Question
the Rescue Due Mar 28 by 11:59pm Points 150 Submitting a file upload Coding Projects 150 pts You are an airport operations software engineer. Part
the Rescue Due Mar 28 by 11:59pm Points 150 Submitting a file upload Coding Projects 150 pts You are an airport operations software engineer. Part of your job is to write a program that manages a computerized list of departures and arrivals to/from your small impoverished island's single busy airport. Because of the condition of many of the airplanes in your country's airline, airline crashes are very frequent, often several per day. When an airplane crashes, you remove that aircraft from your flight schedule, which conveniently opens spots in your list for other aircraft, which are frequently standing by, waiting for a slot to open up. Additionally, since the island's airport is small, arriving pilots frequently make navigation errors and land on the wrong island. This also opens up slots in your list. Additionally, social cohesion is poor in your country, and flights are frequently canceled because of belligerent passengers and fistfights in the aisles of aircraft. These aircraft are also pulled from the list at the last minute. Mechanical problems, drunk pilots, missing, possibly repossessed planes, and strikes, are other operational challenges causing you and your software untold headaches and flight cancellations. If that weren't enough, the corrupt president of your country is very moody and travels to and from your airport for last-minute shopping junkets and "diplomatic" excursions. His flights are almost always unscheduled and because hes the president, his plane jumps the line of scheduled departures and arrivals. The presidents top cronies and generals have similar privileges and frequently also jump the queue on their junkets into and out of the country on private or military planes. As a well-trained software engineer, luckily, you have had the foresight of using a linked list for your flight schedules. Link lists, luckily for you, are very efficient for performing frequent delete and insert operations on a list, and adding items to the front or end of the list. If you had instead used a regular list like an ArrayList, (which would require complex shifting of items in your list when doing these types of operations), your country might be even more chaotic! You deserve a raise for your good thinking, but unfortunately, you have not been paid for several months because of corruption and mismanagement of the airport budget. * * * * * * * Here is a class diagram showing the attributes and operations of your system. Following the diagram, is a table describing the purpose of the operations listed. You should understand the class diagram and operations descriptions before starting to code. LinkedListAircraftOps.JPG Class/Enum Method Name Description FlightOps initializeFlightList eads in a sample flight list into a LinkedList from a CSV file. ReadCSVWithScanner LinkedList getFlightListFromCSV(String filePath) eads in a sample flight list into a LinkedList from a CSV file. FlightOps void doSimuluation() driver method for airport operations simulation - initializes the LinkedList, modifies statuses randomly, and then processes the status changes, modifying the LinkedList as necessary FlightsOps void changeStatuses() Changes statuses from default Scheduled to random values FlightOps void removeCancelledFlights() Removes flights with statuses causes cancellation of flight FlightOps void printFlights() Prints a list of flights in the LinkedList FlightOps void moveQueuedFlights() { Flights with status Queued should be moved to the end of the LinkedList FlightOps void presidentAndCroniesJumpTheQueue() President and cronies jump to the front of the list on demand. Sample of Linked List Methods you will likely find useful. Use as many of these as you can: addLast(); addFirst(); add(Flight e) indexOf(Object o) getFirst() getLast(); peek(); peekLast(); poll() or remove() pollLast(); peekLast(); add(index, element) Preliminary Instructions: Download and extract the provided stubbed out project zip file into your IdeaProjects home directory. Open the project. This project will compile but is incomplete. Your job will be to correct it. Note that a provided Flights.csv (Comma-Delimited-Value) file is located in the resources directory under your src directory in IntelliJ. Take a look at this file. There is also a file called Employees.csv in this directory. ReadCSVFromScannerEmployee has a main method and you can run ReadCSVFromScannerEmployee from your project. Just open this source file and select the second Run menu and you will be able to pick which Runnable to execute. This will load and print out the data in the Employees.csv file. See the Tutorial on CSV files in Unit 9 so that