Question
This assignment will give you practice with defining classes. You are to write a set of classes that define the behavior of certain animals. You
This assignment will give you practice with defining classes. You are to write a set of classes that define the behavior of certain animals. You will be given a program that runs a simulation of a world with many animals wandering around in it. Different kinds of animals will behave in different ways and you are defining those differences. In this world, animals propagate their species by infecting other animals with their DNA, which transforms the other animal into the infecting animals species.
INSTRUCTIONS
For this assignment you will be given a lot of supporting code that runs the simulation. You are just defining the individual critters that wander around this world trying to infect each other. While it is running the simulation will look something like this:
n order to get started with this assignment, start by downloading the CS145 Assignment 2 ZIP file from Canvas. Inside that zip file is a folder called JavaCritter that contains 8 files. Move the folder and all 8 files into your data drive.
Of the 8 files included.
File | Actions |
CritterFrame.java | Do not touch this file |
CriterInfo.java | Do not touch this file |
CritterModel.java | Do not touch this file |
CritterPanel.java | Do not touch this file |
Critter.java | Open this file to look at it, but do not change it. |
Food.java | Open this file to look at it, but do not change it. Use this as an example for your files. |
Flytrap.java | Open this file to look at it, but do not change it. Use this as an example for your files. |
CritterMain.java | This is the file that you will run. You can make very minimal changes to this file to add more critters to the simulation. |
You objective is to implement the following 4 classes that are subclasses of critter. You will create 4 new classes and add them to the simulation.
Animal class
Each of your critter classes should extend a class known as Critter. So each Critter class will look like this:
public class SomeCritter extends Critter { ...
}
The extends clause in the header of this class establishes a polymorphic inheritance relationship as discussed in class. The main point to understand is that the Critter class has several methods and constants defined for you.
On each round of the simulation, each critter is asked what action it wants to perform. There are four possible responses each with a constant associated with it.
Constant | Description |
Action.HOP | Move forward one square in its current direction |
Action.LEFT | Turn left (rotate 90 degrees counter-clockwise) |
Action.RIGHT | Turn right (rotate 90 degrees clockwise) |
Action.INFECT | Infect the critter in front of you |
There are three key methods in the Critter class that you will override in your own classes. When you override these methods, you must use exactly the same method header as what you see below. The three methods to override for each Critter class are:
public Action getMove(CritterInfo info) { ... } public Color getColor() {
... } public String toString() { ... }
There are three key methods in the Critter class that you will override in your own classes, however you may want to implement additional methods to implement your own class actions.
For the getMove method you should return one of the four Action constants described earlier in the writeup. For the getColor method, you should return whatever color you want the simulator to use when drawing your critter. And for the toString method, you should return whatever text you want the simulator to use when
displaying your critter (normally a single character).
For example, below is a defnition for a critter that always infects and that displays itself as a green letter F:
import java.awt.*;
public class Food extends Critter { public Action getMove(CritterInfo info) {
return Action.INFECT; }
public Color getColor() { return Color.GREEN;
}
public String toString() { return "F";
} }
Notice that it begins with an import declaration to be able to access the Color class. All of your Critter classes will have the basic form shown above.
The getMove method is passed an object of type CritterInfo. This is an object that provides you information about the current status of the critter. It includes eight methods for asking about surrounding neighbors plus a method to find out the current direction the critter is facing. Below are the methods of the CritterInfo class:
CritterInfo Method | Description |
public Neighbor getFront() | returns neighbor in front of you |
public Neighbor getBack() | returns neighbor in back of you |
public Neighbor getLeft() | returns neighbor to your left |
public Neighbor getRight() | returns neighbor to your right |
public Direction getDirection() | returns direction you are facing |
public Direction getFrontDirection() | returns the direction of the neighbor in front of you |
public Direction getBackDirection() | returns the direction of the neighbor in back of you |
public Direction getLeftDirection() | returns the direction of the neighbor to your left |
public Direction getRightDirection() | returns the direction of the neighbor to your right |
The getDirection method tells you what direction you are facing (one of four direction constants):
The return type for the frst group of four CritterInfo methods is Neighbor. There are four different constants for the different kind of neighbors you might encounter:
Constant | Description |
Direction.NORTH | facing north |
Direction.SOUTH | facing south |
Direction.EAST | facing east |
Direction.WEST | facing west |
Constant | Description |
Neighbor.WALL | The neighbor in that direction is a wall |
Neighbor.EMPTY | The neighbor in that direction an empty square |
Neighbor.SAME | The neighbor in that direction is a critter of your species |
Neighbor.OTHER | The neighbor in that direction is a critter of another species |
Notice that you are only told whether critters are of your species or some other species. You cant find out exactly what species they are. The second group of four methods tells you what direction the neighbors on each
This program will probably be confusing at first because this time you are not writing the main method. Your code will not be in control. Instead, you are defining a series of objects that become part of a larger system. For example, you might find that you want to have one of your critters make several moves all at once. You wont be able to do that. The only way a critter can move is to wait for the simulator to ask it for a move. The simulator is in control, not your critters. Although this experience can be frustrating, it is a good introduction to the kind of programming we do with objects. A typical Java program involves many different interacting objects that are each a small part of a much larger system.
Critters move around in a world of finite size that is enclosed on all four sides by walls. You can include a constructor for your classes if you want, although it should generally be a zero-argument constructor (one that takes no arguments). The one exception is that you are to define a class called Bear that takes a Boolean parameter specifying whether it is a black bear or a polar bear, which will change how its color is displayed. The simulator treats bears in a special way, basically flipping a coin each time it constructs one to decide whether or not to create a white bear or black bear. Your Bear class does not have to fgure this out. You just have to make sure that each bear pays attention to the boolean value passed to the constructor by the simulator.
YOU ARE TO IMPLEMENT FOUR CLASSES.
BEAR
Constructor o public Bear(boolean polar)
getColor o Color.WHITE for a polar bear
(when polar is true), o Color.BLACK otherwise
(when polar is false) toString
o Should alternate on each different move between a slash character (/) and a backslash character (\) starting with a slash.
CSE142 critter simulation X fee L Bear - 30 fee fedT F T F F F L Fly Trap - 30 T fes fee Food - 30 fee T fee F Giant - 30 fee L fes fee L F Lion - 30 fee T/ fee fee fee F T fee L T fea L fece step = 0 F fee slow fast start stop step debug next 100 CSE142 critter simulation X fee L Bear - 30 fee fedT F T F F F L Fly Trap - 30 T fes fee Food - 30 fee T fee F Giant - 30 fee L fes fee L F Lion - 30 fee T/ fee fee fee F T fee L T fea L fece step = 0 F fee slow fast start stop step debug next 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
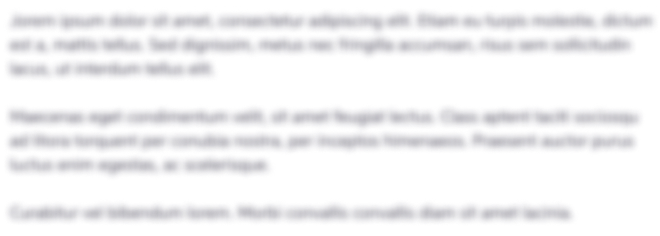
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started