Question
There's two small things we must do before we start, we're going to create a main.c file that we will use to test the functions
There's two small things we must do before we start, we're going to create a "main.c" file that we will use to test the functions we write, and a header file "lab3.h" to prototype any of the functions we write.
Note: Instead of recreating the "lab3.h" file every time we add something, we will simply create one header function with all of the required protypes and libraries.
%%file include/lab3.h
#include
/*** * Function Prototypes ***/
/* 2. reverseList.c */ void swapIntegers(int *one, int *two);
void reverseIntegers(int list[], int length);
/* 3. incrementPointer.c */ void nextChar(char **position);
char *findChar(char *string, char toFind);
/* 4. commandLineArguments.c */ void sortIntegers(int value[], int length);
int convertToInt(char *string);
int *sortArguments(int argc, char *argv[]);
/* 5. 2dArray.c */ void printTable(int *arr[], int columnLine, int numRows, int numColumns);
2. Passing an Array (2 marks) Rather than passing members of an array individually, it's often convenient to pass the entire array. This way the code is more modular (so it can be reused) and the array can be entirely processed.
In this section we are going to write two functions, the first accepts an array of integers to reverse the order without returning another array and the second swaps two integers.
Note: You can use either of the following function signatures for the print list function, but we will use the first one.
void reverseIntegers(int list[], int length) void reverseIntegers(int *list, int length) Hint: Use a while loop when reversing the list. It may not be apparent immediately, but there's a simple solution.
Do not skip the "swapIntegers" function, it will be used again later!
%%file src/reverseList.c
/* Swaps the value of two integers by swapping the pointers */ void swapIntegers(int *one, int *two) {
}
/* Reverse the order of a list of integers. */ void reverseIntegers(int list[], int length) {
}
%%file src/main.c
#include "lab3.h"
void printList(int *list, int length) { int i; for (i = 0; i < length; i++) { printf("%d, ", list[i]); } printf(" "); }
int main(int argc, char *argv[]) { /* Notice that there are at least one even and one odd list being tested.*/ int intList[] = {1, 2, 3, 4, 5}; int intKnownList[4] = {6, 7, 8, 9}; printf("Hello, World "); printf("List 1: "); printList(intList, 5); reverseIntegers(intList, 5); printList(intList, 5); printf("List 2: "); printList(intKnownList, 4); /* Something interesting about memory in c, try switching the length below from 4 to 5*/ reverseIntegers(intKnownList, 4); printList(intKnownList, 4); return 0; }
3. Relation Between a Pointer and Array (2 marks) As mentioned previously, we could have used two different function signatures for the "reverseIntegers" function. The signature we used passes an array of integers instead of an integer pointer. The main difference between the two appers when using the "sizeof" function on a pointer.
A pointer is always the same size (it only stores a memory address), but an array variable will store the individual elements. The two have very close ties and are occasionally used interchangably because arrays and pointers access individual elements the same way. However, you should make sure you understand the difference or you will frequently run into segmentation faults.
For this section, we are going to take a different approach to iterating through an array. We are going to write two functions "findChar" and "nextChar".
nextChar - Takes in a char pointer and increments it one char sized space in memory
findChar - Using nextChar, increment through the given list until the char stored in toFind is found, then return the matching chars memory address. If no match is found, return NULL
%%file src/incrementPointer.c
#include "lab3.h"
char *findChar(char *string, char toFind) {
}
/* Increment pointer */ void nextChar(char **position) {
}
%%file src/main.c
#include "lab3.h"
/* Test as whatever you would like*/ int main(int argc, char *argv[]) { char *str = "1234567"; char *pos; printf("Hello, World "); printf("Original - >"); printf("%s ", str); pos = findChar(str, '5'); /* Could easily automate this test using strcmp */ printf("pos (Should print 567) - >"); printf("%s ", pos); return 0; }
4. Command Line Aguments (4 marks) It's often beneficial to write programs that can dynamically process data without changing the code every time. Imagine only being able to open a single file or visit a single website (although I'm sure it feels like you only use courselink anyways). A useful tool for doing this is command line arguments.
