Question
This array list stores the values for each calculation that the user makes. When the application ends, it prints a summary of those calculations to
This array list stores the values for each calculation that the user makes. When the application ends, it prints a summary of those calculations to the console. This summary should that looks something like this:
Future Value Calculations Inv
/Mo Rate Years Future value
$100.00 8.0% 10 $18,416.57
$125.00 8.0% 10 $23,020.71
$150.00 8.0% 10 $27, 624.85
1. Import the project named Future Value in the ex starts folder. Then, review the code for this application and run it to make sure it works correctly.
these are the code for the main file package murach.ui; import java.text.NumberFormat; import murach.calculators.Financial; public class Main { public static void main(String[] args) { // displayLine a welcome message Console.displayLine("Welcome to the Future Value Calculator"); Console.displayLine(); String choice = "y"; while (choice.equalsIgnoreCase("y")) { // get input from user double monthlyInvestment = Console.getDouble("Enter monthly investment: "); double yearlyInterestRate = Console.getDouble("Enter yearly interest rate: "); int years = Console.getInt("Enter number of years: "); // call the future value method double futureValue = Financial.calculateFutureValue( monthlyInvestment, yearlyInterestRate, years); // format and displayLine the result Console.displayLine("Future value: " + NumberFormat.getCurrencyInstance().format(futureValue)); Console.displayLine(); // see if the user wants to continue choice = Console.getString("Continue? (y/n): "); Console.displayLine(); } Console.displayLine("Bye!"); } }
/*FINANCIAL*/
package murach.calculators;
public class Financial {
public static double calculateFutureValue(double monthlyInvestment, double yearlyInterestRate, int years) {
// convert yearly values to monthly values double monthlyInterestRate = yearlyInterestRate / 12 / 100; int months = years * 12; // calculate the future value double futureValue = 0; for (int i = 1; i <= months; i++) { futureValue += monthlyInvestment; double monthlyInterestAmount = futureValue * monthlyInterestRate; futureValue += monthlyInterestAmount; } return futureValue; } }
/*CONSOLE*/
package murach.ui;
import java.util.Scanner;
public class Console {
private static Scanner sc = new Scanner(System.in); public static void displayLine() { System.out.println(); }
public static void displayLine(String s) { System.out.println(s); }
public static String getString(String prompt) { System.out.print(prompt); String s = sc.nextLine(); return s; }
public static int getInt(String prompt) { int i = 0; while (true) { System.out.print(prompt); try { i = Integer.parseInt(sc.nextLine()); break; } catch (NumberFormatException e) { System.out.println("Error! Invalid integer. Try again."); } } return i; }
public static double getDouble(String prompt) { double d = 0; while (true) { System.out.print(prompt); try { d = Double.parseDouble(sc.nextLine()); break; } catch (NumberFormatException e) { System.out.println("Error! Invalid decimal. Try again."); } } return d; } }
2. Declare a variable at the beginning of the main method for an array list that Stores strings.
3. After the code that calculates, formats, and displays the results for each calculation, add code that formats a string with the results of the calculation and then stores the string in the array list.
4. Add code to print the elements in the array list to the console when the user exits the application. Then, test the application by making at least 3 future value calculations.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
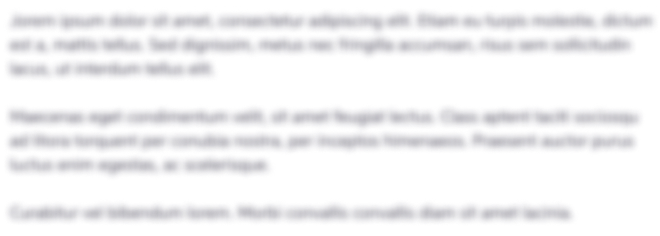
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started