Question
This challenges you to complete the SymbolGraph class which implements the generic Graph interface. The test cases use String as the generic data type, but
This challenges you to complete the SymbolGraph class which implements the generic Graph interface. The test cases use String as the generic data type, but in theory it is quite flexible.
package graphs;
import java.util.Collection;
public interface Graph
{
/**
* @return the number of vertices in the graph
*/
int getNumberOfVerticies();
/**
* @return the number of edges in the graph
*/
int getNumberOfEdges();
/**
* Adds the specified undirected edge to the graph
* @param from the start vertex
* @param to the end vertex
* @return true if the vertex did not already exist
*/
boolean addEdge(E from, E to);
/**
* Get all vertices adjacent to the specified vertex
* @param to the specified vertex
* @return a Collection of all adjacent vertices
*/
Collection
/**
* Removes the edge both from and to
* @param from the start vertex
* @param to the end vertex
* @return true if an edge was removed
*/
boolean removeEdge(E from, E to);
}
package graphs;
import java.util.ArrayList; import java.util.Collection;
public class ArrayGraph implements IndexedGraph { private int vertex; private int edge; private Collection[] edges; @SuppressWarnings("unchecked") public ArrayGraph(int veritcies) { }
@Override public int getNumberOfVerticies() { return 0; }
@Override public int getNumberOfEdges() { return 0; }
@Override public boolean addEdge(Integer from, Integer to) { return false; }
@Override public Collection getAdjacent(Integer to) { return new ArrayList(); }
@Override public boolean removeEdge(Integer from, Integer to) { return false; } }
// Test unit
package graphs.test;
import static org.junit.Assert.assertArrayEquals; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue;
import java.util.ArrayList; import java.util.Collection;
import org.junit.Test;
import graphs.ArrayGraph; import graphs.IndexedGraph;
public class GraphTest { private static final int SIZE = 100; @Test @GradedTest(name="Test Graph(), getNumberOfVerticies()", max_score=10) public void testInit() { IndexedGraph uut = new ArrayGraph(SIZE); assertEquals("The size of the created Graph does not match", SIZE, uut.getNumberOfVerticies()); assertEquals("The new Graph has edges before adding", 0, uut.getNumberOfEdges()); } @Test @GradedTest(name="Test add(), getAdjacent(), getNumberOfEdges()", max_score=5) public void testAdd() { int bonusTest = SIZE/4; testInit(); IndexedGraph uut = new ArrayGraph(SIZE); Collection expected = new ArrayList(); assertArrayEquals("Your graph reported edges at vertex 0 before any were added", expected.toArray(), uut.getAdjacent(0).toArray()); for (int i = SIZE - 1; i > SIZE / 2; i--) { expected.add(i); assertTrue("Your graph did not add the expected value " + i, uut.addEdge(0, i)); assertTrue("Your graph did not add the expected value " + i, uut.addEdge(bonusTest, i)); } assertArrayEquals("Your graph did not save the expected edges to vertex 0", expected.toArray(), uut.getAdjacent(0).toArray()); assertArrayEquals("Your graph did not save the expected edges to vertex " + bonusTest, expected.toArray(), uut.getAdjacent(bonusTest).toArray()); assertEquals("The Graph reported the wrong size", expected.size() * 2, uut.getNumberOfEdges()); }
@Test @GradedTest(name="Test remove()", max_score=5) public void testRemove() { testAdd(); IndexedGraph uut = new ArrayGraph(SIZE); Collection expected = new ArrayList(); for (int i = SIZE - 1; i > SIZE / 2; i--) { expected.add(i); uut.addEdge(0, i); }
for (int i = SIZE - 1; i > SIZE / 2; i--) { expected.remove(i); uut.removeEdge(0, i); assertEquals("The number of edges did nto match expected", expected.size(), uut.getNumberOfEdges()); assertArrayEquals("The vertex 0 should did not match expected", expected.toArray(), uut.getAdjacent(0).toArray()); } }
@Test @GradedTest(name="Test Exceptional Cases", max_score=5) public void testExceptions() { testRemove(); IndexedGraph uut = new ArrayGraph(SIZE); assertFalse("Your code reported you added at " + SIZE + " which is greater than the size", uut.addEdge(0, SIZE)); assertFalse("Your code reported you added at -1 which is nonsensical", uut.addEdge(0, -1)); assertFalse("Your code reported it removed an edge that was not in the edges", uut.removeEdge(0, 1)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
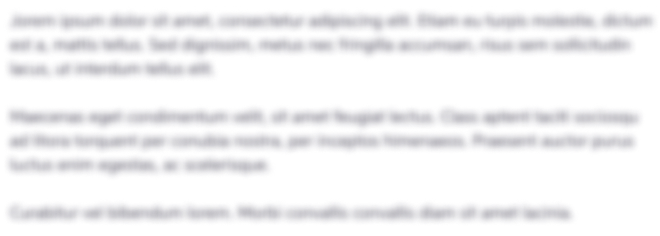
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started