Question
This assignment handles object oriented programming: Write a main class named myNumberList which has the following functions: - constructor: has an optional argument that will
This assignment handles object oriented programming: Write a main class named myNumberList which has the following functions: - constructor: has an optional argument that will be added to the list - add(value): will insert it at the end of the list - remove(value): will remove the item from the list (if item is there multiple times, it removes all occurrences. - head(): returns the value in the beginning of the list - getList(): will return the list - if I call print(myNumberList) the list should be printed
Write a subclass named myRevOrderedNumberList (from high to low) which has overwrites - head() function and returns the highest value and - overwrites the print functionality to be printed appropriately - getList(): will return the list
Some general notes: - your lists do only handle numbers (float + int); if a function receives anything else, print a warning and ignore it. - if variables are used, they should be all private
some test are below:
myL = myNumberList() myL.add(15) myL.add(20) myL.add(1) print(myL.head()) #15 print(myL) #[15,20,1] myL.add("5") #Only numbers can be added. print(myL) #[15,20,1] myL.add(15) print(myL) #[15,20,1,15]
myL2 = myRevOrderedNumberList() myL2.add(15) myL2.add(20) myL2.add(1) myL.add("hello") #Only numbers can be added. print(myL2) #[20, 15, 1] print(myL2.head()) #20
code needs Python V3.x
Step by Step Solution
There are 3 Steps involved in it
Step: 1
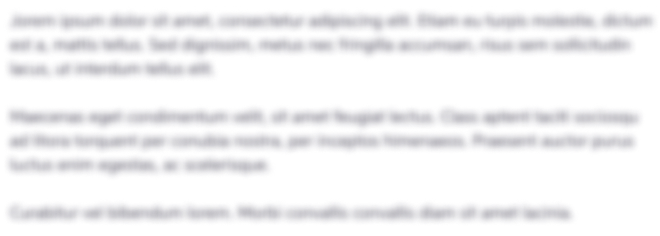
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started