Question
This assignment requires the writing of a program that simulates the operations of a bank, from the perspective of one client who has several different
This assignment requires the writing of a program that simulates the operations of a bank, from the perspective of one client who has several different types of bank accounts. An object will be created for each type of account, then transactions will be applied to the accounts and the accounts will be output in various orders to both the screen and a file.
HAND IN: Submit the final code that you produced (in your ClientUpdate.java file).
1. Examine & add comments to the Bank Account class:
The following code defines a very simple class called BankAccount. Observe that the class contains the data associated with only one account, and the core functionality (methods) that the account needs. More specifically, it contains 4 data items (or attributes) and a collection of instance methods (or behaviours) for changing the values of that data.
/* ADD COMMENTS TO THIS CLASS, DO NOT CHANGE ANY CODE! */ import java.util.*; public class BankAccount { private int accountNumber; private String ownerName; private double balance; private String type; // Personal, Business, Charitable public BankAccount() { accountNumber = 0; ownerName = ""; balance = 0.0; type = "Personal"; } public BankAccount(int number, String name, double initialDeposit, String type) { accountNumber = number; ownerName = name; balance = initialDeposit; this.type = type; // Why does 'this' need to be used here?? } public int getAccountNumber() { return accountNumber; } public void setAccountNumber(int number) { accountNumber = number; } public String getOwnerName() { return ownerName; } public void setOwnerName(String name) { ownerName = name; } public double getBalance() { return balance; } public void setBalance(double newAmount) { balance = newAmount; } public String getType() { return type; } public void deposit(double amount) { balance += amount; |
} public void withdrawl(double amount) { balance -= amount; } public String toString() { return type + ": " + accountNumber + " " + ownerName + " " + balance; } } |
Use this code but do not alter it: enhance the documentation by including: For each method add a precise description of its purpose, input and output. Explain the purpose of each member variable. 2. The Bank: A program that uses the BankAccount class: In a different Java class, called ClientUpdate.java, create a banks client processing program with the following specifications: The single clients file contains information on all that persons accounts (personal, business and charitable), including deposits, withdrawals, interest earned and charges. These are all stored in a text file, the name of the file should be input from the keyboard. - The first line of the file contains an integer, called number, indicating the number of accounts and the clients name. - The next number lines of the file contain, for each account, a String token indicating the type of the account and a unique integer representing the account number and a real number indicating the starting balance. An array of BankAccounts can be created to hold the client file information. Use a constructor from the BankAccount class to instantiate each of the accounts.
- | Following that, there are an unknown number of lines, each contains the integer identification number for the account, a String (one of deposit, withdrawal, interest, charge) indicating the type of transaction, and a real number indicating the amount of the transaction. |
The amount of a deposit must be added to the BankAccounts balance; The amount of a withdrawl must be subtracted from the balance; The amount of interest requires adding amount % interest to the account; The amount of a (service) charge must be subtracted from the account. |
Use member methods of the BankAccount class to alter the attributes of the appropriate BankAccount object. Once the entire file has been processed output all attributes of each account to the screen: - Use the instance method called toString()to make a single String containing all attributes of a BankAccount object that is being printed. HAND IN: Submit the final code that you produced (in your ClientUpdate.java file)
Sample output if the SampleTransactions.dat file was used: T R A N S A C T I O N U P D A T I N G Input: Name of Client Information File ==> SampleTransactions.dat Jones,John Personal: 32145 Jones,John 3584.57 Business: 34668 Jones,John 146.52 Business: 34669 Jones,John 22537.42 Charitable: 21356 Jones,John 5424.65
Account Summary:
Personal: 32145 Jones,John 3525.5074400000008 Business: 34668 Jones,John -1438.7100000000003 Business: 34669 Jones,John 22843.559549999998 Charitable: 21356 Jones,John 5849.208134664999
In order to show the exact spacing on the output lines, each space is replaced with an @ symbol, each tabl with a \t and each blank line with a below: T@R@A@N@S@A@C@T@I@O@N@@@U@P@D@A@T@I@N@G Input:@ @@Name@of@Client@Information@File@==>@SampleTransactions.dat Jones,John Personal:@32145@Jones,John@3584.57 Business:@34668@Jones,John@146.52 Business:@34669@Jones,John@22537.42 Charitable:@21356@Jones,John@5424.65 Account@Summary:@ Personal:@32145@Jones,John@3525.5074400000008 Business:@34668@Jones,John@-1438.7100000000003 Business:@34669@Jones,John@22843.559549999998 Charitable:@21356@Jones,John@5849.208134664999
Step by Step Solution
There are 3 Steps involved in it
Step: 1
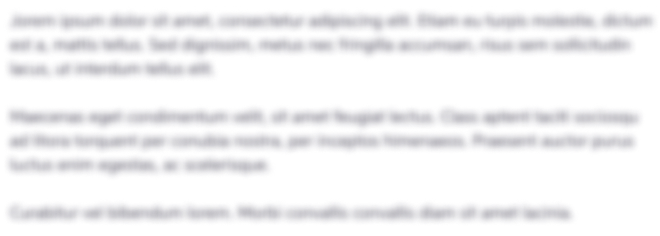
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started