The requirements for this assignment are to:
- Load your custom sprites (128 x 128) into the game engine using Art.txt
- Write the spriteInfo class using the template
- Load your frames (and coordinates) into the ArrayList in the start method
- Create a cursor variable that holds the index of your current displayed frame (relative to your ArrayList). This makes for easy updating of the next frame once a timer is up.
- Using a timer, your ArrayList, and your custom engine, make the images move from the left side of the screen to the right. Once the image reaches the end of the animation, it should reset by making the cursor index 0.
4 images (f1,f2,f3,f4) represent walking stances to imitate a person walking across the screen using queues and ArrayList. Please ask any questions or if you need some more resources. Thanks!
Finish the main.java please. It has been worked on a little and some of the code may not be relevant.
main.java
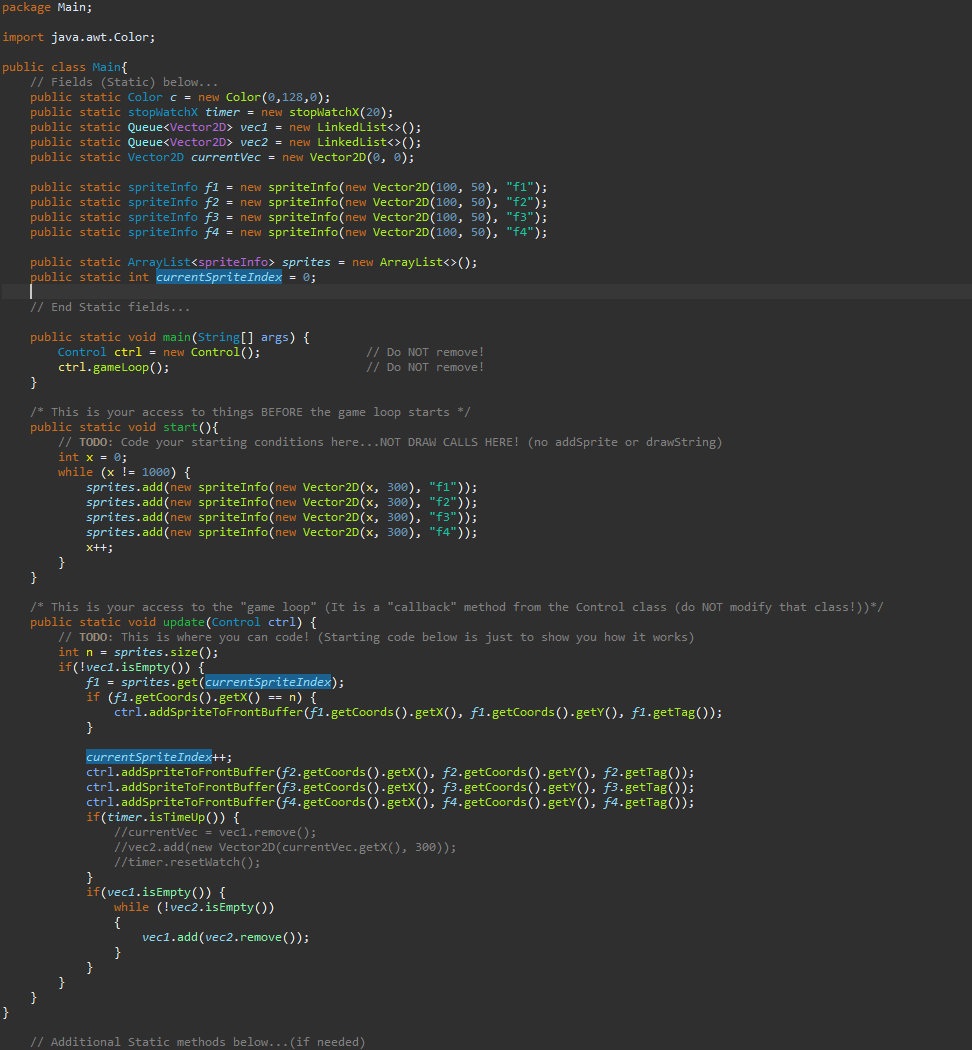
spriteInfo.java
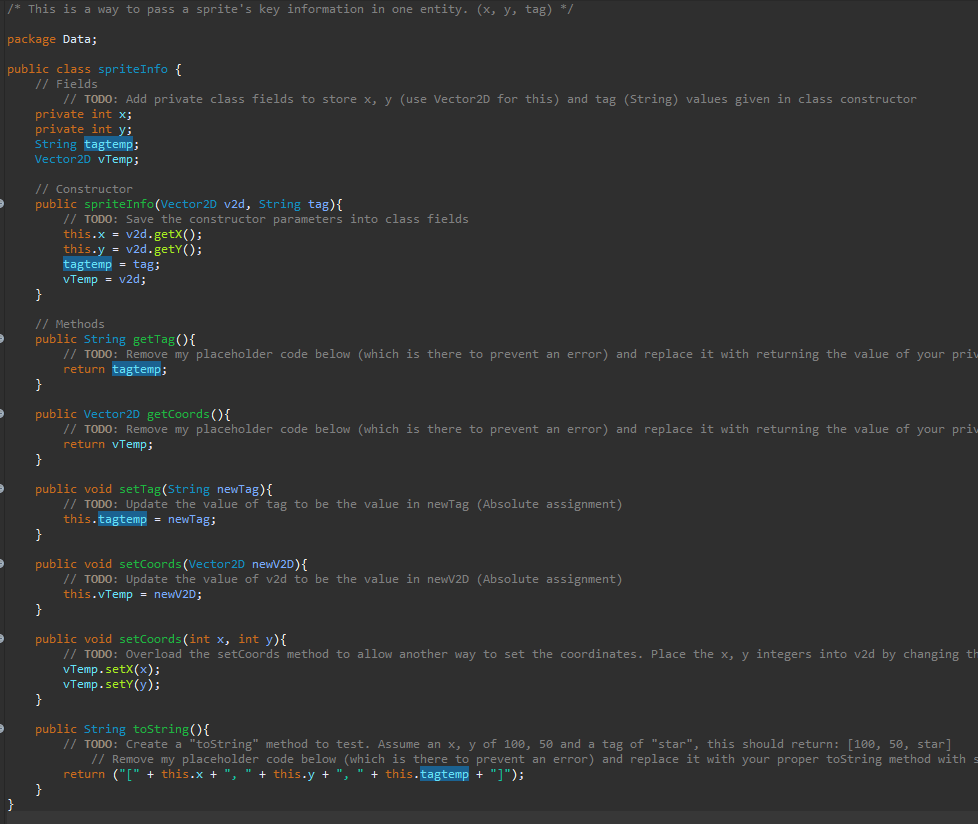
package Main; import java.awt.Color; public class Main{ // Fields (Static) below... public static Color c = new Color(0,128,0); public static stopwatchx timer = new stopwatchx(20); public static Queue
vec = new LinkedList(); public static Queue Vector2D> vec2 = new LinkedList(); public static Vector2D currentVec = new Vector2D(0, 0); public static spriteInfo f1 = new spriteInfo(new Vector2D(100, 50), "f1"); public static spriteInfo f2 = new spriteInfo(new Vector2D(100, 50), "f2"); public static spriteInfo f3 = new spriteInfo(new Vector2D(100, 50), "f3"); public static spriteInfo f4 = new spriteInfo(new Vector2D(100, 50), "f4"); public static ArrayList sprites = new ArrayList(); public static int currentSpriteIndex = 0; // End Static fields... public static void main(String[] args) { Control ctrl = new Control(); ctrl.gameLoop(); } // DO NOT remove! // DO NOT remove! X+; /* This is your access to things BEFORE the game loop starts */ public static void start(){ // TODO: Code your starting conditions here...NOT DRAW CALLS HERE! (no addSprite or drawstring) int x = 0; while (x != 1000) { sprites.add(new spriteInfo(new Vector2D(x, 300), "f1")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f2")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f3")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f4")); } } /* This is your access to the "game loop" (It is a "callback" method from the control class (do NOT modify that class!))*/ public static void update(Control ctrl) { // TODO: This is where you can code! (Starting code below is just to show you how it works) int n sprites.size(); if(!veci.isEmpty()) { f1 = sprites.get(currentSpriteIndex); if (f1.getCoords().getX() == n) { ctrl.addSpriteToFrontBuffer(f1.getCoords().getX(), f1.getCoords().getY(), f1.getTag(); } currentSpriteIndex++; ctrl.addSpriteToFrontBuffer(f2.getCoords().getX(), f2.getCoords().getY(), f2.getTag()); ctrl.addspriteToFrontBuffer (f3.getCoords().getX(), f3.getCoords().getYo, f3.getTag()); ctrl.addSpriteToFrontBuffer (f4.getCoords().getX(), f4.getCoords().getY(), f4.getTag(); if(timer.isTimeUp() { //currentVec = veci.remove(); //vec2.add(new Vector2D(currentVec.getX(), 300)); //timer.resetWatch(); f(vec1. isempty() { while (!vec2.isEmpty()) { veci.add(vec2.remove()); } } // Additional Static methods below...