Question
This code is meant to iterate through a csv file (written in code) and compare each location's distance from the user input coordinates. The program
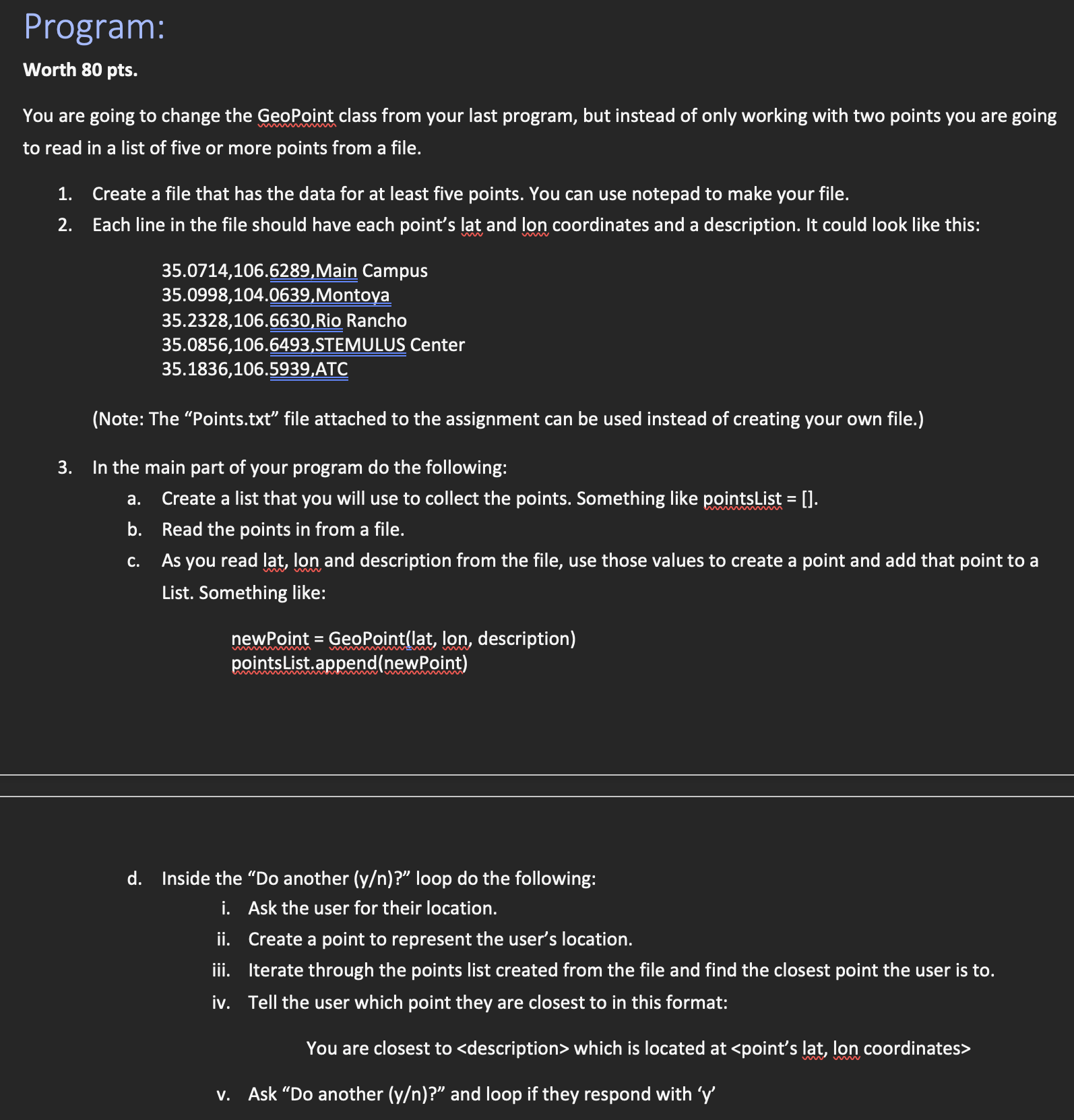
This code is meant to iterate through a csv file (written in code) and compare each location's distance from the user input coordinates. The program will then tell the user which point she is closest to and ask if they want to compare again.
I have attached my code so far.
The CalcDistance function is correct but maybe my indexing is wrong because I am getting a TypeError.
Am I comparing the locations appropriately to the user input?
