Question
This assignment is so confusing. Repl.it is terrible. Please help with explanation: Your task is to complete the implementation of the insertion function (insert_into_array) outlined
This assignment is so confusing. Repl.it is terrible. Please help with explanation:
Your task is to complete the implementation of the insertion function (insert_into_array) outlined to the left.
The insertion function inserts a value into an array at a specified position. All elements to the right of the inserted element are shifted over a position (upon inserting, all elements at the insertion position are shifted up an index, and the last element in the array is overwritten by the previous element).
// ----------------------------------------------------------------- // START DO NOT MODIFY // -----------------------------------------------------------------
#include
// notice the use of size_t, this prevents bugs as indices and array // lengths cannot be negative (and there we don't have to handle nasty // edge cases were positions/lengths are negative when they shouldn't be)! int* insert_into_array(int arr[], size_t arr_len, int value, size_t pos);
// helper functions void print_arr(int arr[], size_t arr_len); void run_test_case(int test_case);
int main() { int test_case; printf("Enter test_case: "); scanf("%d", &test_case); run_test_case(test_case); }
// ----------------------------------------------------------------- // END DO NOT MODIFY // -----------------------------------------------------------------
int* insert_into_array(int arr[], size_t arr_len, int value, size_t pos) { }
// ----------------------------------------------------------------- // START DO NOT MODIFY // ----------------------------------------------------------------- // helper function to print the contents of an array, you should NOT // call this directly, the test cases will call it void print_arr(int arr[], size_t arr_len) { for (int i = 0; i < arr_len; i++) { printf("%d ", arr[i]); } printf(" "); }
// runs test cases void run_test_case(int test_case) { // we use if statements here (instead of a switch) because a switch statement // has a single scope, meaning we can't define different versions of arr for // each case if (test_case == 0) { int arr[] = {3, 4, 5, 6}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, 2); printf("After insertion: "); print_arr(result, arr_len); } else if (test_case == 1) { int arr[] = {3, 4, 5, 6, 86, 10, 123, 134}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, 2); printf("After insertion: "); print_arr(result, arr_len); } else if (test_case == 2) { int arr[] = {3, 4, 5, 6, 86, 10, 123, 134}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, 0); printf("After insertion: "); print_arr(result, arr_len); } else if (test_case == 3) { int arr[] = {3, 4, 5, 6, 86, 10, 123, 134}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, arr_len-1); printf("After insertion: "); print_arr(result, arr_len); } else if (test_case == 4) { int arr[] = {3, 4, 5, 6, 86, 10, 123, 134}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, 15); printf("After insertion: "); print_arr(result, arr_len); } else if (test_case == 5) { int arr[] = {3, 4, 5, 6, 86, 10, 123, 134}; size_t arr_len = sizeof(arr) / sizeof(int); printf("Before insertion: "); print_arr(arr, arr_len); int* result = insert_into_array(arr, arr_len, 6, -10); printf("After insertion: "); print_arr(result, arr_len); } } // ----------------------------------------------------------------- // END DO NOT MODIFY // -----------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
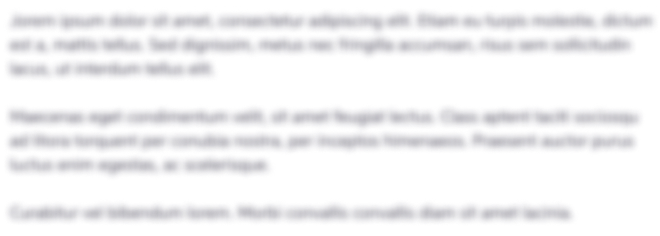
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started