you understand how to use CSV files. Examine the Flight class in the project. Modify the class ReadCSVWithScanner patterning your code from the ReadCSVFromScannerEmployee class. Your modified class should be able to read and process the Flights.CSV file without errors. Test it successfully before proceeding. DoSimulation() method in the FlightOps class: Several methods from the FlightOps class called below are stubbed out in the project file provided and will require you to supply needed code where indicated. You will need to complete the printFlights() method, and add the appropriate LinkedList methods addFirst(), addLast(), add(), and remove(), where appropriate. You are welcome to add features or other operations to make this project your own. A discussion of the doSimulation method follows below. public void doSimuluation(String filePath) { flts = initializeFlightList(filePath); changeStatuses(); System.out.println("Changed statuses"); printFlights(); System.out.println("Remove cancelled flights"); removeCancelledFlights(); printFlights(); presidentAndCroniesJumpTheQueue(); System.out.println("Cronies jump queue"); printFlights(); moveQueuedFlights(); System.out.println("Moved queued flights"); printFlights(); } public LinkedList initializeFlightList(String filePath) { // Load flights from external file ReadCSVWithScanner csvReader = new ReadCSVWithScanner(); LinkedList fltList = csvReader.getFlightListFromCSV(filePath); return fltList; } public LinkedList initializeFlightList(String filePath) { // Load flights from external file ReadCSVWithScanner csvReader = new ReadCSVWithScanner(); LinkedList fltList = csvReader.getFlightListFromCSV(filePath); return fltList; } public static void main(String[] args) { FlightOps fltOPs = new FlightOps(); String filePath = "./resources/Flights.csv"; fltOPs.doSimuluation(filePath); } What happens in DoSimulation() The method initializeFlightList() builds a LinkedList of flights with a default status of Scheduled The method changeStatuses() is then called to set the statuses of the status to a random status to make our operations more interesting. Certain statuses make sense only for arrivals or departures. Examine the setOperationStatus() and understand how it works. OperationStatuses that will result in a flight cancellation are identified and those flights are removed from the list. The operation uses the remove() method The president and his cronies can jump the queue, ThreeVIP flights are created and added to the list. Finally, flights with the status of Queued are moved to the bottom of the LinkedList using the addLast method. Note that we cannot simply use for-next loops to remove or move items, since that would affect the for loop and create a concurrency exception. We, therefore, use a Stack to keep track of items to be removed or moved while going through the for loop, in a separate loop popping them off the stack to do the remove operation. Submission Instructions: Submit Java source code files that solve the defined problems. Submit a screenshot of your test output for each exercise. Rubric Lab 3: Unit 3 (2) (1) (1) Lab 3: Unit 3 (2) (1) (1) Criteria Ratings Pts This criterion is linked to a Learning Outcome Exercise 1: Java souce code file and test output 50 to >0.0 pts Full Marks 0 pts No Marks 50 pts This criterion is linked to a Learning Outcome Exercise2: Java souce code file and test output 50 pts Full Marks 0 pts No Marks 50 pts This criterion is linked to a Learning Outcome DPR 101.1 Identify and select appropriate programming tools for application development. Identify and select appropriate programming tools for application development. threshold: 3.0 pts 5 pts Exceeds Expectation 4 pts - 3 pts Meets Expectations 2 pts - 1 pts Does Not Meet Expectations 0 pts No Evidence -- This criterion is linked to a Learning Outcome DPR 101.6 Use appropriate tools and strategies for debugging and avoiding errors. Use appropriate tools and strategies for debugging and avoiding errors. threshold: 3.0 pts 5 pts Exceeds Expectation 4 pts - 3 pts Meets Expectations 2 pts - 1 pts Does Not Meet Expectations 0 pts No Evidence -- This criterion is linked to a Learning Outcome DPR 101.10 Learn about using computers to process information, find patterns and test hypotheses about digitally processed information to gain insight and knowledge. Learn about using computers to process information, find patterns and test hypotheses about digitally processed information to gain insight and knowledge. threshold: 3.0 pts 5 pts Exceeds Expectation 4 pts - 3 pts Meets Expectations 2 pts - 1 pts Does Not Meet Expectations 0 pts No Evidence --
Step by Step Solution
There are 3 Steps involved in it
Step: 1
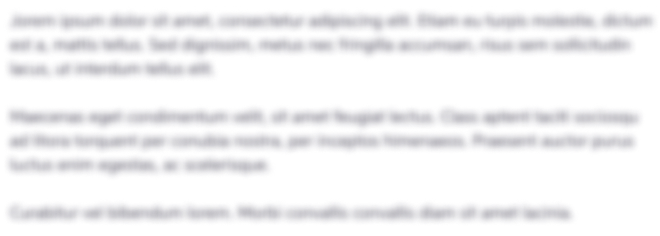
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started