Please let me know if you have questions. I also uploaded the assignment file for reference.
from math import sin, cos, atan2, sqrt, radians
class GeoPoint:
"""This class will keep track of a point in space using latitude and longitude."""
def __init__(self, lat=0, long=0, pin="TBD"):
"""Initalize the coordinates with parameterized and default constructors."""
self.__lat = lat
self.__long = long
self.__pin = pin
def SetPoint(self, coords):
"""This will be a comparison point in space."""
self.__lat = coords[0]
self.__long = coords[1]
def GetPoint(self):
"""Returns a tuple of the coordinates."""
return (self.__lat, self.__long)
def CalcDistance(self, point):
"""Calculates the distance in kilometers between between two points."""
dlat = radians(point[0] - (self.__lat))
dlong = radians(point[1] - (self.__long))
r = 6371
A = ( sin(dlat/2) **2) + ( cos(radians(self.__lat)) * cos(radians(point[0])) * (sin(dlong/2) **2) )
C = 2 * atan2(sqrt(A), sqrt(1-A))
D = r * C
return D
def SetPin(self, pin):
"""Here the descirption attributes are set."""
self.__pin = pin
def GetPin(self):
"""Method to return object attributes."""
return self.__pin
##__property method utilized to access and set pin values and descriptions__
point = property(GetPoint, SetPoint)
pin = property(GetPin, SetPin)
## Main Program ##
import csv
# this program will write a csv file (comma-separated values)
f = open('geopoint_list.csv', 'w+')
pinWriter = csv.writer(f)
pinWriter.writerow(["Latitude", "Longitude", "Description"])
pinWriter.writerow([39.2474, -114.8886, "Ely, NV, USA"])
pinWriter.writerow([27.8666, -108.215, "Uruachi, CH, MX"])
pinWriter.writerow([35.6870, -105.9378, "Santa Fe, NM, USA"])
pinWriter.writerow([37.7749, -122.4194, "San Francisco, CA, USA"])
pinWriter.writerow([-0.2167, -78.4, "Tumbaco, EC"])
f.close()
# Empty list is created to be populated
pinList = []
with open('geopoint_list.csv','r') as f:
for line in f:
elements = f.readline().split(",")
lat = elements[0]
long = elements[1]
pin = elements[2]
newLoc = GeoPoint(lat, long, pin)
pinList.append(newLoc)
print(pinList)
#Initiate conversation with the user using a while loop
newPin = 'y'
while newPin == 'y':
lat, long = map(float,input("Enter a location's coordinates as latitude, longitude to track it:\n"
+"Example: 34.4839, -114.3225\n").split(","))
#calculate user coordinate input distance to each location in the pinList.
toPinOne = pinList[1].CalcDistance([lat, long])
toPinTwo = pinList[2].CalcDistance([lat, long])
toPinThree = pinList[3].CalcDistance([lat, long])
toPinFour = pinList[4].CalcDistance([lat, long])
toPinFive = pinList[5].CalcDistance([lat, long])
#compare the distance for the user and output results
if toPinOne > toPinTwo:
print(f"You are closest to the coordinates {pinList[2].GetPin()} which is ", tuple(pinList[2].point))
if toPinOne > toPinThree:
print(f"You are closest to the coordinates {pinList[3].GetPin()} which is ", tuple(pinList[3].point))
if toPinOne > toPinFour:
print(f"You are closest to the coordinates {pinList[4].GetPin()} which is ", tuple(pinList[4].point))
if toPinOne > toPinFive:
print(f"You are closest to the coordinates {pinList[5].GetPin()} which is ", tuple(pinList[5].point))
else:
print(f"You are closest to the coordinates {pinList[1].GetPin()} which is ", tuple(pinList[1].point))
#establish if another loop is required or needed
newPin = input("Track another location (y/n)? \n").lower()
if newPin == "n":
print("Thank you, Come again!")
break
Program: Worth 80 pts. You are going to change the GeoPoint class from your last program, but instead of only working with two points you are going to read in a list of five or more points from a file. 1. Create a file that has the data for at least five points. You can use notepad to make your file. 2. Each line in the file should have each point's lat and lon coordinates and a description. It could look like this: 35.0714,106.6289,Main Campus 35.0998,104.0639,Montoya 35.2328,106.6630, Rio Rancho 35.0856,106.6493,STEMULUS Center 35.1836,106.5939,ATC (Note: The "Points.txt file attached to the assignment can be used instead of creating your own file.) 3. In the main part of your program do the following: a. Create a list that you will use to collect the points. Something like pointsList = []. b. Read the points in from a file. C. As you read lat, lon and description from the file, use those values to create a point and add that point to a List. Something like: newPoint = GeoPoint(lat, lon, description) pointsList.append(newPoint) d. Inside the "Do another (y/n)? loop do the following: i. Ask the user for their location. ii. Create a point to represent the user's location. iii. Iterate through the points list created from the file and find the closest point the user is to. iv. Tell the user which point they are closest to in this format: You are closest to which is located at
Step by Step Solution
3.32 Rating (146 Votes )
There are 3 Steps involved in it
Step: 1
1 The code provided is meant to iterate through a csv file and compare each locations distance from the user input coordinates using the GeoPoint clas...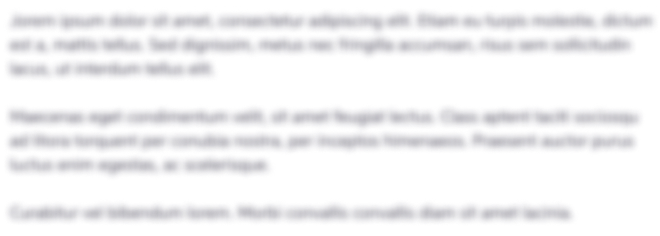
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started