Question
//This class is Matrix //my question in MatrixOperation class public class Matrix { private int nRow, nCol; private double[] data; // must store as linear
//This class is Matrix
//my question in MatrixOperation class
public class Matrix
{
private int nRow, nCol;
private double[] data; // must store as linear array unless 0
//getter for NRow - do not modify
public int getNRow()
{
return nRow;
}
//getter for NCol - do not modify
public int getNCol()
{
return nCol;
}
/**
* set nRow to r if r is not negative, otherwise set nRow to 0
* @param r: value to be assigned to nRow
*/
public void setNRow(int r)
{
if(r
nRow = 0;
else
nRow = r;//to be completed
}
/**
* set nCol to c if c is not negative, otherwise set nCol to 0
* @param c: value to be assigned to nCol
*/
public void setNCol(int c)
{
if(c
nCol = 0;
else
nCol = c;//to be completed
}
/**
*
* @return true if both nRow and nCol are zero, false otherwise
*/
public boolean isEmpty()
{
if(nRow == 0 && nCol ==0)
return true;
else
return false; //to be completed
}
/**
* DO NOT MODIFY
* if arr is null, instance array data should become null,
* otherwise if arr.length is not equal to nRow * nCol,
* set nRow, nCol to 0 and data to null,
* otherwise instantiate instance array data to be of the
* same size as arr. then copy each item of arr into data.
* IMPORTANT: do not re-declare data!
*
* @param arr
*/
public void setData(double[] arr) {
if(arr == null)
data = null;
else {
if(nRow*nCol != arr.length) {
nRow = 0;
nCol = 0;
data = null;
return;
}
data = new double[arr.length];
for(int i=0; i
data[i] = arr[i];
}
}
}
/**
* Default constructor.
* instance variables nRow and nCol should be set to 0 using the setters.
* the data member data should be set to null
*/
public Matrix()
{
setNRow(0);
setNCol(0);
data = null;//to be completed
}
/**
* Constructor a matrix which has r rows and c columns.
* data member nRow and nCol should be set accordingly using setters.
* AFTER THAT, instance array data should be instantiated to size nRow*nCol
* @param r: number of rows
* @param c: number of columns
*/
public Matrix(int r, int c)
{
setNRow(r);
setNCol(c);
data = new double [r*c];//to be completed
}
/**
*
* @return true if the matrix is a square matrix (a matrix
* for which number of rows and number of columns is the same)
*/
public boolean isSquare() {
if(nRow == nCol)
return true;
return false; //to be completed
}
/**
*
* @param other
* @return true if calling object and parameter object have the same
* dimensions (they both have the same number of rows compared to each
* other, and the same number of columns compared to each other), false otherwise
*/
public boolean sameDimensions(Matrix other)
{
if(nRow == other.nRow)
if(nCol == other.nCol)
return true;
return false; //to be completed
}
/**
* @param r
* @return true if r is a valid row number, false otherwise
* only row numbers 0 to nRow-1 (inclusive on both sides) are valid
*/
public boolean isValidRowNumber(int r) {
if(r >= 0 && r return true; } return false; //to be completed } /** * @param c * @return true if c is a valid column number, false otherwise * only column numbers 0 to nCol-1 (inclusive on both sides) are valid */ public boolean isValidColumnNumber(int c) { if(c >= 0 && c return true; } return false; //to be completed } /** * The constructor must first check that r * c is equal to the length of array d. * if this requirement does not meet, member nRow and nCol should be set to 0 and * data should be set to null. * if the requirement is met, nRow should be set to r and nCol should be set to c. * d should be copied into data using setData(double[] arr) method * @param r: number of rows * @param c: number of columns * @param d: array d to populate data */ public Matrix(int r, int c, double[] d) { if(r * c == d.length) { nRow = r; nCol = c; setData(d); } else{ nRow = 0; nCol = 0; data = null;//to be completed } } /** * The method must check that r and c are valid row numbers and column numbers * respectively. Note that row and column numbers begin with 0. * It should return 0 if r or c is out of range of the dimension of the matrix * @param r * @param c * @return an element of the Matrix at row r and column c */ public double get(int r, int c) { if(isValidRowNumber(r) && isValidColumnNumber(c)) { return data[r*nCol +c]; } else return 0; //to be completed } /** * setting and element to row r and column c with value v * The method must check that r and c are in the range of numberOfRow() and numberOfColumn(). * It should not do anything if r or c is out of range of the dimension of the matrix * @param r * @param c * @param v */ public void set(int r, int c, double v) { int numberOfRow = r; int numberOfColumn = c; double[][] matrix = new double[r][c]; if(r>=numberOfRow && c >=numberOfColumn) matrix[r-1][c-1] = v;//to be completed } /** * DO NOT MODDIFY */ public String toString() { String result = ""; for(int r = 0; r { result = result + "| "; for(int c = 0; c { result = result + String.format("%7.2f ", get(r, c)); } result = result + "| "; } return result; } } //MatrixOperation class public class MatrixOperations { /** * add a scalar value (constant) to a matrix. * IMPORTANT: the matrix passed should NOT be modified. * @param m: matrix to which scalar is to be added * @param b: scalar to be added * @return resulting Matrix */ public static Matrix add(Matrix m, double b) { double[][] r; r=getData(); // Matrix result = new Matrix(); for(int i=0; i for(int k=0; i r[i][k] = r[i][k] + b; } } return result; } /** * add to matrices. * IMPORTANT: neither of the passed matrices should be modified * @param m: matrix 1 * @param n: matrix 2 * @return if m and n have the same dimensions, return * result of adding the two matrices, otherwise return null */ public static Matrix add(Matrix m, Matrix n) { Matrix result = new Matrix(); return result; //to be completed }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
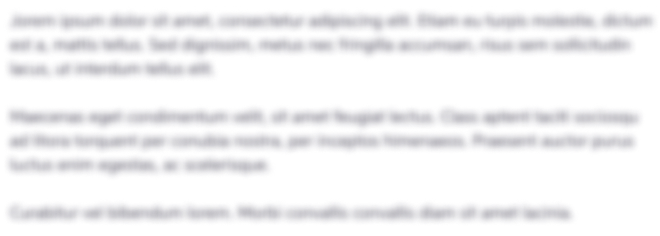
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started