Question
The purpose of this project is to work more with lists, looping, file I/O, functions, and dictionaries. For this assignment, you will work with real
The purpose of this project is to work more with lists, looping, file I/O, functions, and dictionaries. For this assignment, you will work with real data from the web.
Background
Major solar storms are usually associated with peaks in sunspot activity, and sunspot activity is cyclic. These geomagnetic storms can adversely affect electric power grids, disrupt terrestrial electronic telecommunications, and cause disorientation or failure of communications satellites. The next expected peak in sunspot activity is the next likely time for damaging solar storms.
Sunspot data has been collected regularly for over 300 years, and the raw data is available on the web. There are a number of agencies that record sunspot activity. The National Oceanic and Atmospheric Agency (NOAA) is an example of one such agency.
In this project, you will write code that manipulates this data. You are to get the raw sunspot data from the web and calculate the monthly averages. You will be writing two separate programs to do so, each using a different, custom data type that you will be creating! Then you will answer several questions based on your experience creating and using these data structures and finally, looking at that data, predict the next year for solar storms.
Program Specification
We will use data from NOAA's Sunspot Numbers page. Specifically, we are going to be looking at the American Relative Sunspot Numbers data. Conveniently, the data has been published in list form as text files on this page. We will be using the data in the list_aavso-arssn_daily.txt file for this assignment. Download that file and save it in the same directory where you create your Python programs for this project.
In the file, each line has data separated by spaces in this format:
Year Month Day SSN
where SSN is the number of sunspots that were observed on that day.
Be aware that the final data of 2017 is missing, that is, there are lines with year, month, and day, but no sunspot data. Ignore those months.
(On that same web page there are some useful files for checking your results. The list_aavso-arssn_monthly.txt file contains the average numbers of sunspots observed per day for each month. You can use these numbers to ensure your calculations are correct.)
Algorithm
Your high-level algorithm will be as follows:
- Read the raw sunspot data from the input file.
- Programmatically build and populate the data structure with the data from the file.
- Using the data in the data structure compute the average number of sunspots per month.
- Write the computed averaged to a file named monthly_activity.txt using a format similar to the original data (be sure to include appropriate headers in the output file) Each line will have:
year month sunspotAverage
Data Structures
- Part 1
- For Part 1 of the assignment, you will read in and store the data from the file in a data structure composed of lists and tuples.
- Create a list to act as a container for all the data in the file. The elements in this list will themselves be lists.
- These lists will each have 13 elements. Element 0 is the year and the remaining 12 elements will each be another list that contains the data for one of the months of that year. (Note the year 2017 will have less than the full complement of 13 elements.)
- These lists will have 29–32 elements each. Element 0 is a number representing the month and the remaining elements will each be a 2-tuple consisting of the date and the number of sunspots observed on that date.
- An example snippet of this data structure is shown below.
[
[ 1945,
[ 1, (1, 10), (2, 0), (3, 1), (4, 2), (5, 11), (6, 17), (7, 26), (8, 20),
(9, 19), (10, 18), (11, 27), (12, 29), (13, 29), (14, 26), (15, 24),
(16, 20), (17, 14), (18, 28), (19, 20), (20, 23), (21, 30), (22, 31),
(23, 25), (24, 18), (25, 10), (26, 11), (27, 23), (28, 23), (29, 16),
(30, 17), (31, 7)
],
[ 2, (1, 4), (2, 9), (3, 22), (4, 21), (5, 16), (6, 15), (7, 8), (8, 4),
(9, 3), (10, 0), (11, 0), (12, 0), (13, 6), (14, 14), (15, 16), (16, 14),
(17, 13), (18, 18), (19, 23), (20, 15), (21, 1), (22, 0), (23, 20),
(24, 14), (25, 20), (26, 16), (27, 20), (28, 18)
],
[ 3, (1, 16), (2, 28), (3, 21), (4, 22), (5, 19), (6, 14), (7, 13), (8, 16),
(9, 19), (10, 20), (11, 20), (12, 18), (13, 14), (14, 11), (15, 0),
(16, 2), (17, 2), (18, 2), (19, 4), (20, 6), (21, 8), (22, 16), (23, 16),
(24, 16), (25, 19), (26, 30), (27, 39), (28, 46), (29, 51), (30, 53),
(31, 49)
],
[ 4, (1, 48), (2, 47), (3, 50), (4, 43), (5, 40), (6, 36), (7, 24), (8, 12),
(9, 11), (10, 0), (11, 0), (12, 34), (13, 7), (14, 10), (15, 9), (16, 16),
(17, 19), (18, 30), (19, 30), (20, 34), (21, 36), (22, 34), (23, 32),
(24, 40), (25, 47), (26, 53), (27, 56), (28, 51), (29, 44), (30, 56)
],
[ 5, (1, 50), (2, 53), (3, 41), (4, 39), (5, 31), (6, 40), (7, 37), (8, 33),
(9, 16), (10, 11), (11, 2), (12, 1), (13, 0), (14, 13), (15, 29),
(30, 24), (31, 30)
],
...
