There is no arrayTree or testTreeADT description!!!!!
1. Problem Description You are to develop a program that plays the perfect game of TicTacToe. It should never lose. Your project can be done in three steps of increasing complexity.
Develop the Tree ADT as an array-based concrete implementation.
For the program, there is no code to start with; you are to develop everything. However, the UML design and test drivers for the Tree ADT have been supplied so you can test your implementation. You can review the sample output below to get an idea of the interaction.
Create the Array-based Tree ADT. Run it with TestTreeADT.java to insure it works.
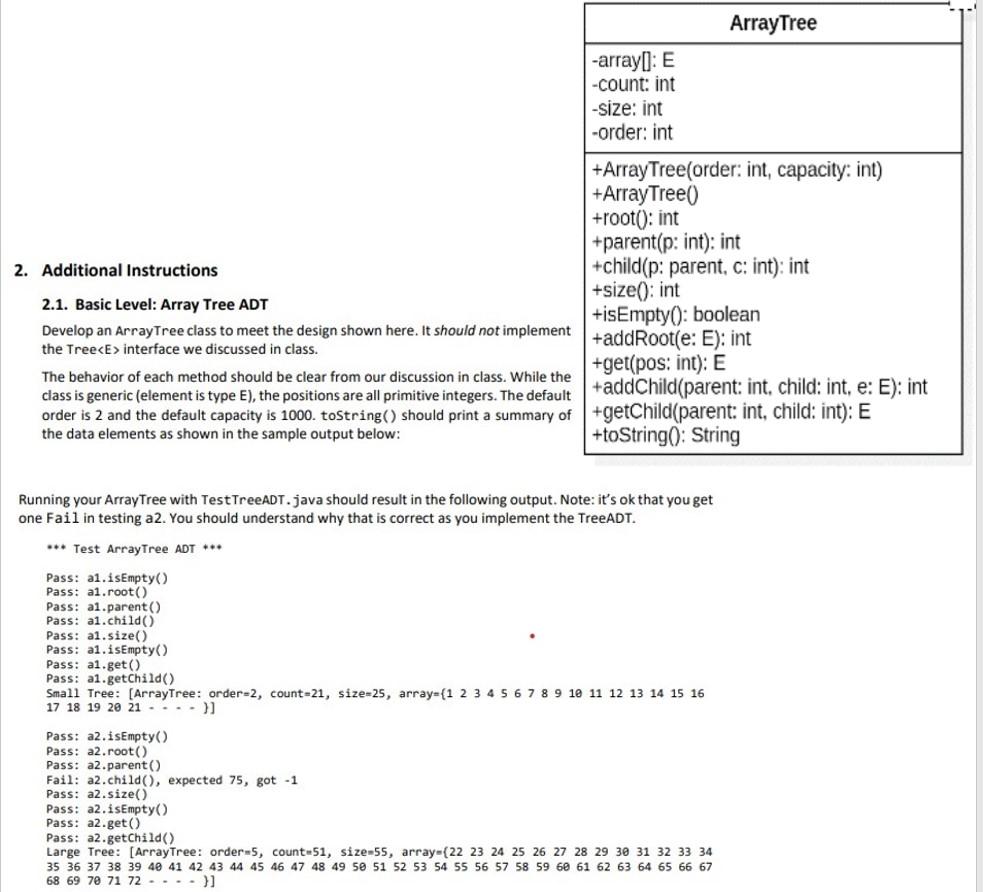
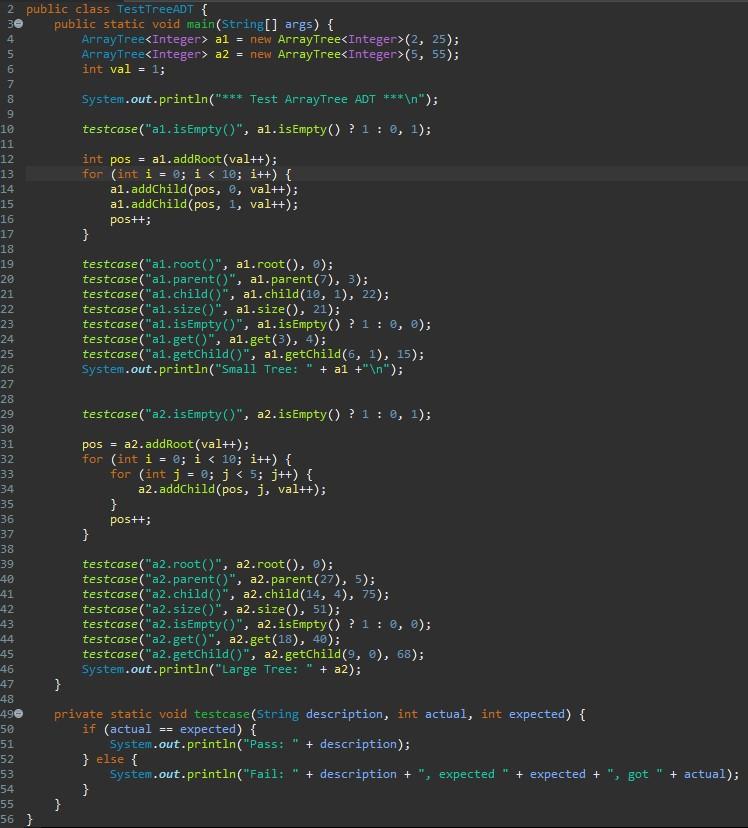
ArrayTree -array(): E -count: int -size: int -order: int +Array Tree(order: int, capacity: int) +Array Tree() +root(): int +parent(p: int): int 2. Additional Instructions +child(p: parent, c: int): int +size(): int 2.1. Basic Level: Array Tree ADT Develop an ArrayTree class to meet the design shown here. It should not implement +addRoot(e: E): int +isEmpty(): boolean the Tree
interface we discussed in class. +get(pos: int): E The behavior of each method should be clear from our discussion in class. While the class is generic (element is type E), the positions are all primitive integers. The default +addChild(parent: int, child: int, e: E): int order is 2 and the default capacity is 1000. toString() should print a summary of+getChild(parent: int, child: int): E the data elements as shown in the sample output below: +toString(): String Running your ArrayTree with Test TreeADT.java should result in the following output. Note: it's ok that you get one Fail in testing a2. You should understand why that is correct as you implement the TreeADT. *** Test Array Tree ADT *** Pass: a1.isEmpty() Pass: a1.root() Pass: al.parent() Pass: a1.child() Pass: al.size() Pass: a1.isEmpty() Pass: al.get() Pass: a1.getChild() Small Tree: [ArrayTree: order-2, count-21, size=25, array={1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 ...)] Pass: a2.isEmpty() Pass: a2.root() Pass: a2.parent() Fail: a2.child(), expected 75, got -1 Pass: a2.size() Pass: a2.isEmpty() Pass: a2.get() Pass: a2.getChild() Large Tree: [ArrayTree: order-5, count=51, size-55, array-( 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 ....) 