Question
This assignment is in C++ Please post code This is the starter code #include #include #include #include using namespace std; class Item { public: void
This assignment is in C++
Please post code
This is the starter code
#include
Pointers/Inheritance - Inventory system
In this assignment, youll make an inventory system for a stores items, including produce and
books. The starter program is an inventory system for only produce.
1. Include the price of an item by adding to the Item class the protected data member int
priceInDollars that stores the price in dollars of an individual item.
Write a public function SetPrice with a single int parameter prcInDllrs and returns nothing.
SetPrice assigns the value of prcInDllrs to priceInDollars.
Modify the AddItemToInventory to prompt the user to Enter the price per item: $, then set the
Produces price to the userentered value.
Modify Produces Print function output to include the items individual price. Here is an example
output for 20 grape bundles at $3 each that expire on May 22.
Grape bundle x20 for $3 (Expires: May 22)
2. Add a new class Book that derives from Item. The Book class has a private data member that
is a string named author. The Book class has a public function member SetAuthor with the
parameter string authr that sets the private data member authors value. SetAuthor returns
nothing.
The class Book overloads the Print member function with a similar output as the Produces Print
function, except Expires is replaced with Author and the variable expiration is replaced with
author. Here is an example output for 22 copies of
To Kill a Mockingbird
by Harper Lee that
sells for $5 each:
To Kill a Mockingbird x22 for $5 (Author: Harper Lee)
3. Modify the AddItemToInventory function to allow the user to choose between adding a book (b)
and produce (p). If the user does not enter b or p, then output Invalid choice then return the
AddItemToInventory function.
4. Create a class named Inventory. The Inventory class should have one private data member:
vector
parameters and return nothing: PrintInventory, AddItemToInventory, UpdateItemQtyInInventory,
and RemoveItemFromInventory.
Development suggestion: First convert only the PrintInventory function to be a member function
of Inventory with the other functions disabled (commented out). Then, convert the other functions
one by one.
5. Add to the Inventory class a private data member int totalInvPriceInDollars that stores the total
inventory price in dollars.
Write a private member function SumInv in Inventory class that has no parameters and returns
nothing. The SumInv function should set the total inventory value as a price in dollars. Since the
Inventory class cannot access an Items quantity and priceInDollars, youll need to write a public
member function GetTotalValueAsPrice to the Item class that returns the multiplication of the
Items quantity and priceInDollars.
The SumInv function should be called at the end (but before the return statement) of each
Inventory member function that modifies the inventory: AddItemToInventory,
UpdateItemQtyInInventory, and RemoveItemFromInventory.
Modify the PrintInventory function to also output the total inventory value in dollars. Here is an
example:
0 Grapes x2 for $3 (Expires: May)
1 To Kill a Mockingbird x4 for $5 (Author: Harper Lee)
Total inventory value: $26
Here is an example program execution (user input is highlighted here for clarity):
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
a
Enter choice of adding (b)ook or (p)roduce:
p
Enter name of new produce:
Grape bundle
Enter quantity:
20
Enter expiration date:
May 12
Enter the price per item: $
2
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
p
0 - Grape bundle x20 for $2 (Expires: May 12)
Total inventory value: $40
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
a
Enter choice of adding (b)ook or (p)roduce:
b
Enter name of new book:
Illiad
Enter quantity:
10
Enter author:
Homer
Enter the price per item: $
5
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
p
0 - Grape bundle x20 for $2 (Expires: May 12)
1 - Illiad x10 for $5 (Author: Homer)
Total inventory value: $90
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
a
Enter choice of adding (b)ook or (p)roduce:
b
Enter name of new book:
To Kill a Mockingbird
Enter quantity:
2
Enter author:
Harper Lee
Enter the price per item: $
10
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
u
0 - Grape bundle x20 for $2 (Expires: May 12)
1 - Illiad x10 for $5 (Author: Homer)
2 - To Kill a Mockingbird x2 for $10 (Author: Harper Lee)
Total inventory value: $110
Update which item #:
2
Enter new quantity:
25
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
p
0 - Grape bundle x20 for $2 (Expires: May 12)
1 - Illiad x10 for $5 (Author: Homer)
2 - To Kill a Mockingbird x25 for $10 (Author: Harper Lee)
Total inventory value: $340
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
r
0 - Grape bundle x20 for $2 (Expires: May 12)
1 - Illiad x10 for $5 (Author: Homer)
2 - To Kill a Mockingbird x25 for $10 (Author: Harper Lee)
Total inventory value: $340
Remove which item #:
1
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
p
0 - Grape bundle x20 for $2 (Expires: May 12)
1 - To Kill a Mockingbird x25 for $10 (Author: Harper Lee)
Total inventory value: $290
Enter (p)rint, (a)dd, (u)pdate, (r)emove, or (q)uit:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
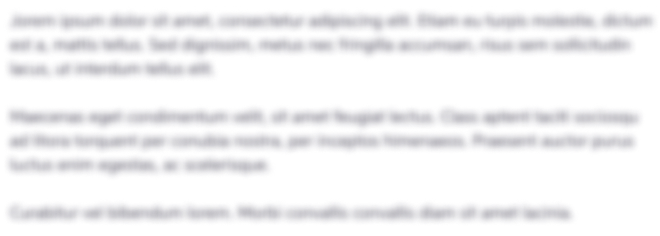
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started