Question
The script must have 3 functions: get_command_line_arguments print_path_dirs executable_file_count This function must have the following header: def get_command_line_arguments(arg_number): This function is used to get a
The script must have 3 functions:
- get_command_line_arguments
- print_path_dirs
- executable_file_count
This function must have the following header:
def get_command_line_arguments(arg_number):
This function is used to get a certain number of command line arguments, specified by the parameter arg_number and return them as a tuple. If the user does not enter the proper number of the command line arguments, the code should print a usage message and exit the script. This message must have the following format
Usage: SCRIPT_NAME ARGUMENT_1 ARGUMENT_2
If the proper number of command line arguments has been provided, the function should return their values in a tuple.
print_path_dirs
This function must have the following header:
def get_command_line_arguments:
This function should print the pathnames of directories listed in the Unix PATH variable. Each pathname should be on a separate line.
executable_file_count
This function must have the following header:
def executable_file_count(dir):
This function should count the number of files with a .py extension and return that value.
Script for this assignment
Open an a text editor and create the file hw6.py.
You can use the editor built into IDLE or a program like Sublime.
Test Code
Your hw6.py file must contain the following test code at the bottom of the file:
get_command_line_arguments(2) agr1, arg2 = get_command_line_arguments(2) print(agr1, arg2) print() print_path_dirs() print() dir = "/home/it576gh/bin/python/edu: 0" py_count = executable_file_count(dir) print("Python files", py_count)
Suggestions
Write this program in a step-by-step fashion using the technique of incremental development.
In other words, write a bit of code, test it, make whatever changes you need to get it working, and go on to the next step.
- Create the file hw6.py. Import the os and sys modules. Enter the headers for each of the required functions. Under each header write the Python statement pass. Run the script. Fix any errors you find.
- Remove the pass statement from get_command_line_arguments. Write a statement that prints the list of things on the command line. Copy the test code to the bottom of the script. Comment out all but the first line of the code. Run the script with 0, 1 and 2 command line arguments. Fix any errors you find.
- Remove the print statement. Add an if statement that will execute if the user has entered fewer than the number of arguments specified by the parameter arg_number. Remember that the first entry in the list of things on the command line is the name of the script. So the length of the list of command line entries is NOT the number command line arguments. The body of this if statement should print "ERROR". Under the print statement write a statement that quits the script. Run the script with 0, 1 and 2 command line arguments. Fix any errors you find.
- Replace the error message with the usage message given above. Remember I want the name of the script not the pathname used to run it. Run the script with 0, 1 and 2 command line arguments. Fix any errors you find.
- Outside the if statement, return the first two command line arguments. Uncomment the next two lines in the test code. Run the script with 2 command line arguments. You should see the script print these two arguments. Fix any errors you find.
- Remove the pass statement from print_path_dirs. Add a print statement that prints the value of the system variable PATH. To get this value you will need to use a variable in the os module that points to a dictionary where system variable names are the keys and the value are the value of that system variable. Uncomment the next two lines in the test code. Run the script with 2 command line arguments. Fix any errors you find.
- Remove the print statement. The PATH system variable contains the absolute pathnames of directories holding executable files. Each of these pathnames is separated from the next with a :. Create a list of directories using the string split method on the value of the PATH variable. You will have to give split the right argument to make this work. Print this list. Run the script with 2 command line arguments. Fix any errors you find.
- Remove the print statement. Replace it with a for loop that prints each directory in the list. Run the script with 2 command line arguments. You should see a list of directories on your machine. Fix any errors you find.
- Remove the pass statement from executable_file_count. Add statement that will change directory to the pathname contained the dir parameter. Add a statement that create a list of entries in the current directory. Print this list. Uncomment the next two lines in the test code.
- Run the script with 2 command line arguments. Fix any errors you find.
- Remove the print statement. Write a for loop that iterates through the list of directory entries. Inside the for loop write an if statement that print an entry if it is a file. Fix any errors you find.
- Before the for loop, create the variable count and set is to 0. Remove the print statement. Replace it with an if statement that looks for files whose name ends in ".py". In the body of this if statement write a statement which increments count by 1. Outside the for loop return the value of count. Uncomment the last line of the test code. Run the script with 2 command line arguments. The number of ".py" files should be 7. Fix any errors you find.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
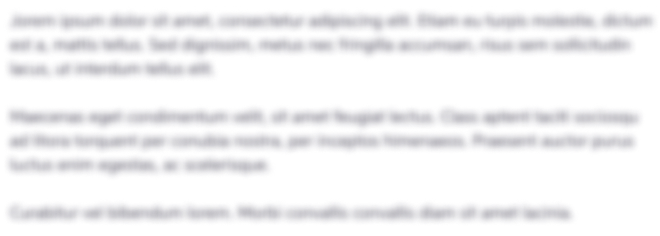
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started