Command line arguments are passed to a program when it is run after compiling. Arguments are commonly used to tell a program which flags to activate, or information about the files read/written to.
Instruction: In this secion we are going to read command line arguments from the terminal, convert them all to integers then sort them from least to greatest.
%%file src/commandLineArguments.c
#include "lab3.h"
/* Use the swapIntegers function you already coded */ void sortIntegers(int value[], int length) { /* Hint: Bubble sort is the easiest sorting algorithm to understand */ }
/* Hint: atoi */ int convertToInt(char *string) {
}
int *sortArguments(int argc, char *argv[]) { /* allocate enough memory: sizeof(int) times number of integers */ int *list = malloc(sizeof(int) * (argc-1)); /*load argv values into list*/
}
%%file src/main.c
#include "lab3.h"
/* Pay attention to the boundary conditions on the loop below */ int main(int argc, char *argv[]) { int i = 0; int *result; printf("Hello, World "); /* Here is how you access the command line arguments*/ printf("Before: "); for (i = 1; i < argc; i++) { printf("%s, ", argv[i]); } printf(" "); result = sortArguments(argc, argv);
printf(" After: "); for (i = 0; i < argc-1; i++) { printf("%d, ", result[i]); } /* Memory must be freed when allocated, but only freed once */ free(result); return 0; }
%%bash gcc -Wall -pedantic -ansi -Iinclude src/*.c -o bin/runMe ./bin/runMe 0 234 345345 91 -3 12 3 19 17
5. Multi-Dimensional Arrays (2 marks) Multi-dimensional arrays are used to store data for many different reasons. For example, command line arguemts are flexible two dimensional arrays (array of an array). It's common for data to be stored in two dimensional arrays.
For this section we will print a table of integers based on the format requested by the user. For simplicity we are only going to use integers from 0 - 99. You can assume the table will not be tested with integers greater than 99 but integers from 0 - 9 must be in the format 00, 01, 02, ... etc.
Users will have the option to add a line between all columns or no columns using this character |
Format:
The table cell must be printed with a space before and after each integer -> " 11 ". If the user would like a line in the column it should print with no lines on the left or right. i.e." 01 | 17 | 33 | 27 " columnLine = 1 prints columns, anything else ignores them Print the newline character at the end of each row " " You need to following the instructions exactly when print the array or you will receive 0 marks for this section
%%file src/2dArray.c
#include "lab3.h"
/* Access arr as arr[row][column] */ void printTable(int *arr[], int columnLine, int numRows, int numColumns) {
} %%file src/main.c
#include "lab3.h"
/* Testing is done for you */ int main(int argc, char *argv[]) { int *array[30]; int temp[30][5]; int i; int j; for (i = 0; i < 30; i++) { /*int temp[5];*/ for (j = 0; j < 5; j++) { int val = (i*5)+j; temp[i][j] = val%100; } array[i] = temp[i]; } /* Without lines */ printTable(array, 0, 30, 5); /* With lines */ printTable(array, 1, 30, 5);
return 0; }
%%bash gcc -Wall -pedantic -ansi -Iinclude src/*.c -o bin/runMe ./bin/runMe
Step by Step Solution
There are 3 Steps involved in it
Step: 1
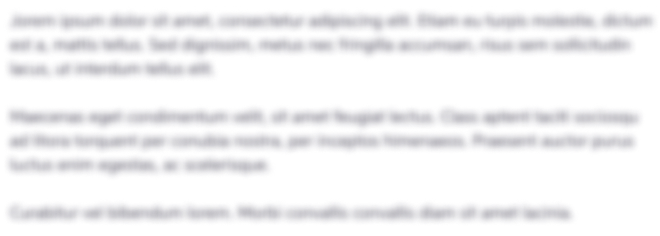
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started