4 6 7 8 9 10 12 13 14 2 public class TestTreeADT { @ public static void main(String[] args) { ArrayTree a1 = new ArrayTree Integer>(2, 25); ArrayTree Integer> a2 = new ArrayTree Integer>(5, 55); int val = 1; System.out.println("*** Test ArrayTree ADT *** "); testcase("al.isEmpty()", al.isEmpty(?1 : , 1); 11 int pos = a1.addRoot(val++); for (int i = 0; i interface we discussed in class. +get(pos: int): E The behavior of each method should be clear from our discussion in class. While the class is generic (element is type E), the positions are all primitive integers. The default +addChild(parent: int, child: int, e: E): int order is 2 and the default capacity is 1000. toString() should print a summary of+getChild(parent: int, child: int): E the data elements as shown in the sample output below: +toString(): String Running your ArrayTree with Test TreeADT.java should result in the following output. Note: it's ok that you get one Fail in testing a2. You should understand why that is correct as you implement the TreeADT. *** Test Array Tree ADT *** Pass: a1.isEmpty() Pass: a1.root() Pass: al.parent() Pass: a1.child() Pass: al.size() Pass: a1.isEmpty() Pass: al.get() Pass: a1.getChild() Small Tree: [ArrayTree: order-2, count-21, size=25, array={1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 ...)] Pass: a2.isEmpty() Pass: a2.root() Pass: a2.parent() Fail: a2.child(), expected 75, got -1 Pass: a2.size() Pass: a2.isEmpty() Pass: a2.get() Pass: a2.getChild() Large Tree: [ArrayTree: order-5, count=51, size-55, array-( 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 ....) 4 6 7 8 9 10 12 13 14 2 public class TestTreeADT { @ public static void main(String[] args) { ArrayTree a1 = new ArrayTree Integer>(2, 25); ArrayTree Integer> a2 = new ArrayTree Integer>(5, 55); int val = 1; System.out.println("*** Test ArrayTree ADT *** "); testcase("al.isEmpty()", al.isEmpty(?1 : , 1); 11 int pos = a1.addRoot(val++); for (int i = 0; i