 25000.00 purchasing@tires.com 0.07 JeweleryInc 211 52000.00 purchasing@jewelery.com 0.08 Vassar 002 53000.00 purchasing@vassar.edu education Hertz 521 22200.00 purchasing@hertz.com 0.07 JCU 009 135678.50 purchasing@jcu.edu education Enterprise 120 75300.00 purchasing@enterprise.com 0.06 Avis 958 89999.00 purchasing@avis.com 0.03 Tufts 699 340020.23 purchasing@tufts.edu education Truckers2Corp 910 55000.00 purchasing@truckers2corp.com 0.10 LandsEnd 825 20054.00 purchasing@landsend.com 0.04 TheFutureProject 853 48920.20 purchasing@tfp.org other Bromilows 225 3300.50 purchasing@bromilows.com 0.5 Thrifty 539 6600.00 purchasing@thrify.com 0.07 FlowersRus 339 59300.50 purchasing@flowers.com 0.08 Prudential 660 28900.00 purchasing@prudential.com 0.07 MassMutual 567 5890.00 purchasing@massmutual.com 0.08 Montclair 111 39800.00 purchasing@montclair.edu education SaveTheChildren 578 28760.00 purchasing@savechildren.com non-profit NJDOE 154 158000.50 purchasing@njdoe.gov other ShopRite 333 82100.00 purchasing@shoprite.com 0.07 Turtles 943 88888.00 purchasing@turtles.com 0.08 Brandeis 901 340020.23 purchasing@brandeis.edu education TruckersRus 610 54000.00 purchasing@truckersrus.com 0.08 Clothier&Son 821 20044.00 purchasing@clothiersson.com 0.05 MLH 823 900.20 purchasing@mlh.org other Calandras 725 43000.50 purchasing@calandra.com 0.7 PinkElephant 536 600.00 purchasing@pinkelephant.com 0.05 Essie 338 5000.50 purchasing@essie.com 0.07 Uber 348 1500.00 purchasing@uber.com 0.05 NYDOE 157 12000.50 purchasing@nydoe.gov other Zales 213 54300.00 purchasing@zales.com 0.07 Columbia 322 54500.00 purchasing@columbia.edu education CheapCars 555 23210.00 purchasing@cheapcars.com 0.09 NJIT 099 13978.50 purchasing@NJIT.edu education DAV 358 4860.20 purchasing@dav.org non-profit LLBean 852 2234.00 purchasing@llbean.com 0.03 ArthritisREU 238 4890.20 purchasing@arthritisreu.org other Etsy 902 20400.00 purchasing@etsy.com 0.03 BedBath 212 55000.00 purchasing@Bedbath.com 0.05 UPenn 011 350000.00 purchasing@upenn.edu education EnginesRus 522 23500.00 purchasing@enginesrus.com 0.06 SetonHall 008 158090.50 purchasing@setonhall.edu education TiresToGo 108 8300.00 purchasing@tirestogo.com 0.06 BillShop 956 9999.00 purchasing@billshop.com 0.08 NorthEastern 798 40020.23 purchasing@northeastern.edu education Chocolatiers 318 59000.00 purchasing@chocolatiers.com 0.03 DunkinDonuts 819 2044.00 purchasing@dunkingdonuts.com 0.06 DiabetesOrg 850 4900.20 purchasing@diabetes.org non-profit Beans 126 1000.50 purchasing@beans.com 0.03 BabyGap 538 6100.00 purchasing@babygap.com 0.07 PartyStore 334 500.50 purchasing@partystore.com 0.03 Threads 349 29000.00 purchasing@threads.com 0.05 ColorsInc 218 2000.00 purchasing@colorsinc.com 0.06 CountyCollege1 12 5000.00 purchasing@cc1.edu education BusinessRUs 531 1200.00 purchasing@businessrus.com 0.08 CountyCollege2 109 1678.50 purchasing@cc2.edu education Business2 128 5300.00 purchasing@business2.com 0.02 Oleander 959 2999.00 purchasing@oleander.com 0.04 Harvard 698 34020.23 purchasing@harvard.edu education TaxisInc 914 5000.00 purchasing@taxisinc.com 0.09 Jjill 829 2054.00 purchasing@jjill.com 0.05 CSTA 859 4820.20 purchasing@csta.org other Godiva 229 3100.50 purchasing@godiva.com 0.06 DryCleaners 540 6800.00 purchasing@drycleaners.com 0.05 Macys 332 58200.50 purchasing@macys.com 0.07 CADOE 669 2900.00 purchasing@cadoe.gov.com other Prudential 561 58290.00 purchasing@prudential.com 0.07 Linden 112 3800.00 purchasing@linden.gov other FoodPantry 579 25760.00 purchasing@foodpantry.org non-profit WADOE 159 18000.50 purchasing@wadoe.gov other StopandShop 377 8100.00 purchasing@stopandshop.com 0.06 WholeFoods 953 8888.00 purchasing@wholefoods.com 0.06 FIT 907 30020.23 purchasing@fit.edu education CarRental1 690 5400.00 purchasing@carrental1.com 0.04 Office1 851 2044.00 purchasing@office1.com 0.05 CodeHS 822 1000.20 purchasing@codehs.org other Bakery1 727 4000.50 purchasing@bakery1.com 0.07 BabyToys 539 6000.00 purchasing@babytoys.com 0.04 Google 003 500.50 purchasing@google.com 0.01 NYYankees 019 15500.00 purchasing@nyyankees.com 0.07 Census 155 102000.50 purchasing@census.gov other Fridays 110 5300.00 purchasing@fridays.com 0.06 CUNY 328 5500.00 purchasing@cuny.edu education CheapFood 551 2210.00 purchasing@cheapfood.com 0.04 LegoInc 018 1978.50 purchasing@lego.com 0.02 DAV2 359 4860.20 purchasing@dav2.org non-profit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
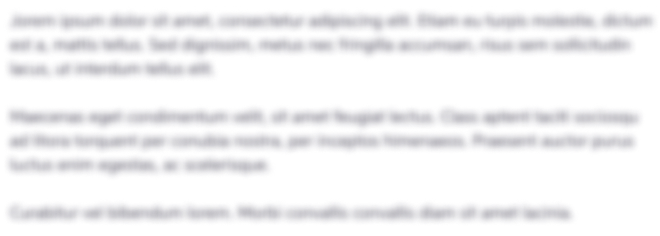
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started