(if needed) /* This is a way to pass a sprite's key information in one entity. (x, y, tag) */ package Data; public class spriteInfo { // Fields // TODO: Add private class fields to store x, y (use Vector2D for this) and tag (String) values given in class constructor private int x; private int y; String tagtemp; Vector 2D vTemp; // Constructor public spriteInfo(Vector2D v2d, String tag){ // TODO: Save the constructor parameters into class fields this.x = v2d.getX(); this.y = v2d.getY(); tagtemp = tag; vTemp = v2d; } // Methods public String getTag(){ // TODO: Remove my placeholder code below (which is there to prevent an error) and replace it with returning the value of your priv return tagtemp; } public Vector2D getCoords(){ // TODO: Remove my placeholder code below (which is there to prevent an error) and replace it with returning the value of your priv return vTemp; } public void setTag (String newTag) { // TODO: Update the value of tag to be the value in newtag (Absolute assignment) this.tagtemp = newTag; } public void setCoords (Vector2D newV2D) { // TODO: Update the value of v2d to be the value in newV2D (Absolute assignment) this.vTemp = newV2D; } public void setCoords(int x, int y){ // TODO: Overload the setCoords method to allow another way to set the coordinates. Place the x, y integers into v2d by changing th Temp.setx(x); vTemp.setY(y); } public String toString(){ // TODO: Create a "toString" method to test. Assume an x, y of 100, 50 and a tag of "star", this should return: [100, 50, star] // Remove my placeholder code below (which is there to prevent an error) and replace it with your proper toString method with s return ("[" + this.x + ", + this.y + ", + this.tagtemp + "]"); } } package Main; import java.awt.Color; public class Main{ // Fields (Static) below... public static Color c = new Color(0,128,0); public static stopwatchx timer = new stopwatchx(20); public static Queue vec = new LinkedList(); public static Queue Vector2D> vec2 = new LinkedList(); public static Vector2D currentVec = new Vector2D(0, 0); public static spriteInfo f1 = new spriteInfo(new Vector2D(100, 50), "f1"); public static spriteInfo f2 = new spriteInfo(new Vector2D(100, 50), "f2"); public static spriteInfo f3 = new spriteInfo(new Vector2D(100, 50), "f3"); public static spriteInfo f4 = new spriteInfo(new Vector2D(100, 50), "f4"); public static ArrayList sprites = new ArrayList(); public static int currentSpriteIndex = 0; // End Static fields... public static void main(String[] args) { Control ctrl = new Control(); ctrl.gameLoop(); } // DO NOT remove! // DO NOT remove! X+; /* This is your access to things BEFORE the game loop starts */ public static void start(){ // TODO: Code your starting conditions here...NOT DRAW CALLS HERE! (no addSprite or drawstring) int x = 0; while (x != 1000) { sprites.add(new spriteInfo(new Vector2D(x, 300), "f1")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f2")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f3")); sprites.add(new spriteInfo(new Vector2D(x, 300), "f4")); } } /* This is your access to the "game loop" (It is a "callback" method from the control class (do NOT modify that class!))