[ 12, (1, 35), (2, 28), (3, 29), (4, 37), (5, 32), (6, 28), (7, 29),
(8, 21), (9, 18), (10, 23), (11, 23), (12, 26), (13, 39), (14, 42),
(15, 31), (16, 38), (17, 40), (18, 38), (19, 27), (20, 25), (21, 26),
(22, 26), (23, 20), (24, 24), (25, 29), (26, 26), (27, 24), (28, 21),
(29, 16), (30, 20), (31, 39)
]
],
[ 1946,
[ 1, (1, 36), (2, 36), (3, 36), (4, 31), (5, 23), (6, 14), (7, 15), (8, 16),
(9, 29), (10, 35), (11, 29), (12, 34), (13, 97), (14, 117), (15, 100),
(16, 110), (17, 93), (18, 82), (19, 68), (20, 64), (21, 65), (22, 68),
(23, 60), (24, 43), (25, 38), (26, 37), (27, 51), (28, 55), (29, 60),
(30, 74), (31, 95)],
[ 2, (1, 103), (2, 123), (3, 112), (4, 106), (5, 114), (6, 106), (7, 104),
(8, 100), (9, 97), (10, 109),
...
If you want to visualize your data similarly to how I did above take a look at the pprint module. For the output above I used this code (and tweaked the output a bit for clarity):
pp = pprint.PrettyPrinter(indent=2, compact=True)
pp.pprint(sunspot_data)
{
1945: {
1: {
1: 10,
2: 0,
3: 1,
4: 2,
5: 11,
6: 17,
7: 26,
8: 20,
9: 19,
10: 18,
11: 27,
12: 29,
13: 29,
14: 26,
15: 24,
16: 20,
17: 14,
18: 28,
19: 20,
20: 23,
21: 30,
22: 31,
23: 25,
24: 18,
25: 10,
26: 11,
27: 23,
28: 23,
29: 16,
30: 17,
31: 7
},
2: {
1: 4,
2: 9,
3: 22,
4: 21,
5: 16,
6: 15,
7: 8,
8: 4,
9: 3,
10: 0,
11: 0,
12: 0,
13: 6,
14: 14,
15: 16,
...
12: {
1: 35,
2: 28,
3: 29,
4: 37,
5: 32,
6: 28,
7: 29,
8: 21,
9: 18,
10: 23,
11: 23,
12: 26,
13: 39,
14: 42,
15: 31,
16: 38,
17: 40,
18: 38,
19: 27,
20: 25,
21: 26,
22: 26,
23: 20,
24: 24,
25: 29,
26: 26,
27: 24,
28: 21,
29: 16,
30: 20,
31: 39
}
},
1946: {
1: {
1: 36,
2: 36,
3: 36,
...
Functions
build_list(file_name, sunspot_data)
This function takes as an argument the name of the file to open and an empty list (for part one) or an empty dictionary (for part two) and has no return value but does fill build the appropriate data structure as described above.
average_sunspots(sunspot_data, year, month)
This function takes in the initialized sunspot list and two, 2-tuples. Each 2-tuple is in the form (start, end) where start and end are inclusive. The first is a year tuple (with a potential range from 1945 to 2017 inclusive) and the second is a month tuple (with a potential range from 1 to 12 inclusive). It will return an average sunspot value for the given ranges. If no value is given for either tuple, the default is the full range.
main()
This function is written for you in the provided starter files for each part of the project. Do not modify this function. Your code must run with the provided main which is how your code will be tested.
You must provide error checking for all of these functions. The ranges must be correct or an error should be raised. The file must be available or an error should be raised. Think about what errors should be checked. If an error occurs, it should raise an appropriate error (see notes below).
Assignment Notes
- The trickiest part of this part of the project is building the required data structures. Think carefully as you do, and write the code to build it incrementally.
- Do not modify the data file in any way.
- Do not modify the main function in any way.
- Do not hard code the solution. I encourage you to use the list_aavso-arssn_monthly.txt file to check your computations but do not simply write, for example, 18.5 for the average in January 1945. You must write code that computes the average of the daily values for each month in the dataset.
- Do not read the monthly averages from list_aavso-arssn_monthly.txt in your code. You may only use the values in this file to ensure the accuracy of your computed values. You must do this by eye.
- If you have a list of lists (of lists of ...), be careful if you make a copy. (Depending on your algorithm you may or may not be making a copy.) Remember the difference between a shallow copy and a deep copy.
- The raise() function can be used to cause an error to be thrown. It takes as an argument the name of a named error such as KeyError, IndexError, IOError and others. See the documentation at http://docs.python.org/tutorial/errors.html#raising-exceptions
- The IOError of opening a bad file name is generated automatically. However, the KeyError of a bad year range or the IndexError of a bad month range should be raised by your function.
- Please remember the shebang, encoding line, and module-level docstring.
- Every function needs a docstring that describes its arguments and return value and summarizes its purpose.
- Do not zip your files.
Step by Step Solution
3.44 Rating (147 Votes )
There are 3 Steps involved in it
Step: 1
Part 2 For Part 2 of the assignment you will read in and store the data from the file in a data stru...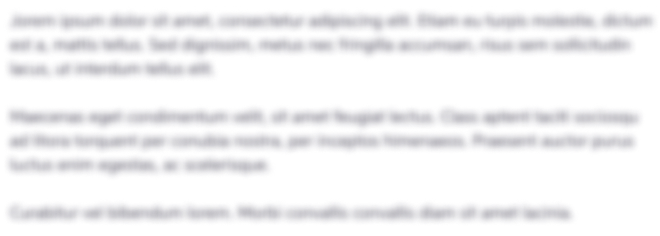
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started