Question
This code has the start of a class representing a card shoe -- the actual name of the things that casino dealers use to help
This code has the start of a class representing a "card shoe" -- the actual name of the things that casino dealers use to help shuffle and deal cards.
package edu.buffalo.cse116;
import java.util.List;
public class CardShoe {
private List cards;
public CardShoe(List originalList) {
cards = originalList;
}
public void shuffle(ListGenerator gen) {
List firstHalf = gen.createNewList();
List secondHalf = gen.createNewList();
}
}
For this problem, you will need to complete the shuffle()method by implementing the following algorithm:
/*
shuffle():
Move the first half of cards into firstHalf // This may or may not be done in one line of code
Move the remaining elements in cards into secondHalf // This may or may not be done in one line of code
While there are elements in firstHalf
Remove the element at the start of firstHalf and add it to cards
Remove the element at the start of secondHalf and add it to cards
EndWhile
If secondHalf has any elements remaining
Remove the element at the start of secondHalf and add it to cards
EndWhile
*/
The classes required to complete this code are the following:
package edu.buffalo.cse116;
import java.util.ArrayList; import java.util.LinkedList; import java.util.List; import java.util.Random;
/** * Class that can be used to get some type of list, but not a predictable type of list. This forces * students to only use the methods defined by all Lists. * @author Matthew Hertz */ public class ListGenerator {
/** * Generates an instance of list, but which type of list is unpredictable. * @return A new List instance. */ public List createNewList() { Random rnd = new Random(); if (rnd.nextBoolean()) { return new ArrayList(); } else { return new LinkedList(); } } }
package edu.buffalo.cse116;
public class PlayingCard {
public enum Suits {
CLUBS, DIAMONDS, HEARTS, SPADES;
@Override
public String toString() {
return name().substring(0, 1);
}
}
public enum Ranks {
TWO("2"), THREE("3"), FOUR("4"), FIVE("5"), SIX("6"), SEVEN("7"), EIGHT("8"), NINE("9"), TEN("T"), JACK("J"),
QUEEN("Q"), KING("K"), ACE("A");
private String display;
private Ranks(String s) {
display = s;
}
@Override
public String toString() {
return display;
}
}
private Suits suit;
private Ranks value;
public PlayingCard(Suits s, Ranks r) {
suit = s;
value = r;
}
public Suits getSuit() {
return suit;
}
public Ranks getValue() {
return value;
}
@Override
public String toString() {
return value.toString() + suit.toString();
}
public static PlayingCard[] generate(int numDecks) {
int length = numDecks * Suits.values().length * Ranks.values().length;
PlayingCard[] retVal = new PlayingCard[length];
int idx = 0;
for (int i = 0; i < numDecks; i++) {
for (Suits s : Suits.values()) {
for (Ranks r : Ranks.values()) {
PlayingCard playa = new PlayingCard(s, r);
retVal[idx] = playa;
idx += 1;
}
}
}
return retVal;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
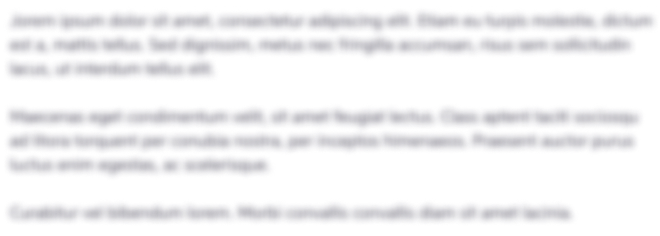
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started