Question
This assignment adds to the BankAccount hierarchy from Python for Everyone 3rd edition - How to 10.1. The following classes have been provided as per
This assignment adds to the BankAccount hierarchy from Python for Everyone 3rd edition - How to 10.1. The following classes have been provided as per the source code of the text:
-
BankAccount
-
SavingsAccount
-
CheckingAccount
The tester is also provided.
In this exercise, you need to complete all of the TODO items, which include
-
__repr__ for BankAccount, SavingsAccount, CheckingAccount such that it displays the class name (__class__.__name__) followed by the instance variables for that class in the format [variable=value]. Note that the __repr__ for BankAccount will display the class name and the subclasses will concatenate to its superclasses __repr__ its own instance variables.
-
You need to write the code for the TimeDepositAccount class, based on the UML diagram specification given on Canvas. A TimeDepositAccount is a savings account that you have agreed to keep the money in until it matures. If you withdraw money before its maturity, an early withdrawal penalty will be charged.
Download the completed main.py and submit on Canvas once submitted on Repl.it
Below is the expected output for the given tester:
------------------------------------------------------------------------------------------------------------------
Test with Penalty
Before withdrawal ($500): 1000.29
After withdrawal ($500): 490.28999999999996
Test without Penalty
Before withdrawal ($500): 1000.5800840999999
After withdrawal ($500): 500.5800840999999
SavingsAccount[balance=10025.0][interestRate=0.015]
CheckingAccount[balance=125.0][withdrawals=0]
TimeDepositAccount[balance=515.29][interestRate=0.029][monthsToMaturity=1]
TimeDepositAccount[balance=525.5800840999999][interestRate=0.029] [monthsToMaturity=0]
## # This module defines several classes that implement a banking account # class hierarchy. #
## A bank account has a balance and a mechanism for applying interest or fees at # the end of the month. # class BankAccount : ## Constructs a bank account with zero balance. # def __init__(self) : self._balance = 0.0
## Makes a deposit into this account. # @param amount the amount of the deposit # def deposit(self, amount) : self._balance = self._balance + amount ## Makes a withdrawal from this account, or charges a penalty if # sufficient funds are not available. # @param amount the amount of the withdrawal # def withdraw(self, amount) : self._balance = self._balance - amount ## Carries out the end of month processing that is appropriate # for this account. # def monthEnd(self) : return ## Gets the current balance of this bank account. # @return the current balance # def getBalance(self) : return self._balance
## TODO: __repr__ ## A savings account earns interest on the minimum balance. # class SavingsAccount(BankAccount) : ## Constructs a savings account with a zero balance. # def __init__(self) : super().__init__() self._interestRate = 0.0 self._minBalance = 0.0
## Sets the interest rate for this account. # @param rate the monthly interest rate in percent # def setInterestRate(self, rate) : self._interestRate = rate
## Overrides superclass method. # def withdraw(self, amount) : super().withdraw(amount) balance = self.getBalance() if balance < self._minBalance : self._minBalance = balance
## Overrides superclass method. # def monthEnd(self) : interest = self._minBalance * self._interestRate / 100 self.deposit(interest) self._minBalance = self.getBalance()
## TODO: __repr__
## A checking account has a limited number of free deposits and withdrawals. # class CheckingAccount(BankAccount) : ## Constructs a checking account with a zero balance. # def __init__(self) : super().__init__() self._withdrawals = 0
## Overrides superclass method. # def withdraw(self, amount) : FREE_WITHDRAWALS = 3 WITHDRAWAL_FEE = 1 super().withdraw(amount) self._withdrawals = self._withdrawals + 1 if self._withdrawals > FREE_WITHDRAWALS : super().withdraw(WITHDRAWAL_FEE)
## Overrides superclass method. # def monthEnd(self) : self._withdrawals = 0
## TODO: __repr__
## TODO: TimeDepositAccount class
# ACO 240 Using SavingsAccount and CheckingAccount for How To 10.1 Python for Everyone # Tester code mySavings = SavingsAccount() mySavings.deposit(10000) mySavings.setInterestRate(.015)
myChecking = CheckingAccount() myChecking.deposit(100)
print("Test with Penalty") tda1 = TimeDepositAccount() tda1.deposit(1000) tda1.setInterestRate(.029) tda1.setMonthsToMaturity(3) tda1.monthEnd() # month 1 tda1.monthEnd() # month 2 print("Before withdrawal ($500): ", tda1.getBalance()) tda1.withdraw(500) print("After withdrawal ($500): ", tda1.getBalance())
print("Test without Penalty") tda2 = TimeDepositAccount() tda2.deposit(1000) tda2.setInterestRate(.029) tda2.setMonthsToMaturity(3) tda2.monthEnd() # month 1 tda2.monthEnd() # month 2 tda2.monthEnd() # month 2 print("Before withdrawal ($500): ", tda2.getBalance()) tda2.withdraw(500) print("After withdrawal ($500): ", tda2.getBalance())
# Quarterly Bonus: For all accounts, give customer $25 bonus and display accounts
accts = [mySavings, myChecking, tda1, tda2] for a in accts: a.deposit(25) print(a)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
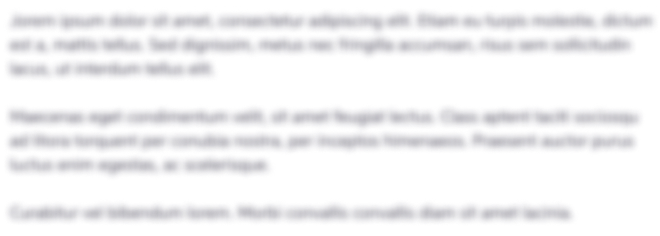
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started