Question
There is something wrong with this code. It goes into segfault when n = 50,000. What do I need to add/change? changing int to long
There is something wrong with this code. It goes into segfault when n = 50,000. What do I need to add/change? changing int to long int does not work, Thank you
#include #include #include #include #include #include
typedef struct monster { int id; char name[64]; char element[64]; int population; double weight; } monster;
monster *make_some_monsters(int n) { monster *monsters = malloc(sizeof(monster) * n);
time_t t;
srand((unsigned) time(&t));
for(int i = 0; i { monsters[i].id = i; sprintf(monsters[i].name, "Monster #%d", rand()); sprintf(monsters[i].element, "Element #%d", rand()); monsters[i].population = rand(); monsters[i].weight = 500.0 * ((double) rand() / (double) RAND_MAX); }
return monsters; }
void output_monster_list(monster *list, int n, char *title) { printf("List %s: ", title); for(int i = 0; i printf(" Monster %d: %s %s %d %lf ", i, list[i].name, list[i].element, list[i].population, list[i].weight); } printf(" "); }
void print_clocks(clock_t clocks) { printf(" %lfs CPU time used ", ((double) clocks) / CLOCKS_PER_SEC); }
void swap_monsters(monster *list, int i, int j) { monster temp;
memcpy(&temp, list + i, sizeof(monster)); memcpy(list + i, list + j, sizeof(monster)); memcpy(list + j, &temp, sizeof(monster)); }
void check_monster_sort(monster *list, int n, int use_name, int use_weight) { for(int i = 1; i if(compare_monsters(list + i - 1, list + i, use_name, use_weight) > 0) { printf("*** The list is NOT sorted. "); return; } } printf("The list is sorted. "); }
void merge_sort_merge(monster *list, int l1, int h1, int l2, int h2, int *comparisons, int *copies, int *block_copies, int *mallocs, int use_name, int use_weight) {
monster temp[l2+h2]; int index = l1; int k=l1; (*mallocs)++; (*block_copies)++ ; while(l1=h1> (*comparisons)++; if(use_name == 1){ if(strcmp(list[l1].name, list[l2].name) (*copies)++; temp[index] = list[l1]; l1 = l1+1; }else{ (*copies)++; temp[index] = list[l2]; l2 = l2+1; } } if(use_weight == 1){ if(list[l1].weight (*copies)++; temp[index] = list[l1]; l1 = l1+1; }else{ (*copies)++; temp[index] = list[l2]; l2 = l2+1; } } index++; }
if(l1>h1){ (*block_copies)++; while(l2 (*copies)++; (*comparisons)++; temp[index] = list[l2]; index++; l2++; } }else{ (*block_copies)++; while(l1 (*copies)++; (*comparisons)++; temp[index] = list[l1]; index++; l1++; } } (*block_copies)++; while(k (*copies)++; list[k]=temp[k]; k++; }
void merge_sort_recursive(monster *list, int low_index, int high_index, int *comparisons, int *copies, int *block_copies, int *mallocs, int use_name, int use_weight) { // YOUR CODE GOES HERE. int m; if (low_index m = (low_index + high_index) / 2; merge_sort_recursive(list, low_index, m, comparisons, copies, block_copies, mallocs, use_name, use_weight);
merge_sort_recursive(list, m + 1, high_index, comparisons, copies, block_copies, mallocs, use_name, use_weight);
merge_sort_merge(list, low_index, m, m + 1, high_index, comparisons, copies, block_copies, mallocs, use_name, use_weight); }
}
/* Implement merge sort. */
void merge_sort(monster *list, int n, int use_name, int use_weight) { int comparisons = 0; int copies = 0; int block_copies = 0; int mallocs = 0; clock_t start_cpu, end_cpu;
printf("Merge sort %d monsters... ", n);
start_cpu = clock(); merge_sort_recursive(list, 0, n-1, &comparisons, &copies, &block_copies, &mallocs, use_name, use_weight); end_cpu = clock();
printf("Sort complete with %d comparisons, %d block copies, %d total copies, %d mallocs. ", comparisons, block_copies, copies, mallocs); print_clocks(end_cpu - start_cpu); }
/* Recursive function for merge-insertion sort. */
void merge_insertion_sort_recursive(monster *list, int low_index, int high_index, int *comparisons, int *copies, int *block_copies, int *mallocs, int use_name, int use_weight) { // YOUR CODE GOES HERE. int n = high_index+1; if(n insertion_sort_internal(list, n, comparisons, copies,block_copies, use_name, use_weight); }else{ merge_sort_recursive(list, 0, high_index, comparisons, copies, block_copies, mallocs, use_name, use_weight); }
}
/* Implement merge sort. */
void merge_insertion_sort(monster *list, int n, int use_name, int use_weight) { int comparisons = 0; int copies = 0; int block_copies = 0; int mallocs = 0; clock_t start_cpu, end_cpu;
printf("Merge-insertion sort %d monsters... ", n);
start_cpu = clock(); merge_insertion_sort_recursive(list, 0, n-1, &comparisons, &copies, &block_copies, &mallocs, use_name, use_weight); end_cpu = clock();
printf("Sort complete with %d comparisons, %d block copies, %d total copies, %d mallocs. ", comparisons, block_copies, copies, mallocs); print_clocks(end_cpu - start_cpu); }
/* Main program. */
void run_all_sorts(int n, int only_fast, int use_name, int use_weight) { monster *our_list = make_some_monsters(n); monster *our_unsorted_list = malloc(sizeof(monster) * n);
printf("SORT SET: n = %d, %s, by %s ", n, only_fast ? "fast sorts only" : "all sorts", use_name ? "name" : "weight");
if(only_fast == 0) {
memcpy(our_unsorted_list, our_list, sizeof(monster) * n); merge_sort(our_unsorted_list, n, use_name, use_weight); check_monster_sort(our_unsorted_list, n, use_name, use_weight);
memcpy(our_unsorted_list, our_list, sizeof(monster) * n); merge_insertion_sort(our_unsorted_list, n, use_name, use_weight); check_monster_sort(our_unsorted_list, n, use_name, use_weight);
printf("SORT SET COMPLETE ");
free(our_list); free(our_unsorted_list); }
int main(void) { run_all_sorts(50, 0, 0, 1); run_all_sorts(50, 0, 1, 0); run_all_sorts(500, 0, 0, 1); run_all_sorts(500, 0, 1, 0); run_all_sorts(5000, 0, 0, 1); run_all_sorts(5000, 0, 1, 0); run_all_sorts(50000, 1, 0, 1); run_all_sorts(50000, 1, 1, 0); run_all_sorts(500000, 1, 0, 1); run_all_sorts(500000, 1, 1, 0);
return 0;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
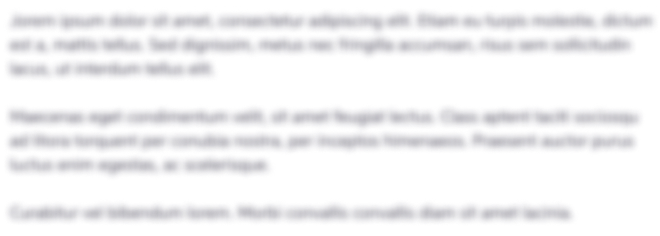
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started