*/ public static void update(Control ctrl) { // TODO: This is where you can code! (Starting code below is just to show you how it works) int n sprites.size(); if(!veci.isEmpty()) { f1 = sprites.get(currentSpriteIndex); if (f1.getCoords().getX() == n) { ctrl.addSpriteToFrontBuffer(f1.getCoords().getX(), f1.getCoords().getY(), f1.getTag(); } currentSpriteIndex++; ctrl.addSpriteToFrontBuffer(f2.getCoords().getX(), f2.getCoords().getY(), f2.getTag()); ctrl.addspriteToFrontBuffer (f3.getCoords().getX(), f3.getCoords().getYo, f3.getTag()); ctrl.addSpriteToFrontBuffer (f4.getCoords().getX(), f4.getCoords().getY(), f4.getTag(); if(timer.isTimeUp() { //currentVec = veci.remove(); //vec2.add(new Vector2D(currentVec.getX(), 300)); //timer.resetWatch(); f(vec1. isempty() { while (!vec2.isEmpty()) { veci.add(vec2.remove()); } } // Additional Static methods below...(if needed) /* This is a way to pass a sprite's key information in one entity. (x, y, tag) */ package Data; public class spriteInfo { // Fields // TODO: Add private class fields to store x, y (use Vector2D for this) and tag (String) values given in class constructor private int x; private int y; String tagtemp; Vector 2D vTemp; // Constructor public spriteInfo(Vector2D v2d, String tag){ // TODO: Save the constructor parameters into class fields this.x = v2d.getX(); this.y = v2d.getY(); tagtemp = tag; vTemp = v2d; } // Methods public String getTag(){ // TODO: Remove my placeholder code below (which is there to prevent an error) and replace it with returning the value of your priv return tagtemp; } public Vector2D getCoords(){ // TODO: Remove my placeholder code below (which is there to prevent an error) and replace it with returning the value of your priv return vTemp; } public void setTag (String newTag) { // TODO: Update the value of tag to be the value in newtag (Absolute assignment) this.tagtemp = newTag; } public void setCoords (Vector2D newV2D) { // TODO: Update the value of v2d to be the value in newV2D (Absolute assignment) this.vTemp = newV2D; } public void setCoords(int x, int y){ // TODO: Overload the setCoords method to allow another way to set the coordinates. Place the x, y integers into v2d by changing th Temp.setx(x); vTemp.setY(y); } public String toString(){ // TODO: Create a "toString" method to test. Assume an x, y of 100, 50 and a tag of "star", this should return: [100, 50, star] // Remove my placeholder code below (which is there to prevent an error) and replace it with your proper toString method with s return ("[" + this.x + ", + this.y + ", + this.tagtemp + "]"); } }